Database
DELETE Operations in MongoDB
In this chapter, we will learn how to perform DELETE operations in MongoDB, which allow us to remove documents from a collection. MongoDB offers several methods to delete documents, either individually or in bulk, depending on the search criteria we specify.
Deleting a Document with deleteOne()
The deleteOne() method deletes the first document that matches the search criteria. If we find multiple documents that meet the criteria, only the first one will be deleted.
Example of deleteOne()
javascript
This command will only delete the first document that matches the search criteria, even if there are several that meet the same criteria.
Deleting Multiple Documents with deleteMany()
If we need to delete multiple documents that match a criterion, we can use deleteMany(). This method deletes all documents that meet the criteria.
Example of deleteMany()
javascript
Conditional Deletion
As with other operations in MongoDB, we can perform deletions based on complex conditions using logical operators like $and, $or, and $not. For example, if we wanted to delete users who are over 30 years old or have not verified their email, we could use the following command:
javascript
Example with $and
We can combine conditions with $and to delete documents that meet several conditions simultaneously:
javascript
Caution When Using deleteMany()
It is important to be careful when using deleteMany() to ensure that we are not deleting more documents than we really want. If we omit the search clause, MongoDB will delete all documents in the collection.
javascript
Alternative: Soft Delete
Instead of permanently deleting documents, some applications prefer to implement a technique called soft delete, which involves marking a document as deleted without actually removing it. This allows the document to remain in the database but be excluded from queries.
Example of Soft Delete
javascript
The advantage of this technique is that we can "restore" the deleted documents simply by removing or updating the deleted_at field.
Summary
In this chapter, we have learned how to delete documents in MongoDB using the deleteOne() and deleteMany() methods. We also explored how to use logical operators in deletions and how to implement the soft delete approach to retain data without permanently deleting it. In the next chapter, we will learn how to manage security and permissions in MongoDB.
- Introduction to Databases
- Introduction to SQL and MySQL
- Relational Database Design
- CREATE Operations in SQL
- INSERT Operations in SQL
- SELECT Operations in SQL
- UPDATE Operations in SQL
- DELETE Operations in SQL
- Seguridad y Gestión de Usuarios en SQL
- Introduction to NoSQL and MongoDB
- Data Modeling in NoSQL
- CREATE Operations in MongoDB
- READ Operations in MongoDB
- Update Operations in MongoDB
- DELETE Operations in MongoDB
- Security and Management in MongoDB
- Database Optimization
- Integration with Applications
- Migración y Escalabilidad de Bases de Datos
- Conclusion and Additional Resources
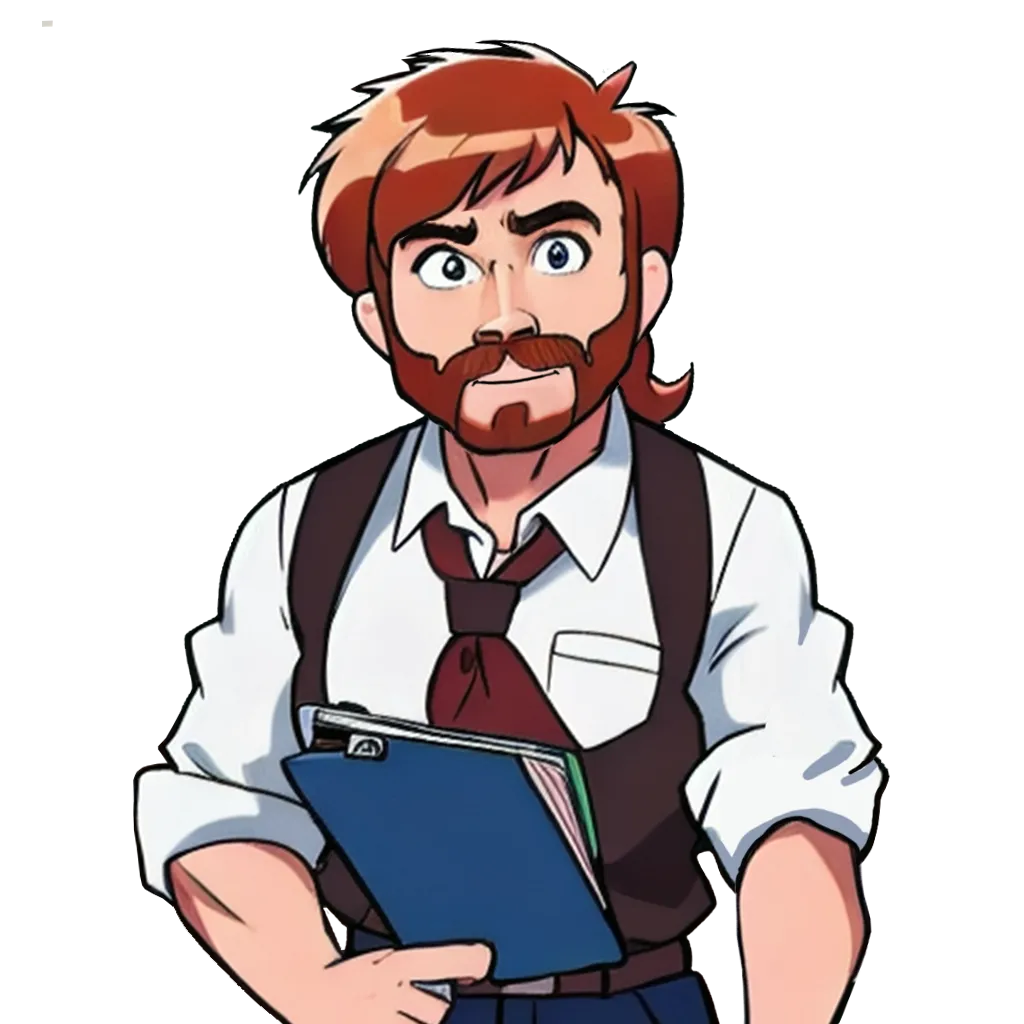