Database
Update Operations in MongoDB
In this chapter, we will learn how to perform UPDATE operations in MongoDB, which allow us to modify existing documents in a collection. MongoDB offers several ways to update documents, from updating individual fields to modifying multiple documents at once.
Updating a Document with updateOne()
To update a single document in MongoDB, we use the updateOne() method. This method allows us to update the first document that matches the search criteria.
Example of updateOne()
javascript
The $set Operator
The $set operator allows us to update one or more fields in a document without modifying others. If a field does not exist, MongoDB will create it.
javascript
Updating Multiple Documents with updateMany()
If we need to update multiple documents that match a criterion, we can use updateMany(). This method allows us to modify all documents that meet the specified criteria.
Example of updateMany()
javascript
[Placeholder for image: This image shows how updateMany modifies multiple documents in a MongoDB collection.]
Updating Arrays in MongoDB
MongoDB offers a range of specific operators for working with arrays within documents. For example, we can use the $push operator to add a new element to an array:
javascript
The $pull Operator
The $pull operator allows us to remove a specific element from an array:
javascript
Conditional Updates
Sometimes it's useful to perform updates based on complex conditions. MongoDB allows us to combine logical operators in our update queries. Here's an example of how to combine $and operators to update a document that meets multiple conditions:
javascript
Incrementing Numeric Values with $inc
MongoDB allows incrementing (or decrementing) numeric values using the $inc operator. This is useful when we want to modify a numeric value without directly replacing it.
javascript
Atomic Updates
Update operations in MongoDB are atomic at the document level, meaning if a document is modified, the update is performed safely and consistently. This is important to ensure data consistency during concurrent updates.
Summary
In this chapter, we have learned how to update documents in MongoDB using methods like updateOne() and updateMany(). We also explored how to work with arrays, perform conditional updates, and use operators like $set, $push, $pull, and $inc. In the next chapter, we will learn how to delete documents from a collection using the delete() method.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
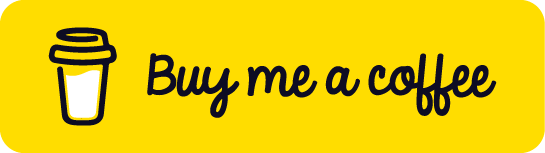
Chat with Chuck
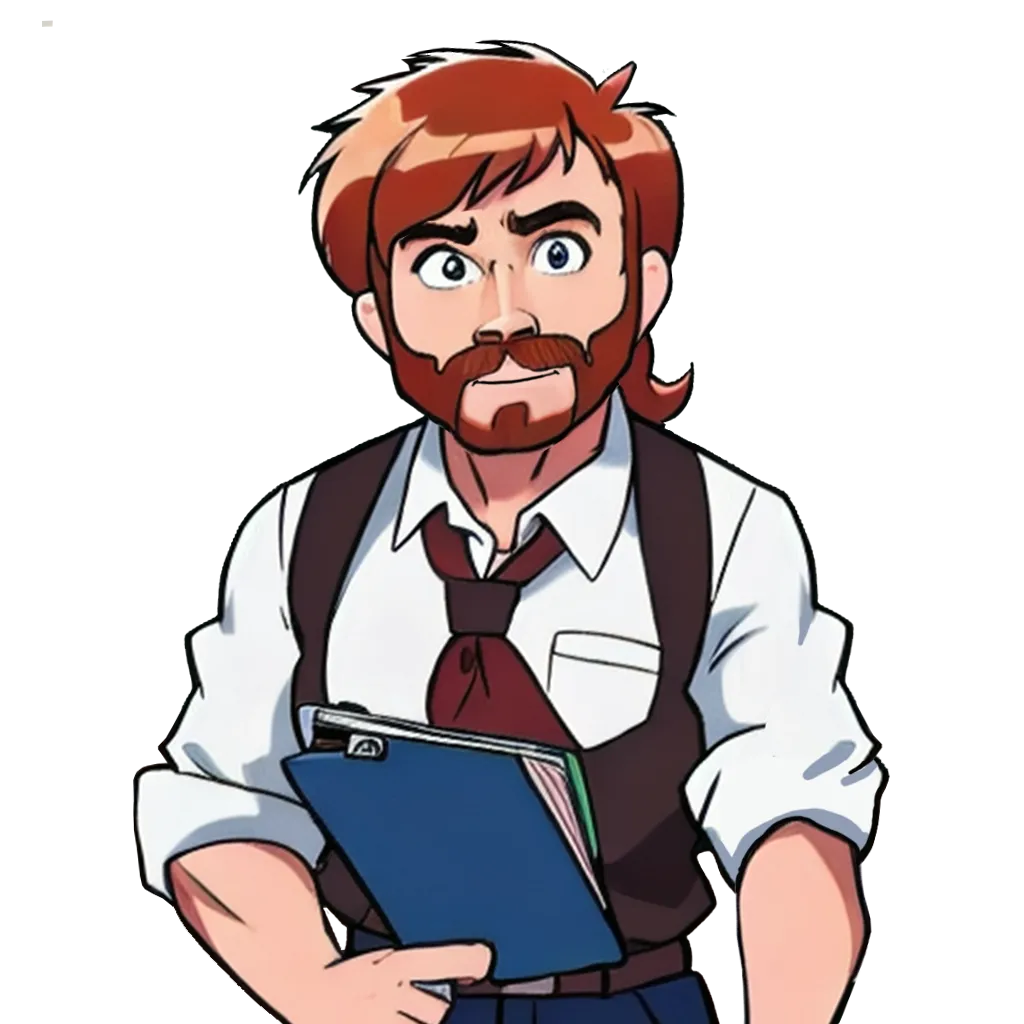
- Introduction to Databases
- Introduction to SQL and MySQL
- Relational Database Design
- CREATE Operations in SQL
- INSERT Operations in SQL
- SELECT Operations in SQL
- UPDATE Operations in SQL
- DELETE Operations in SQL
- Seguridad y Gestión de Usuarios en SQL
- Introduction to NoSQL and MongoDB
- Data Modeling in NoSQL
- CREATE Operations in MongoDB
- READ Operations in MongoDB
- Update Operations in MongoDB
- DELETE Operations in MongoDB
- Security and Management in MongoDB
- Database Optimization
- Integration with Applications
- Migración y Escalabilidad de Bases de Datos
- Conclusion and Additional Resources