Event Handling in JavaScript
Best Practices in Event Handling
Efficient event handling in JavaScript is crucial for creating interactive, fast, and maintainable web applications. Following some best practices can help you avoid common issues and write cleaner, more efficient code. In this chapter, we will explore the best practices for event handling in JavaScript.
1. Use Event Delegation
Event delegation is a powerful technique that improves performance and simplifies code by attaching a single event listener to a common ancestor, rather than attaching multiple listeners to multiple child elements.
Example:
html
2. Remove Event Listeners
Adding event listeners can have an impact on performance and memory management. Make sure to remove event listeners when they are no longer needed.
Example:
html
3. Avoid Multiple Event Listeners
Instead of adding multiple listeners to the same element, consider using a single listener to handle different types of events.
Example:
html
4. Use Descriptive Names for Handler Functions
Use descriptive handler function names that clearly indicate what the event does.
Example:
html
5. Avoid Using inline
JavaScript
Avoid adding event listeners directly in HTML attributes. Instead, use addEventListener
for better separation of content and behavior.
Example:
html
6. Avoid Blocking the UI in Event Handlers
Do not perform heavy or blocking operations in event handlers. Use setTimeout
or requestAnimationFrame
for long processes.
Example:
html
7. Prioritize Accessibility
Ensure that interactive elements can be accessed and used by all users, including those using assistive technologies.
Example:
html
Placeholder for image
Conclusion
Following these best practices will not only improve the performance of your web applications but will also make your code cleaner and more maintainable. By using techniques like event delegation, properly removing listeners, and ensuring interfaces are accessible, you will be well on your way to developing more robust and inclusive applications.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
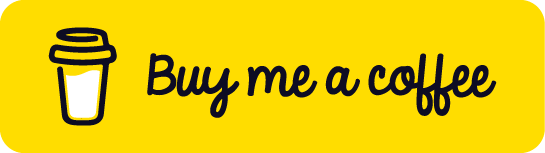
Chat with Chuck
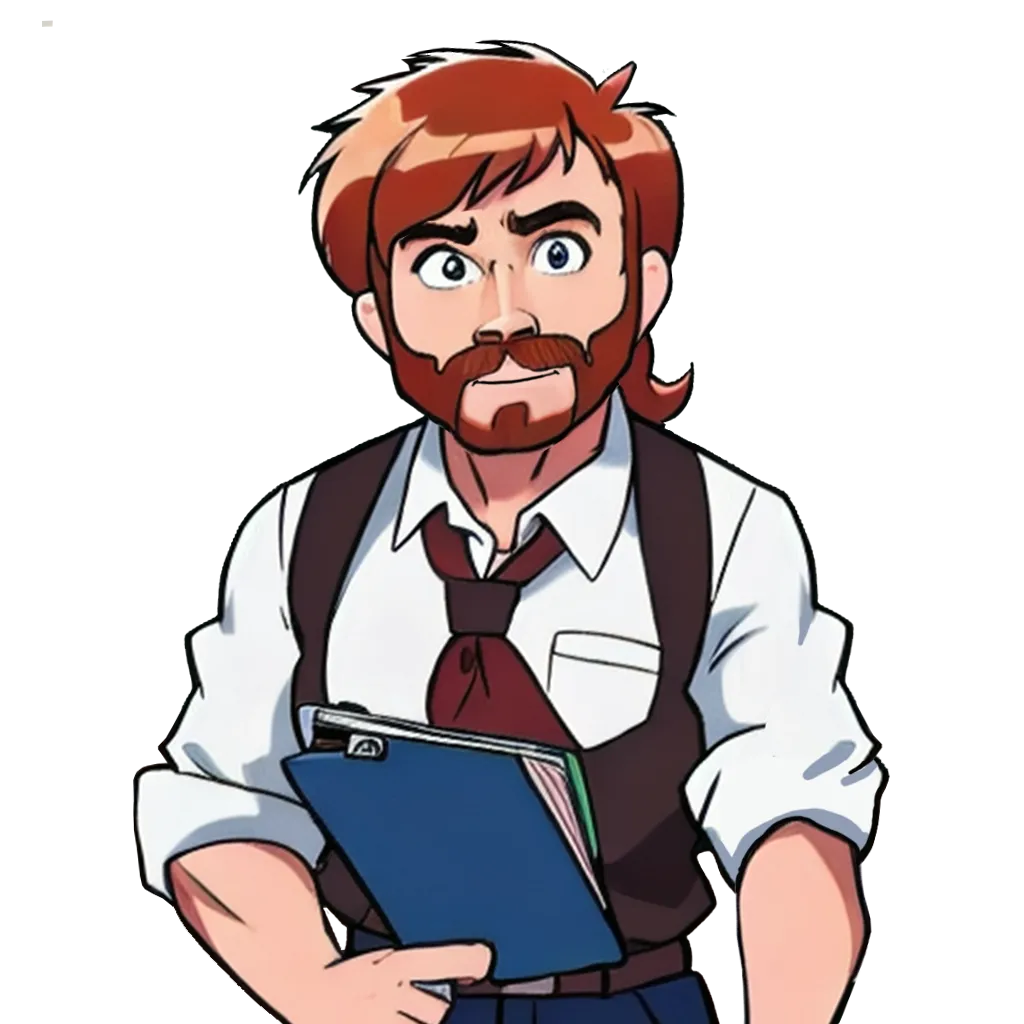
- Introduction to Event Handling in JavaScript
- Types of Events in JavaScript
- Mouse Events
- Keyboard Events
- Form Events
- Loading and Unloading Events
- Focus and Blur Events
- Time Events
- Event Delegation
- Event Propagation and Bubbling
- Preventing Default Events
- Custom Events
- Event Handling with jQuery
- Best Practices in Event Handling
- Conclusion and Next Steps in Event Handling in JavaScript