Database
Data Modeling in NoSQL
In this chapter, we will explore the data modeling process in NoSQL databases, focusing on MongoDB. Unlike relational databases, where the schema is rigidly defined through tables and relationships, NoSQL databases allow for greater flexibility in modeling data, giving us the ability to adapt to unstructured or frequently changing data.
Collections and Documents
In MongoDB, data is stored in collections, which are equivalent to tables in relational databases. Each collection contains multiple documents, which are flexible data structures in JSON format.
Document Example
A document in MongoDB is similar to a JSON object. Here's an example of a document that could be in a collection called users:
json
Flexible Structure
One of the greatest advantages of MongoDB is its flexible structure. Unlike SQL, where every row in a table must follow the same schema, in MongoDB each document can have a different set of fields. For example, we can add a new document to the users collection with additional fields or fewer fields than the previous one:
json
Relationships in NoSQL
Although MongoDB does not use relationships in the traditional sense of relational databases, we can establish relationships between documents using references or embeddings.
Document Embedding
We can embed documents within other documents when data is closely related and we want to avoid performing multiple queries. In the previous example, the orders are embedded in the users document, making it easy to query all of a user's orders in a single operation.
json
Document References
In some cases, data may be more separated and of large size, so it is preferable to use references between documents instead of embeddings. For example, we can have a users collection and an orders collection, where orders documents reference users through the user_id field:
json
Schema Design for NoSQL
Schema design in NoSQL databases is heavily dependent on application needs. Some common design patterns include:
- Embeddings: Used when data is closely related and frequently queried together.
- References: Used when data is large or needed independently in different parts of the application.
- Arrays: MongoDB allows storing arrays within documents, which is useful for lists like tags, shopping cart items, or orders.
Blog Application Modeling Example
If we are designing a blog application, we might have two collections: one for authors and another for articles. Articles documents could have a reference to the authors:
json
Best Practices in NoSQL Data Modeling
Some best practices to follow when modeling data in MongoDB include:
- Optimize for queries: Design the schema so that the most common queries are efficient.
- Avoid extreme normalization: Unlike SQL, in NoSQL it is not necessary to excessively normalize data. Embeddings are preferable when data is closely related.
- Consider document size: MongoDB has a 16 MB limit per document, so it's important to consider this limit when deciding on embeddings.
Summary
In this chapter, we have learned how to model data in MongoDB using collections and documents. We also explored different modeling techniques, such as embeddings and references, and reviewed best practices to optimize schema design. In the next chapter, we will delve into data creation operations in MongoDB and how to insert documents into our collections.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
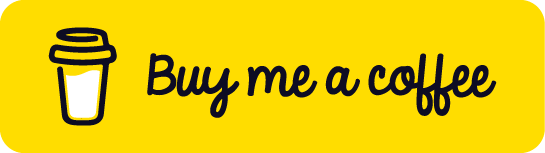
Chat with Chuck
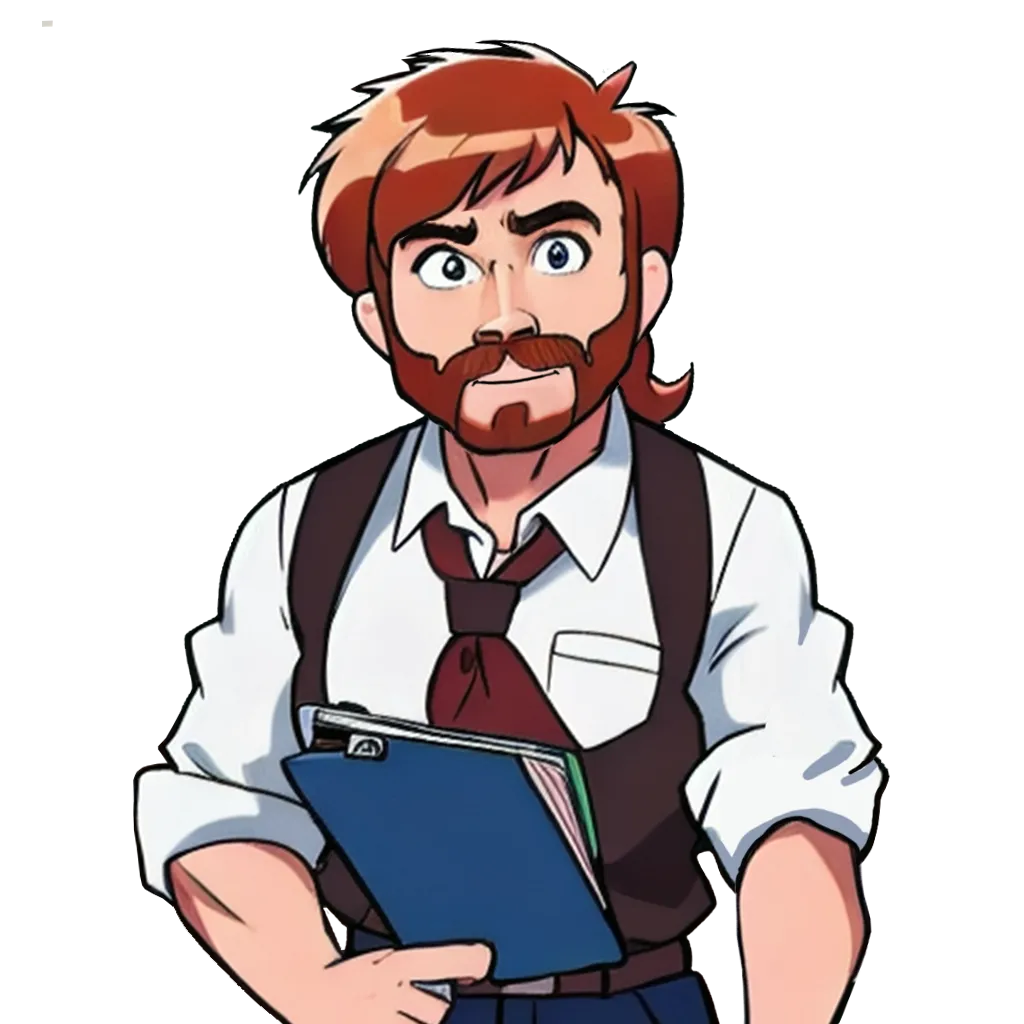
- Introduction to Databases
- Introduction to SQL and MySQL
- Relational Database Design
- CREATE Operations in SQL
- INSERT Operations in SQL
- SELECT Operations in SQL
- UPDATE Operations in SQL
- DELETE Operations in SQL
- Seguridad y Gestión de Usuarios en SQL
- Introduction to NoSQL and MongoDB
- Data Modeling in NoSQL
- CREATE Operations in MongoDB
- READ Operations in MongoDB
- Update Operations in MongoDB
- DELETE Operations in MongoDB
- Security and Management in MongoDB
- Database Optimization
- Integration with Applications
- Migración y Escalabilidad de Bases de Datos
- Conclusion and Additional Resources