Database
READ Operations in MongoDB
In this chapter, we will learn how to perform READ operations in MongoDB, which involves querying documents within collections. The main command to perform reads in MongoDB is find(), which allows us to search for and retrieve documents that match certain criteria.
Retrieving All Documents
The simplest use of find() is to retrieve all documents in a collection. If we want to get all users from the users collection, we use the following command:
javascript
MongoDB returns documents in JSON format, and the find() command without parameters returns all records in the collection. This may not be efficient when the collection contains a large number of documents, so it's often better to use filters.
Filtering Results with find()
To filter results and obtain only documents that meet certain criteria, we can pass a query object to the find() method. Here is an example where we search for users who are over 25 years old:
javascript
Comparison Operators
MongoDB provides several operators for comparing values within documents:
- $gt: Greater than
- $lt: Less than
- $gte: Greater than or equal to
- $lte: Less than or equal to
- $eq: Equal to
- $ne: Not equal to
Below is an example of how to combine comparison operators:
javascript
Field Projection
In some queries, we only need to obtain certain fields from the documents. MongoDB allows us to specify which fields we want to include or exclude in the results using a projection. Here is an example where we want to get only the names and emails of users:
javascript
Including and Excluding Fields
- Include a field: We specify the field with a value of 1 in the projection object.
- Exclude a field: We specify the field with a value of 0.
It is not possible to mix field inclusion and exclusion in the same query, except for the _id field.
Sorting Results
MongoDB allows us to sort query results using the sort() method. Here is an example where we sort users by age in descending order:
javascript
- 1: Ascending order.
- -1: Descending order.
Limiting and Paginating Results
When working with large volumes of data, it is useful to limit the number of results we get. MongoDB allows you to limit results with limit() and skip a specific number of documents with skip(), which is useful for implementing pagination.
Limiting Results
javascript
Pagination
To paginate results, we can use a combination of limit() and skip(). Here is an example of how to get the second page of results with five documents per page:
javascript
Logical Operators in Queries
We can combine multiple conditions in a query using logical operators like $and, $or, and $not. For example, to get users who are over 25 years old or live in New York, we can use the following command:
javascript
Example with $and
If we wanted to combine conditions with $and, the command would be:
javascript
Summary
In this chapter, we have learned how to perform read operations in MongoDB using the find() method. We saw how to filter results, project fields, sort, limit, and paginate results, as well as how to use logical operators in our queries. In the next chapter, we will explore how to update documents in MongoDB using the update() method.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
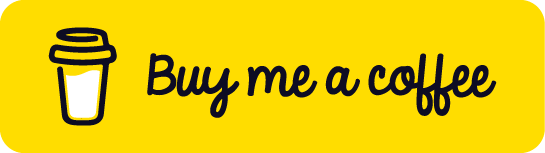
Chat with Chuck
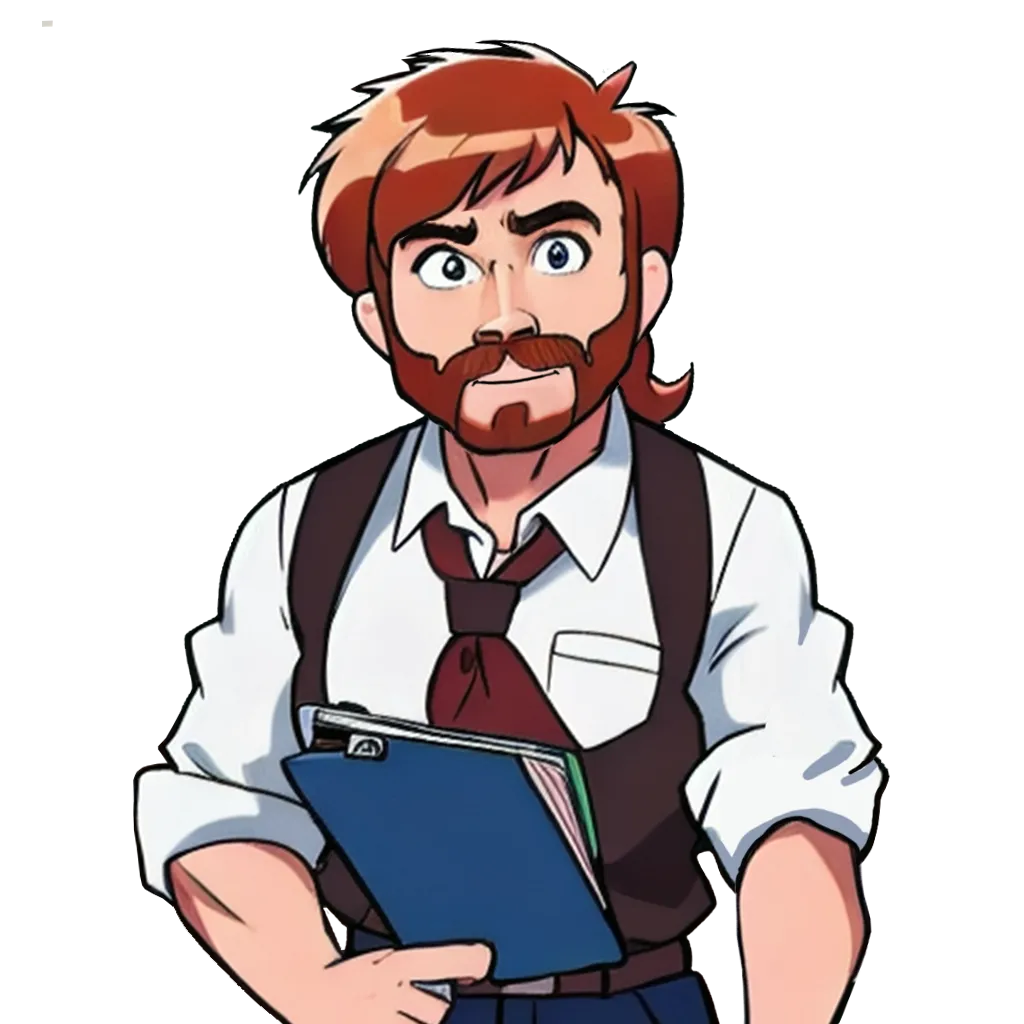
- Introduction to Databases
- Introduction to SQL and MySQL
- Relational Database Design
- CREATE Operations in SQL
- INSERT Operations in SQL
- SELECT Operations in SQL
- UPDATE Operations in SQL
- DELETE Operations in SQL
- Seguridad y Gestión de Usuarios en SQL
- Introduction to NoSQL and MongoDB
- Data Modeling in NoSQL
- CREATE Operations in MongoDB
- READ Operations in MongoDB
- Update Operations in MongoDB
- DELETE Operations in MongoDB
- Security and Management in MongoDB
- Database Optimization
- Integration with Applications
- Migración y Escalabilidad de Bases de Datos
- Conclusion and Additional Resources