Database
INSERT Operations in SQL
After creating our tables in an SQL database, the next step is inserting data. The INSERT operation allows us to add data rows to a table. In this chapter, we will learn to insert data into a database using the INSERT INTO command, as well as some best practices for handling this process.
Inserting Data into a Table
The most basic operation we perform with a table is inserting new data. The INSERT INTO command is how new rows are added to an existing table. Below is an example of how to insert a record into a table called users
.
sql
Inserting Multiple Rows
Often, we need to insert multiple rows of data at once. Instead of making multiple individual insertions, we can use a single INSERT INTO statement to insert multiple rows at once.
sql
Inserting Data into All Columns
If we wish to insert data into all columns of a table, we can omit the column names in the INSERT INTO statement, as long as we provide the values in the same order in which the columns are defined.
sql
Conditional Inserts with ON DUPLICATE KEY UPDATE
In some cases, we may want to update an existing record if there is one with a duplicate key. For this, we can use the ON DUPLICATE KEY UPDATE clause in combination with data insertion.
sql
Best Practices for Data Insertion
-
Use transactions when inserting large amounts of data. This ensures the operation is atomic and data does not remain incomplete in the event of a failure.
-
Validate data before inserting to ensure it meets database constraints (like unique primary keys and non-null fields).
-
Avoid mass insertions without an index in large tables as this can cause performance issues. It's recommended to create indexes before inserting large volumes of data.
sql
Specifying Columns
In the previous example, we specified the columns name and email. If we do not specify all columns, it is necessary to omit those that have a default value or can be null. For example, we did not specify the id column because in the users table, this field is an auto-increment primary key.
sql
Inserting Multiple Rows
The INSERT command also allows inserting multiple rows at once, which is very useful when a table needs to be filled with several records.
sql
Inserting Data with AUTO_INCREMENT
When a table has a column defined as AUTO_INCREMENT, as in our example with the id column in the users table, it is not necessary to specify the value for that column when inserting a new record. MySQL will automatically assign the next available value for the primary key.
sql
Inserting Data with Null Values
Sometimes it is necessary to insert a record that does not have values for all columns. In those cases, we can insert a NULL value in the columns that allow it.
sql
Inserting Data with Subqueries
Instead of providing the values manually, it is also possible to insert data into a table using the results of a query. This technique is called insert with subquery.
sql
Summary
In this chapter, we explored how to insert data into SQL tables using the INSERT INTO command, including inserting multiple rows and using the ON DUPLICATE KEY UPDATE clause. In the next chapter, we will learn to retrieve data from a database using the SELECT command, one of the most powerful in SQL.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
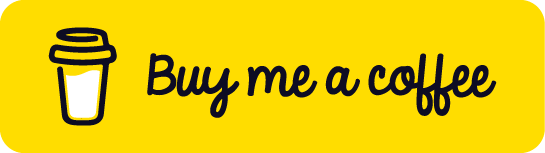
Chat with Chuck
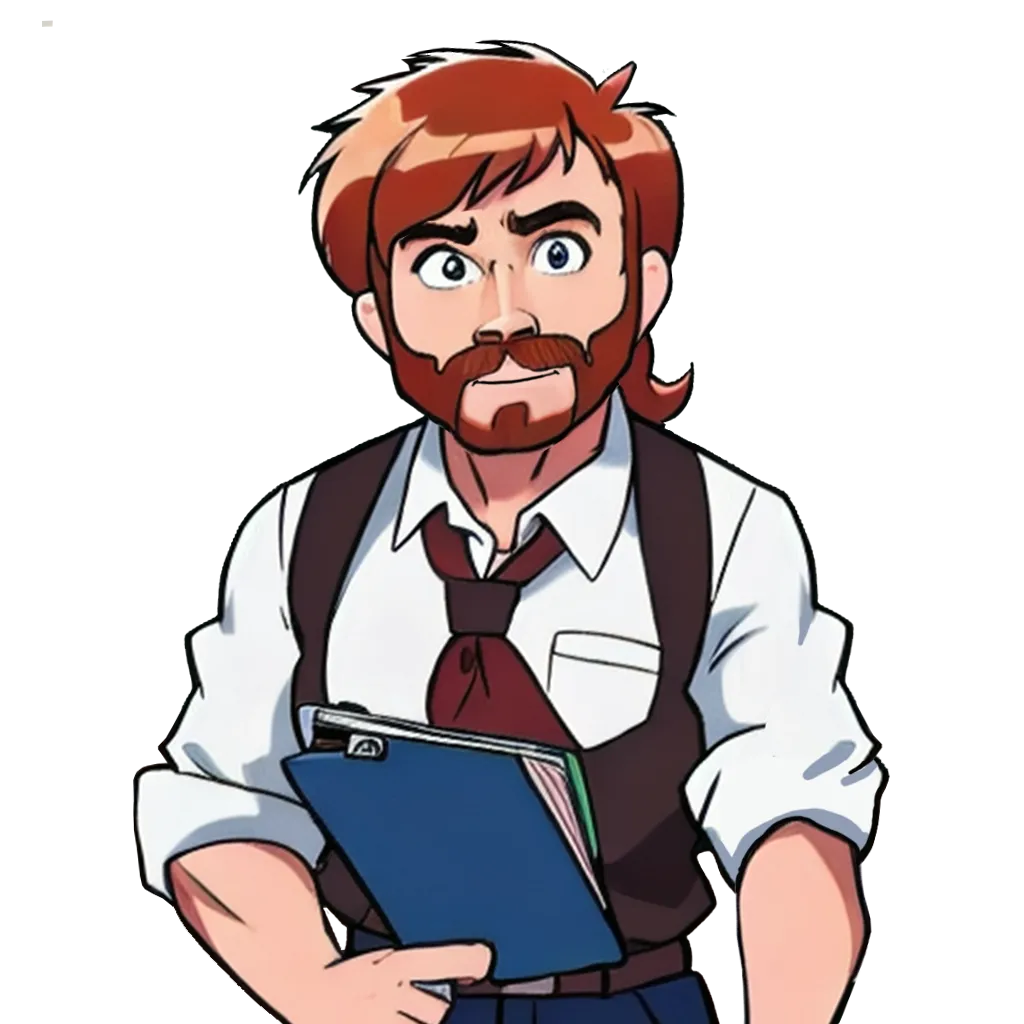
- Introduction to Databases
- Introduction to SQL and MySQL
- Relational Database Design
- CREATE Operations in SQL
- INSERT Operations in SQL
- SELECT Operations in SQL
- UPDATE Operations in SQL
- DELETE Operations in SQL
- Seguridad y Gestión de Usuarios en SQL
- Introduction to NoSQL and MongoDB
- Data Modeling in NoSQL
- CREATE Operations in MongoDB
- READ Operations in MongoDB
- Update Operations in MongoDB
- DELETE Operations in MongoDB
- Security and Management in MongoDB
- Database Optimization
- Integration with Applications
- Migración y Escalabilidad de Bases de Datos
- Conclusion and Additional Resources