HTML5 Canvas
Adding Sound Effects and Music
In this chapter, we will learn how to incorporate sound effects and music into our game on canvas
, which adds a layer of immersion and enhances the player's experience. We will use the Audio API in JavaScript to control sound playback and its integration into game events.
Playing a Basic Sound
The Audio API in JavaScript allows us to create sounds using the Audio
object. Below, we show how to load and play a sound at the start of the game.
javascript
It is important to ensure that the sound file path is correct and that the format is compatible with browsers.
Adding Sounds to Game Events
To make the game more interactive, we can play specific sounds in response to events such as collisions, score increases, or victory conditions.
Example: Sound on a Collision
Let's imagine we want to play a sound each time two rectangles collide on the canvas
. We will use the collision event we configured earlier.
javascript
Playing Background Music
We can add a background music track that plays continuously while the game is active. To make the music loop, we can enable the loop
property of the Audio
object.
javascript
It is a good practice to provide controls to the player to enable or disable background music.
Volume Control and Muting
To provide a flexible user experience, we can add volume controls and a mute button for players to adjust the audio to their preferences.
Example: Volume Control
We can adjust the volume of sound and music by modifying the volume
property, which accepts values between 0
and 1
.
javascript
Example: Mute Button
We can implement a button that allows players to mute or unmute the sound as they prefer.
javascript
Exercise: Add a Victory Sound
As an exercise, implement a special sound to be played when the player reaches a victory condition, such as reaching a certain score.
javascript
Conclusion
In this chapter, we have learned to add sound effects and music to our canvas game, enhancing the player's experience and increasing immersion.
- Introducción a HTML Canvas
- Handling Colors, Strokes, and Fills
- Adding Text to the Canvas
- Capturing User Input
- Handling Images and Sprites in Canvas
- Object Animation in Canvas
- Detección de Colisiones y Lógica de Juego
- Building Game Logic
- Adding Sound Effects and Music
- Enhancing User Interactivity and Visual Experience
- Adding a HUD and Scoreboard
- Optimización del Rendimiento y Compatibilidad del Juego
- Completion and Game Testing
- Game Publishing
- Complete Project – Building the Full Game
- Next Steps
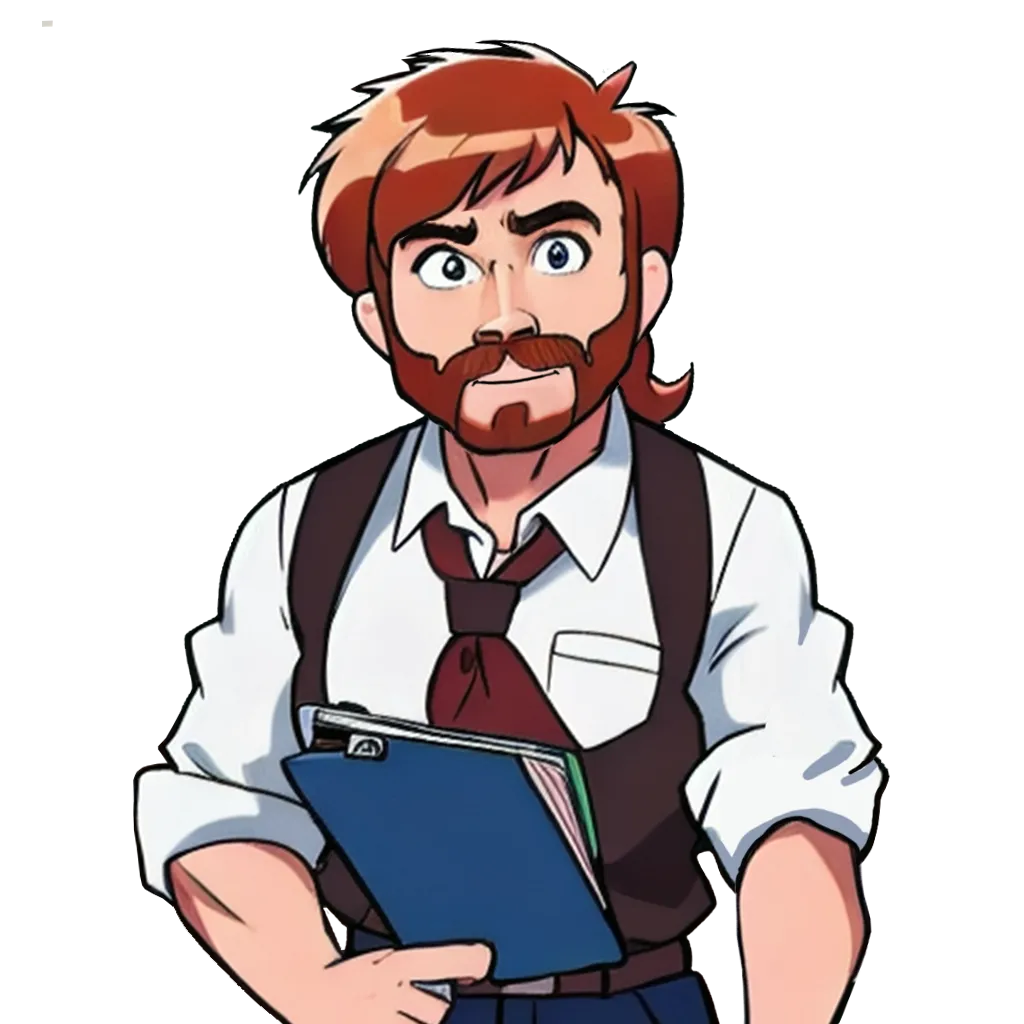