Database
Integration with Applications
Integrating databases with backend applications is a fundamental task for any developer. In this chapter, we will learn how to connect SQL and NoSQL databases with popular backend frameworks and analyze the differences between using ORMs and native queries.
Database Connection to Backend Frameworks
Backend frameworks like Node.js, Django, and Laravel allow developers to connect applications to databases and manage data efficiently. Next, we will see how to connect databases to these frameworks.
Connection in Node.js
In Node.js, we connect databases using specific packages. For example, to connect to a MySQL database, we use the package mysql2:
bash
Then, we configure the connection:
javascript
For MongoDB, we use mongoose as a driver:
bash
javascript
Connection in Django (Python)
Django is a Python framework with built-in support for connecting SQL databases. To set up the connection, we modify the settings.py file:
python
For MongoDB, we use the djongo package:
bash
Then we configure settings.py:
python
Database Drivers (MySQL, MongoDB)
Database drivers allow the connection between an application and the database. The most popular drivers include:
- mysql2 for MySQL in Node.js
- pg for PostgreSQL
- mongoose for MongoDB
- djongo for MongoDB in Django
It's important to choose the correct driver to ensure that connections are secure and efficient.
ORMs vs Native Queries
When developing applications, we can choose to use an ORM (Object-Relational Mapping) or execute native queries directly to the database. Both options have their advantages and disadvantages.
ORMs
An ORM allows developers to work with databases using objects in the programming language. Some popular ORMs are:
- Sequelize for Node.js and MySQL
- Mongoose for MongoDB
- Django ORM for Django
Advantages of ORMs
- Database abstraction: No need to write SQL queries directly.
- Error reduction: ORMs help prevent common errors in SQL queries.
- Portability: Allows changing from one database to another with fewer code changes.
Disadvantages of ORMs
- Performance: Queries generated by ORMs may be slower than native queries.
- Complexity: ORMs can be harder to debug when something goes wrong.
Native Queries
Native queries allow developers to interact directly with the database using SQL (or NoSQL queries in the case of MongoDB).
Advantages of Native Queries
- Greater control: Developers have total control over the query.
- Better performance: Native queries tend to be faster because they are optimized for the specific database.
Disadvantages of Native Queries
- Complexity: Developers must write and manage queries manually.
- Lack of portability: Migrating between databases may require rewriting queries.
Performance Comparison between ORMs and Native Queries
In applications with many read and write operations, there may be a significant performance difference between ORMs and native queries. Generally, native queries are faster, but ORMs provide more ease in managing data in complex applications.
Summary
In this chapter, we learned how to connect SQL and NoSQL databases to different backend frameworks, explored the most common drivers, and discussed the advantages and disadvantages of using ORMs compared to native queries. In the next chapter, we will cover how to handle database migration and scalability in large-scale systems.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
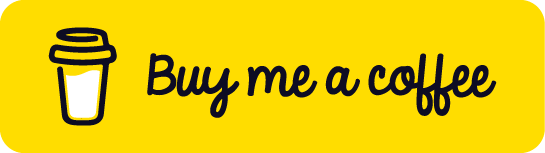
Chat with Chuck
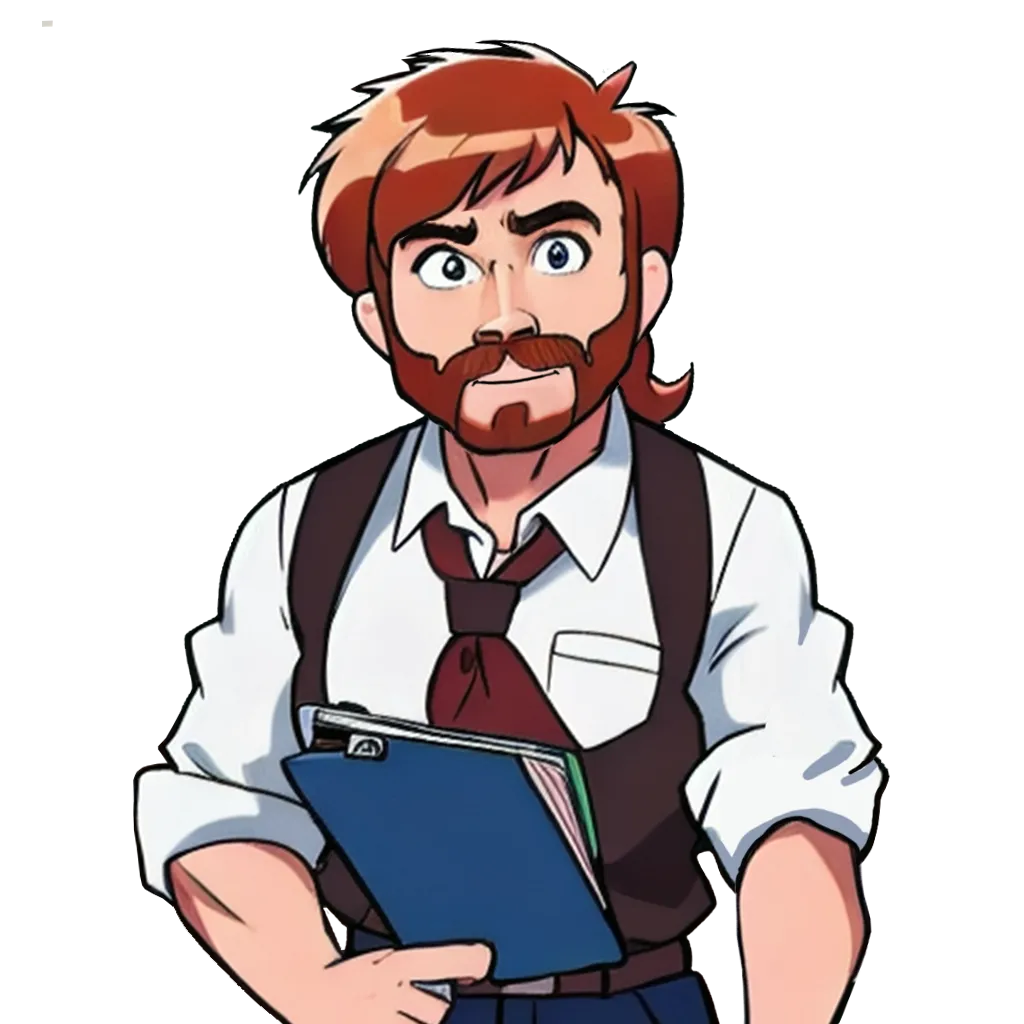
- Introduction to Databases
- Introduction to SQL and MySQL
- Relational Database Design
- CREATE Operations in SQL
- INSERT Operations in SQL
- SELECT Operations in SQL
- UPDATE Operations in SQL
- DELETE Operations in SQL
- Seguridad y Gestión de Usuarios en SQL
- Introduction to NoSQL and MongoDB
- Data Modeling in NoSQL
- CREATE Operations in MongoDB
- READ Operations in MongoDB
- Update Operations in MongoDB
- DELETE Operations in MongoDB
- Security and Management in MongoDB
- Database Optimization
- Integration with Applications
- Migración y Escalabilidad de Bases de Datos
- Conclusion and Additional Resources