Database
UPDATE Operations in SQL
The UPDATE command in SQL allows us to modify existing data in a table. This operation is crucial when we need to fix errors or update outdated information. In this chapter, we will learn how to use UPDATE safely and efficiently.
Updating Records with UPDATE
The most common use of UPDATE is to modify one or more fields of a specific record in a table. Here is a basic example to update a user's email in the users table.
sql
Use WHERE to Avoid Mass Updates
It's important to always use the WHERE clause in our UPDATE queries to avoid accidentally updating all records in a table. If we omit WHERE, all records will be modified.
sql
Updating Multiple Columns
We can update more than one column at a time by specifying multiple assignments in the SET clause. Here we have an example where we update both the name and the email of a user:
sql
Updating Multiple Records
It's possible to update multiple records in a single operation if the WHERE clause selects more than one record. For example, to change the domain of all emails ending in 'old_domain.com' to 'new_domain.com', we can do the following:
sql
Updating with Complex Conditions
We can use logical operators like AND and OR in the WHERE clause to define more complex conditions in our updates. For example, to update all users who were created before 2023 and have an email ending in 'example.com', we can do the following:
sql
Using Subqueries in UPDATE
In some cases, it's useful to update data in a table based on values from another table. This can be done using subqueries in the SET clause. For example, suppose we want to update the total_orders field in the users table based on the number of orders they have in the orders table:
sql
Summary
In this chapter, we learned to use the UPDATE command to modify data in an SQL database. We saw how to update individual records, update multiple columns and records, use complex conditions, and leverage subqueries in our updates. In the next chapter, we will see how to delete data from our tables using the DELETE command.
- Introduction to Databases
- Introduction to SQL and MySQL
- Relational Database Design
- CREATE Operations in SQL
- INSERT Operations in SQL
- SELECT Operations in SQL
- UPDATE Operations in SQL
- DELETE Operations in SQL
- Seguridad y Gestión de Usuarios en SQL
- Introduction to NoSQL and MongoDB
- Data Modeling in NoSQL
- CREATE Operations in MongoDB
- READ Operations in MongoDB
- Update Operations in MongoDB
- DELETE Operations in MongoDB
- Security and Management in MongoDB
- Database Optimization
- Integration with Applications
- Migración y Escalabilidad de Bases de Datos
- Conclusion and Additional Resources
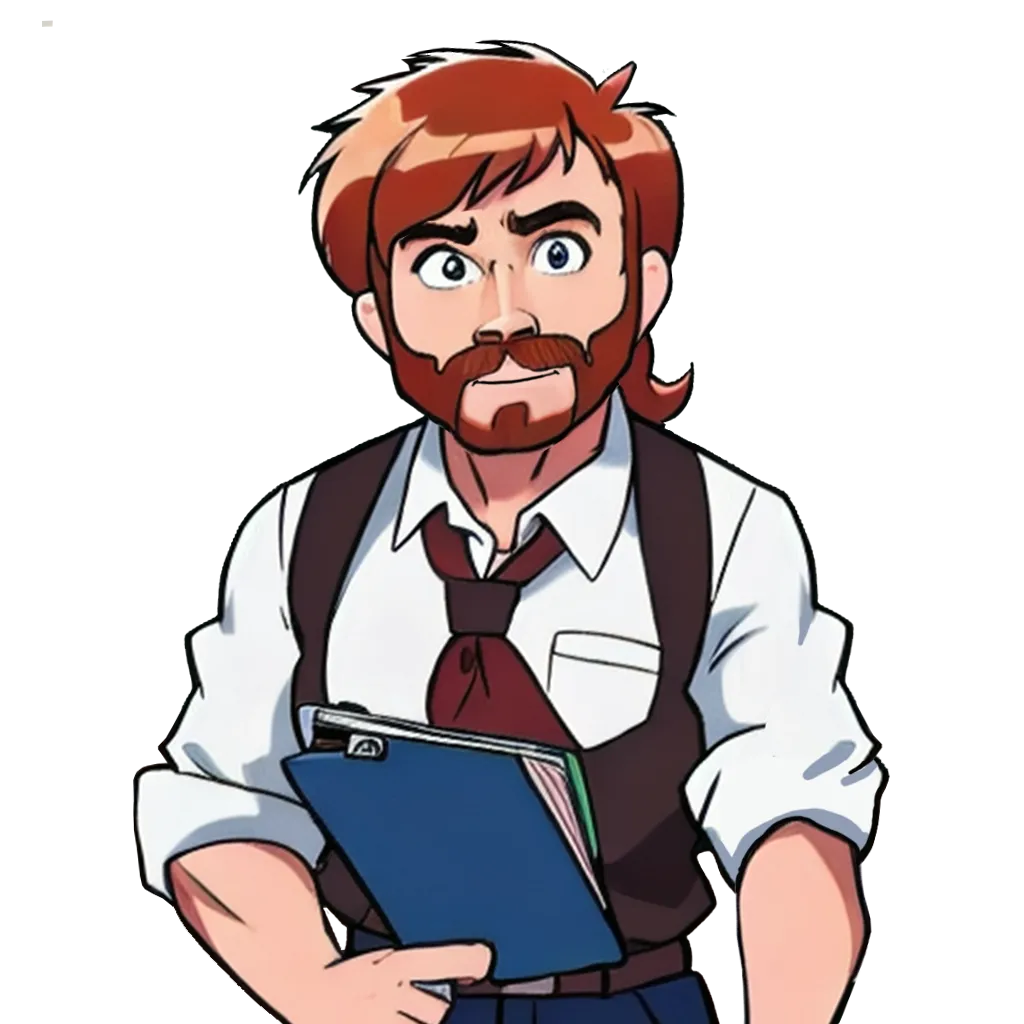