Database
CREATE Operations in SQL
In this chapter, we will learn how to perform CREATE operations in SQL databases, focusing on database creation, table creation, and schema definition. The CREATE operation is fundamental in the database design process, as it allows us to establish the structure we will use to store information.
Creating a Database
Before we can store data, we need to create a database. Below is how to create a database in MySQL:
sql
We can verify that the database was successfully created by executing the following command to list all databases in MySQL:
sql
Creating Tables
Once we have a database, the next step is to create tables. Tables are where data is actually stored. Each table must have a unique name within the database and must be composed of columns that define data types.
Creating a Table in MySQL
sql
Primary Keys and Uniqueness
It is important for each table to have a column that acts as a primary key. The primary key ensures that each row in the table is unique and can be used to uniquely identify each record. In the previous example, the id column is the primary key.
Additionally, we can set uniqueness constraints on other columns, as we have done with the email column in the previous example.
Defining Data Types
When creating a table, it is also crucial to correctly define the data types for each column. Some of the most common data types in MySQL include:
- INT: Integer.
- VARCHAR(n): Text string with a limit of n characters.
- DECIMAL(m, d): Decimal number with m digits, of which d are decimals.
- DATE: Date (without time).
- TIMESTAMP: Date and time.
Below is an example of how to create a table with different data types:
sql
Creating Tables with Relationships
In many cases, tables will be related to each other. For this, we use foreign keys, which establish a relationship between rows of one table and another.
Example of Creating Related Tables
sql
Summary
In this chapter, we have learned to use the CREATE command to create databases and tables in SQL. We have also seen how to define columns, data types, and establish relationships between tables. These are the first steps to efficiently structure our databases.
In the next chapter, we will explore how to insert data into the tables we have created using the INSERT operation in SQL.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
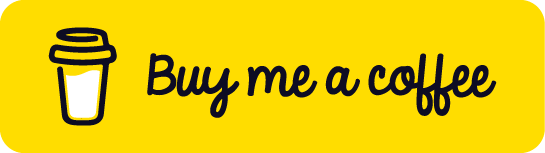
Chat with Chuck
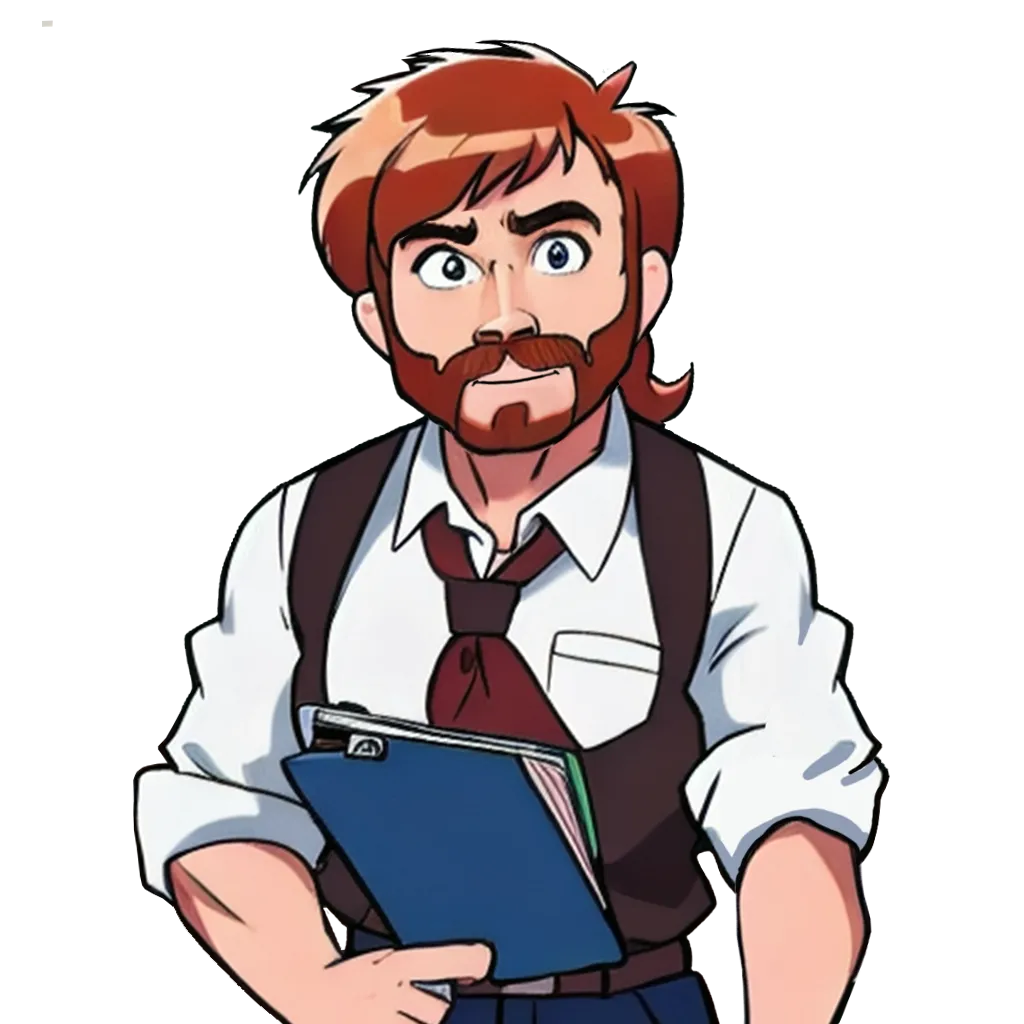
- Introduction to Databases
- Introduction to SQL and MySQL
- Relational Database Design
- CREATE Operations in SQL
- INSERT Operations in SQL
- SELECT Operations in SQL
- UPDATE Operations in SQL
- DELETE Operations in SQL
- Seguridad y Gestión de Usuarios en SQL
- Introduction to NoSQL and MongoDB
- Data Modeling in NoSQL
- CREATE Operations in MongoDB
- READ Operations in MongoDB
- Update Operations in MongoDB
- DELETE Operations in MongoDB
- Security and Management in MongoDB
- Database Optimization
- Integration with Applications
- Migración y Escalabilidad de Bases de Datos
- Conclusion and Additional Resources