HTML5 Canvas
Complete Project – Building the Full Game
In this final project chapter, we will combine everything we've learned to build a complete game using canvas
. This simple game will incorporate character movement, collision detection, a scoring system, sound effects, and a basic HUD. This final project will allow you to apply and consolidate the knowledge acquired in previous chapters.
Step 1: Setting Up the Canvas and Initial Variables
Let's start by creating the basic HTML file and setting up the canvas
element with the necessary initial variables.
html
Now, in the game.js
file, we initialize the canvas
, the 2D context, and some variables for the player, obstacles, and scoring.
javascript
Step 2: Player Movement
We add keyboard controls to move the player up and down.
javascript
Step 3: Creating and Moving Obstacles
To add obstacles to the game, we create a function that generates obstacles and moves them to the left.
javascript
Step 4: Collision Detection
We implement a function to detect collisions between the player and obstacles.
javascript
Step 5: Scoring and HUD
We increase the score while the game is running and display it on screen.
javascript
Step 6: Game Loop
Finally, we create a game loop that updates and draws all elements on the canvas.
javascript
Step 7: Final Polishing and Testing
We review and test the game to make sure the controls work and the difficulty is appropriate. You can also adjust the player's speed, obstacle creation time, and score increment to balance gameplay.
- Introducción a HTML Canvas
- Handling Colors, Strokes, and Fills
- Adding Text to the Canvas
- Capturing User Input
- Handling Images and Sprites in Canvas
- Object Animation in Canvas
- Detección de Colisiones y Lógica de Juego
- Building Game Logic
- Adding Sound Effects and Music
- Enhancing User Interactivity and Visual Experience
- Adding a HUD and Scoreboard
- Optimización del Rendimiento y Compatibilidad del Juego
- Completion and Game Testing
- Game Publishing
- Complete Project – Building the Full Game
- Next Steps
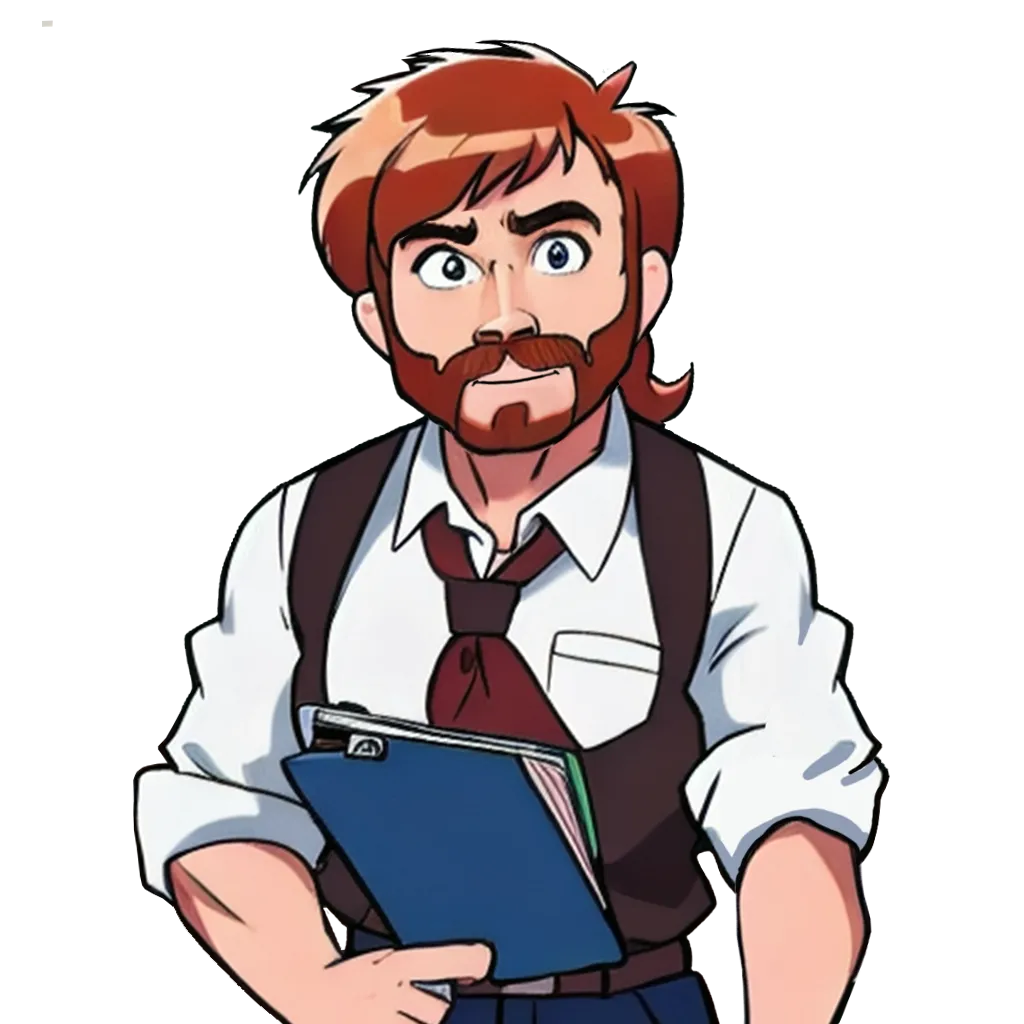