HTML5 Canvas
Capturing User Input
In video games, player interaction is crucial. Capturing user input allows us to move characters, trigger actions, and control game dynamics. In this chapter, we will learn how to capture keyboard and mouse events on the canvas, which will allow us to provide direct control over game elements.
This image shows how both keyboard and mouse input work
Keyboard Events
We can capture keyboard events using the keydown
and keyup
events, which respond when a user presses or releases a key, respectively. This is useful for controlling character movement or responding to key combinations.
Capturing keydown
Events
To detect when a key is pressed, we can add an event listener to the window
object for the keydown
event. Below is a basic example:
javascript
This code listens to the keydown
event and executes an action depending on the pressed key. Here we use arrow keys, but we can capture any key.
Moving the Character with Arrows
We can expand this example to move a character on the canvas. We define variables for the position and adjust the values when the user presses an arrow key:
javascript
In this code, each time the user presses an arrow key, the character's position changes and is updated on the canvas. This creates smooth movement to the left, right, up, and down.
Capturing Mouse Events
Aside from the keyboard, we can also capture mouse events, like click
, mousedown
, mouseup
, and mousemove
. These events are useful for detecting mouse clicks or movements over the canvas.
Click Detection
We can use the click
event to detect when the user clicks on a specific canvas position:
javascript
This code calculates the (x, y)
position of the click on the canvas. Using getBoundingClientRect()
ensures the position is correct even if the canvas isn't at (0, 0)
on the page.
Moving a Character Towards the Click
We can use the click position to move a character to the click location. This adds a new dimension of control to the game:
javascript
This example allows the character to move instantly to the click location, creating a simple and direct control experience.
Drag and Drop on the Canvas
Drag and drop is a common interaction that allows users to move elements on the canvas. We can achieve this by combining mousedown
, mousemove
, and mouseup
events:
javascript
This example allows the user to drag the character across the canvas by clicking and holding the mouse. This type of control is useful for games requiring direct manipulation of elements.
Complete Example: Character Movement and Control
Using what we've learned, we can create a complete control system to allow moving a character with both keyboard and mouse. The following example combines keyboard and click events for versatile control:
javascript
In this example, the character can be controlled with arrow keys or the mouse, enhancing gameplay and interaction on the canvas.
Summary
In this chapter, we've learned to capture keyboard and mouse events on the canvas, allowing us to control the elements of our game. With these concepts, players can interact directly with the characters and objects on screen, making the game more dynamic and immersive.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
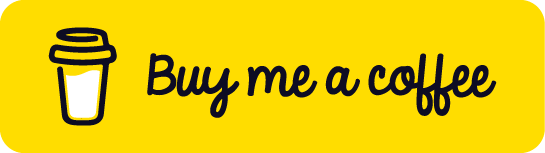
Chat with Chuck
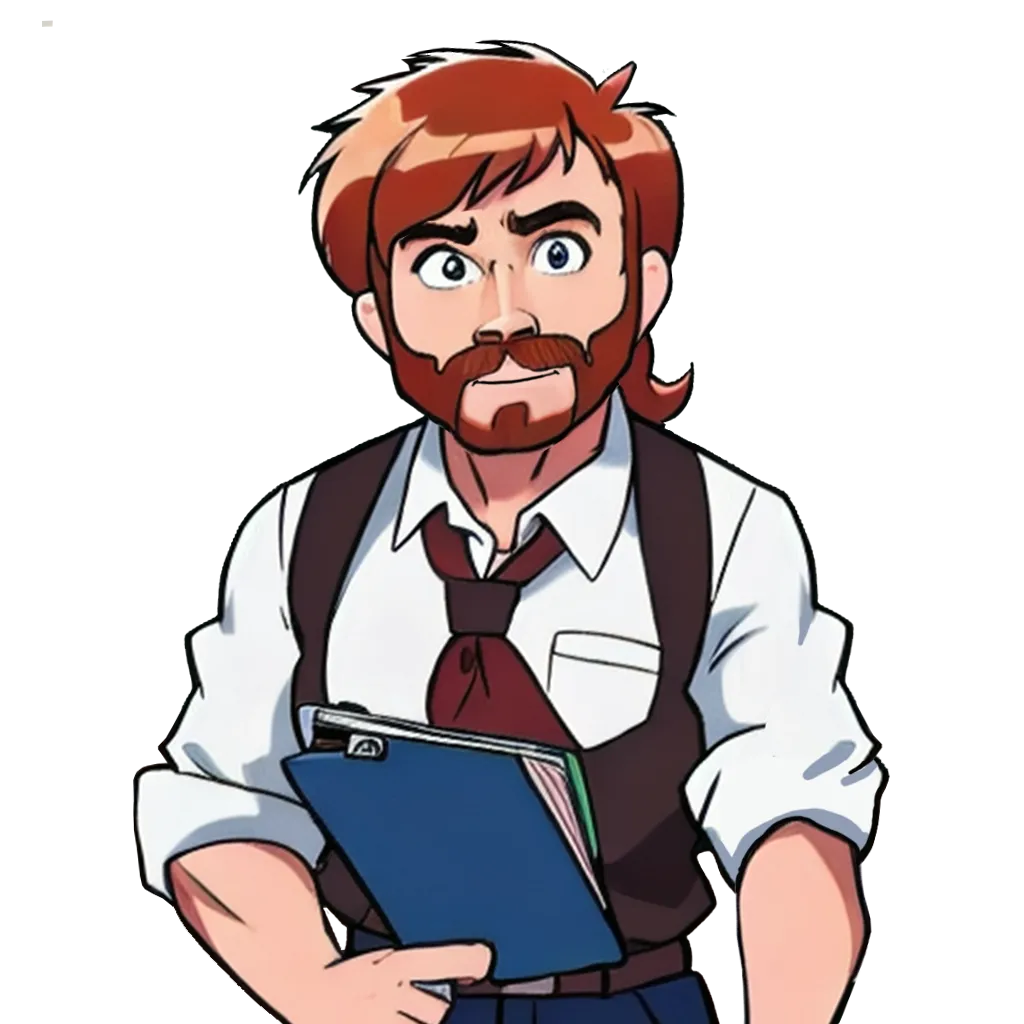
- Introducción a HTML Canvas
- Handling Colors, Strokes, and Fills
- Adding Text to the Canvas
- Capturing User Input
- Handling Images and Sprites in Canvas
- Object Animation in Canvas
- Detección de Colisiones y Lógica de Juego
- Building Game Logic
- Adding Sound Effects and Music
- Enhancing User Interactivity and Visual Experience
- Adding a HUD and Scoreboard
- Optimización del Rendimiento y Compatibilidad del Juego
- Completion and Game Testing
- Game Publishing
- Complete Project – Building the Full Game
- Next Steps