HTML5 Canvas
Building Game Logic
To make our game interesting and challenging, we need to build game logic that includes scoring systems, levels, and win or lose conditions. Additionally, modularizing our code will allow us to reuse functions and make development more efficient. In this chapter, we will see how to integrate these elements into our game using the canvas.
Configuring Score, Levels, and Win/Lose Conditions
Score is an essential part of many games, as it gives the player a goal and an incentive to improve. Next, we'll create a basic scoring system and define conditions for the player to win or lose.
Scoring System
First, let's set up a score
variable to store the player's score and a function to increase this score:
javascript
This basic code increments the player's score. We can call increaseScore
each time the player completes a task or removes an obstacle.
Win and Lose Conditions
Let's define conditions for the player to win or lose the game. For example, we can set the player to win if they reach a certain score and lose if they collide with an obstacle.
javascript
In this example, checkWinCondition
checks if the score reaches the necessary score to win, while checkLossCondition
checks collisions to determine a loss.
Code Modularization for Reuse
To make game code easier to maintain and expand, it is useful to divide it into independent modules or functions. This allows functions to be reused in different parts of the game.
Creating Reusable Functions
Next, we modularize game code into functions that perform specific tasks, such as updating the score, checking game conditions, or resetting the game.
javascript
These modular functions help keep the code clean and avoid repeating logic in various parts of the game.
Incorporating Times and Game Progression
To make the game more challenging, we can add timers or make the difficulty increase progressively. This adds a sense of progress and challenge to the game.
Game Timer
Let's add a timer that limits the time the player has to complete the game.
javascript
This timer gives the player a limited amount of time to win the game, increasing the difficulty.
Difficulty Progression
We can increase the game's difficulty by increasing the speed of obstacles or reducing the available time as the player advances through levels.
javascript
This code allows the game to become more challenging as the player progresses, adding a sense of progression.
Summary
In this chapter, we learned how to implement basic game logic, including scoring systems, win and lose conditions, and how to modularize code to make development more efficient. We also saw how to incorporate a timer and difficulty progression to make the game more interesting and challenging.
- Introducción a HTML Canvas
- Handling Colors, Strokes, and Fills
- Adding Text to the Canvas
- Capturing User Input
- Handling Images and Sprites in Canvas
- Object Animation in Canvas
- Detección de Colisiones y Lógica de Juego
- Building Game Logic
- Adding Sound Effects and Music
- Enhancing User Interactivity and Visual Experience
- Adding a HUD and Scoreboard
- Optimización del Rendimiento y Compatibilidad del Juego
- Completion and Game Testing
- Game Publishing
- Complete Project – Building the Full Game
- Next Steps
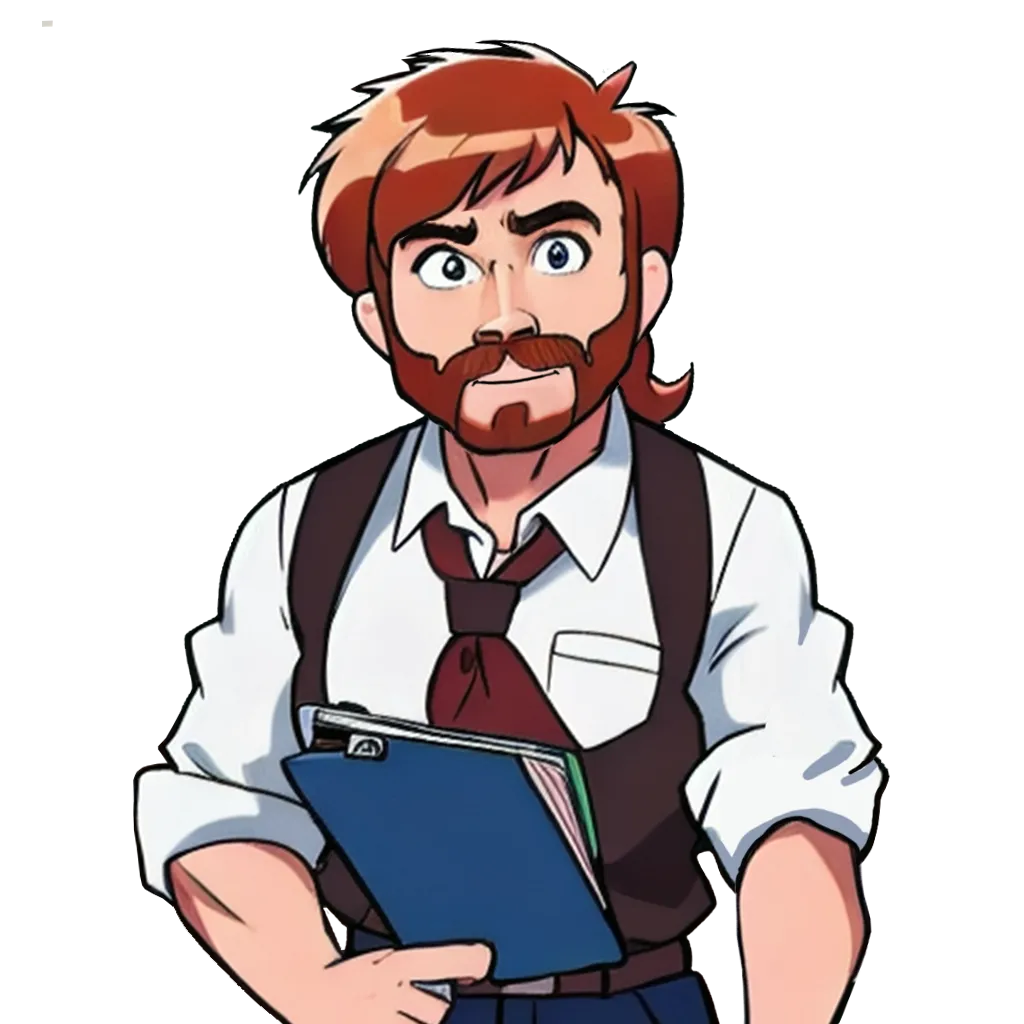