HTML5 Canvas
Adding Text to the Canvas
In games, displaying visual information such as scores, messages, or instructions is essential. The Canvas API allows us to easily add and customize text. In this chapter, we will learn how to use the fillText
and strokeText
methods to draw text on the canvas and explore how to customize the style and alignment of text.
Drawing Text with fillText
To add text to the canvas, we use the fillText
method, which allows us to draw solid text. This method takes three main parameters: the text, the x
coordinate, and the y
coordinate where the text will appear. Here's a basic example:
javascript
In this example, the text "Hello, Canvas!" is drawn at position (100, 100)
with a font size of 20px
and Arial text style. You can change the font and color to customize the text according to your game style.
Drawing Text Outlines with strokeText
If we want to draw only the outline of the text, we can use the strokeText
method. This can be useful for creating text effects or highlighting words on the canvas. Let's look at an example:
javascript
In this case, the text appears with a blue outline instead of being filled, which can be useful for text styles that stand out more in the game.
Setting Font Style and Size
The font
property allows us to customize the style and size of the text. We can specify both the font size and the type in a single value. Here are some style examples:
javascript
These examples demonstrate how to change the style, size, and font of text on the canvas. Using different fonts can enhance the appearance of text elements in the game.
Text Alignment and Positioning
Text alignment on the canvas is managed by the textAlign
property, which defines how the text will be aligned in relation to the specified (x, y)
point. Possible values include "start"
, "end"
, "left"
, "right"
, and "center"
. Here are examples:
javascript
These examples show how to use textAlign
to control the horizontal alignment of text, which is useful for centering text or adjusting it to the sides of the canvas.
Line Height and Text Baseline
We can control the line height of text using textBaseline
, which defines the text baseline relative to the (x, y)
point. Common values include "top"
, "hanging"
, "middle"
, "alphabetic"
, "ideographic"
, and "bottom"
. Here is an example:
javascript
The textBaseline
property allows us to adjust the vertical position of the text to better fit the design of our game.
Complete Example: Displaying a Score
Let's imagine we are developing a game and want to display a score in the upper left corner of the canvas. We can do this using fillText
and customizing the font, color, and position:
javascript
This code displays an initial score of 0
in the upper left corner of the canvas, something common in many games.
Summary
In this chapter, we explored how to add and customize text on the canvas. We learned to use the fillText
and strokeText
methods, and also to adjust the style, size, alignment, and position of the text. These concepts will be fundamental for displaying visual information in our game.
- Introducción a HTML Canvas
- Handling Colors, Strokes, and Fills
- Adding Text to the Canvas
- Capturing User Input
- Handling Images and Sprites in Canvas
- Object Animation in Canvas
- Detección de Colisiones y Lógica de Juego
- Building Game Logic
- Adding Sound Effects and Music
- Enhancing User Interactivity and Visual Experience
- Adding a HUD and Scoreboard
- Optimización del Rendimiento y Compatibilidad del Juego
- Completion and Game Testing
- Game Publishing
- Complete Project – Building the Full Game
- Next Steps
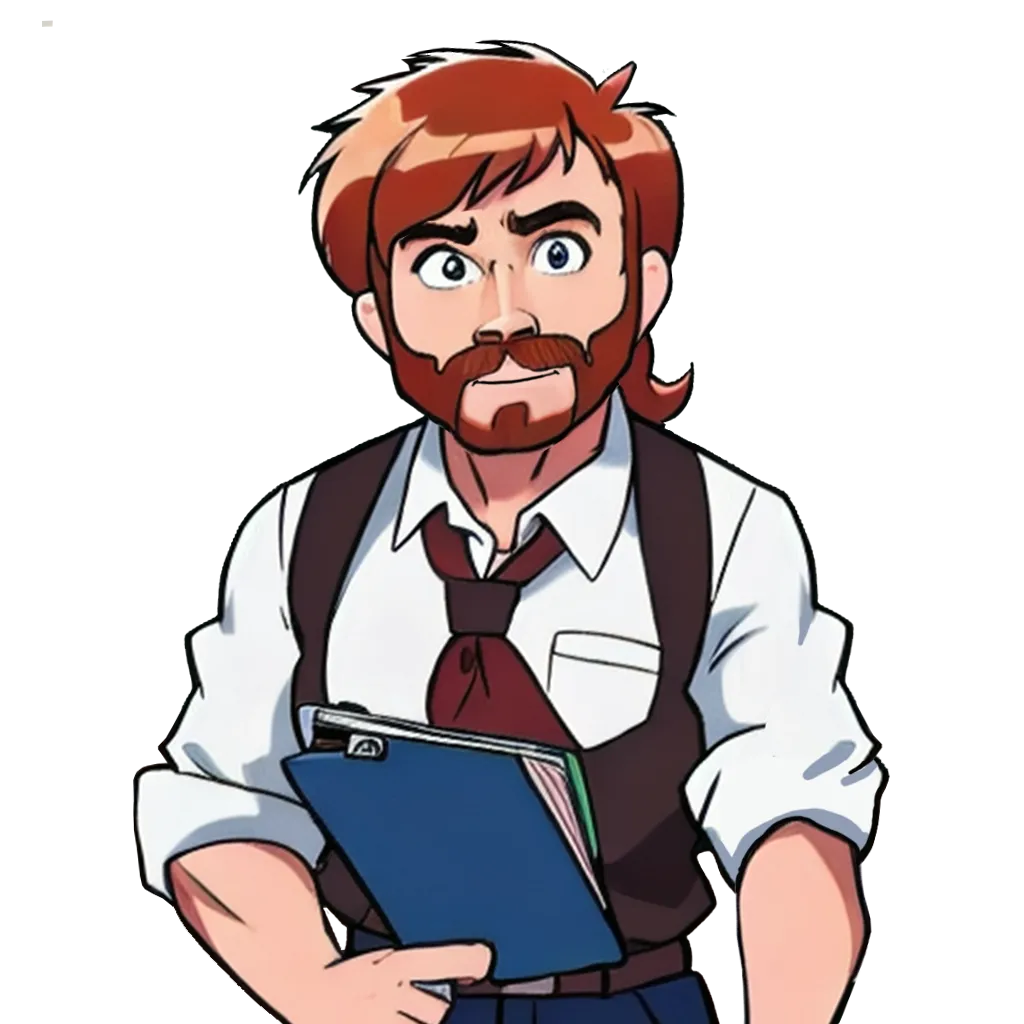