Database
CREATE Operations in MongoDB
In this chapter, we will learn how to perform CREATE operations in MongoDB, which involves inserting new documents into collections. Unlike SQL, where we use INSERT to add data, in MongoDB we work directly with documents in JSON format and use the insert command or its variants to add these documents to collections.
Inserting a Document into a Collection
To insert a document in MongoDB, we use the insertOne() method to add a single document to a collection. Below is an example of how to insert a new user into the users collection:
javascript
Inserting Multiple Documents
If we want to insert multiple documents at once, we can use the insertMany() method. This is useful when we need to add multiple records simultaneously.
javascript
Auto-generated and Optional Fields
In MongoDB, it is not necessary to define all fields of a document before inserting it. Documents can be as flexible as needed, and MongoDB will automatically generate the _id field if we do not specify it.
javascript
MongoDB also allows certain fields to be absent in different documents. Unlike relational databases, it is not necessary for all documents in a collection to have exactly the same fields.
Inserting Nested Documents
MongoDB allows the creation of nested documents, meaning we can include objects within a document. This is useful when working with complex data that has a hierarchical structure.
javascript
Inserting Arrays
In addition to nested objects, MongoDB also allows storing arrays in documents. This is useful when we want to associate multiple values with a single field.
javascript
Common Errors When Inserting Documents
Duplicates of _id
One of the most common errors when inserting documents is trying to add a document with an _id that already exists in the collection. MongoDB does not allow duplicates in the _id field as this serves as the primary key.
javascript
Summary
In this chapter, we have learned how to insert documents in MongoDB using the methods insertOne() and insertMany(). We also explored how to work with nested documents, arrays, and how to handle optional fields in MongoDB. In the next chapter, we will learn how to read and query documents in a MongoDB database using the find() method.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
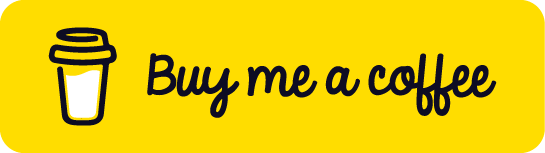
Chat with Chuck
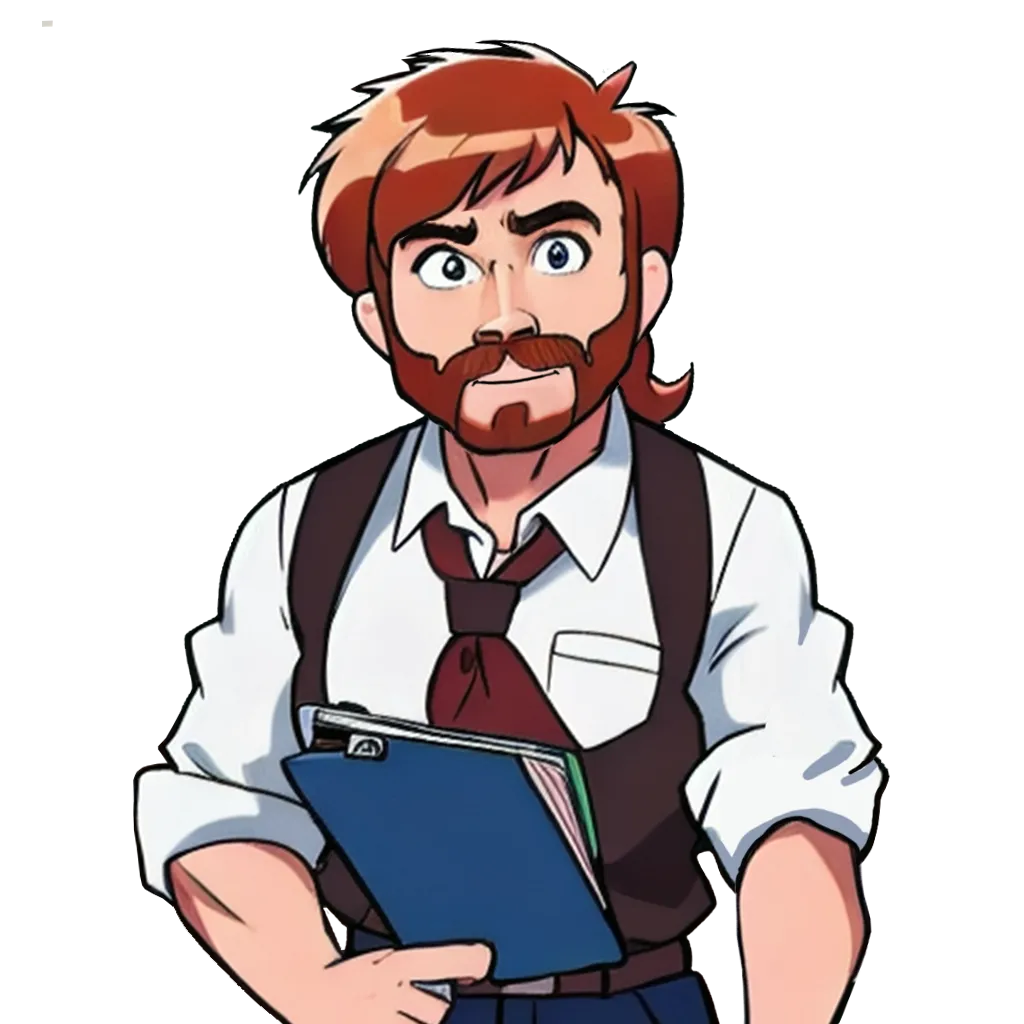
- Introduction to Databases
- Introduction to SQL and MySQL
- Relational Database Design
- CREATE Operations in SQL
- INSERT Operations in SQL
- SELECT Operations in SQL
- UPDATE Operations in SQL
- DELETE Operations in SQL
- Seguridad y Gestión de Usuarios en SQL
- Introduction to NoSQL and MongoDB
- Data Modeling in NoSQL
- CREATE Operations in MongoDB
- READ Operations in MongoDB
- Update Operations in MongoDB
- DELETE Operations in MongoDB
- Security and Management in MongoDB
- Database Optimization
- Integration with Applications
- Migración y Escalabilidad de Bases de Datos
- Conclusion and Additional Resources