Event Handling in JavaScript
Event Propagation and Bubbling
Event propagation is a fundamental concept in JavaScript that defines how events propagate through the Document Object Model (DOM). Understanding how events propagate is crucial for effectively handling user interaction and event delegation. In this chapter, we will explore event propagation, including the bubbling phase and how it can be controlled.
What is Event Propagation?
When an event occurs on a DOM element, it doesn't just stay there. Instead, the event passes (or "propagates") through a series of stages from the element that triggered it to its ancestor elements. There are three main phases of event propagation:
- Capturing phase: The event propagates from the
Window
object down to the event target. - Target phase: The event reaches the target element where it occurred.
- Bubbling phase: The event propagates back up from the target to the
Window
object.
Bubbling Phase
The bubbling phase is where most events are typically handled. In this phase, the event propagates from the target element to its ancestor elements, allowing each of them to respond to the event.
Basic Example:
html
In this example, clicking the button triggers both alerts because the event bubbles from the button to the container div.
Capturing Phase
The capturing phase allows intercepting events before they reach the target element. To use this phase, a third argument (true
) is passed to addEventListener
.
Capturing Example:
html
In this example, the alert for the container div will fire before the button’s alert due to the use of the capturing phase.
Stopping Propagation
In certain cases, it may be necessary to stop an event's propagation so that it does not reach other ancestor elements. This is achieved by using the stopPropagation()
method on the event object.
Example:
html
Here, clicking the button will only show the button alert because stopPropagation()
prevents the event from bubbling up to the container.
Stop Immediate Propagation
To prevent other listeners of the same event from executing on the same element, you can use stopImmediatePropagation()
.
Example:
html
In this case, only the second alert will execute.
Image Placeholder
Conclusion
Understanding and managing event propagation is essential for controlling how and when events are handled on a web page. The ability to stop and modify event propagation provides fine control over user interaction and website functionality, allowing you to create more intuitive and efficient user experiences.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
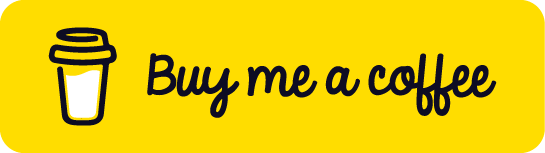
Chat with Chuck
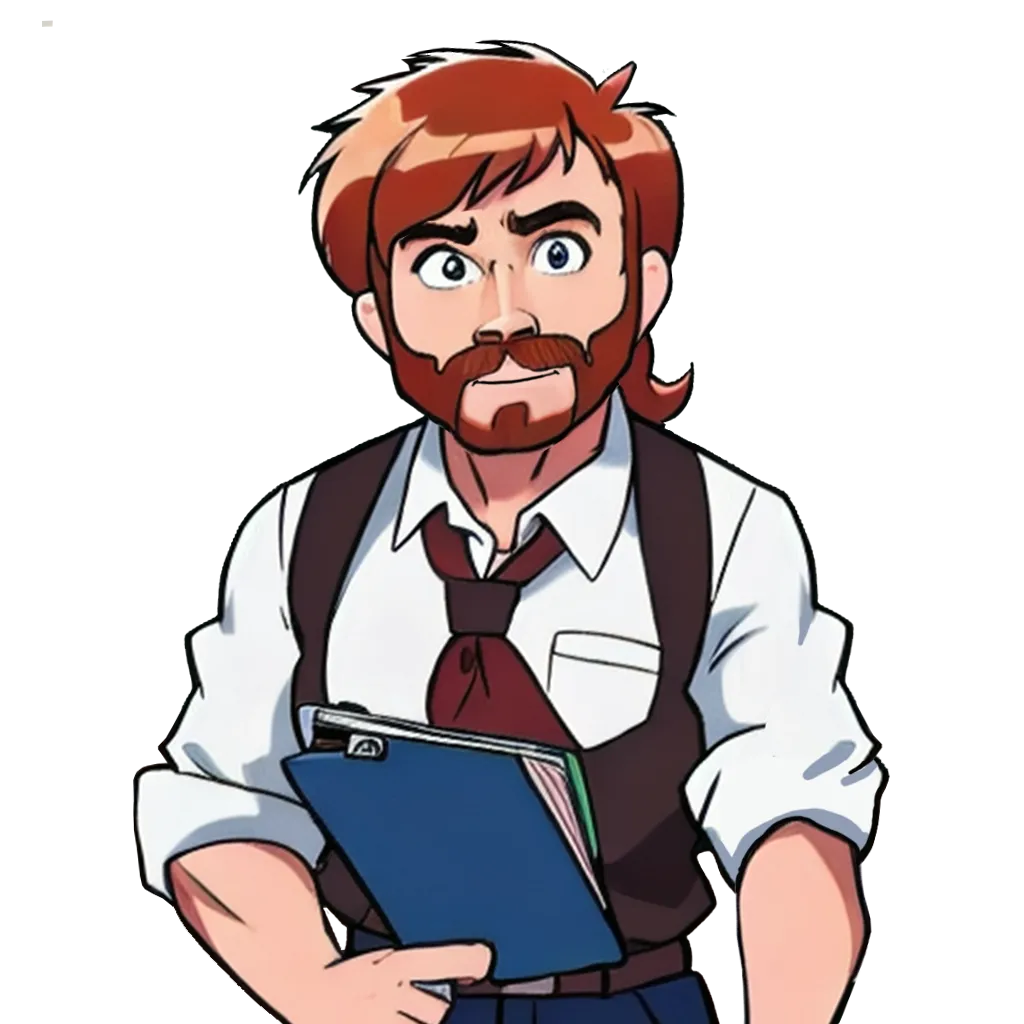
- Introduction to Event Handling in JavaScript
- Types of Events in JavaScript
- Mouse Events
- Keyboard Events
- Form Events
- Loading and Unloading Events
- Focus and Blur Events
- Time Events
- Event Delegation
- Event Propagation and Bubbling
- Preventing Default Events
- Custom Events
- Event Handling with jQuery
- Best Practices in Event Handling
- Conclusion and Next Steps in Event Handling in JavaScript