Event Handling in JavaScript
Keyboard Events
Keyboard events in JavaScript are especially useful for capturing and responding to user interactions with the keyboard. These events are commonly used in forms, web games, accessibility, and many other areas where user input through the keyboard is essential. Below, the most common keyboard events and how to handle them in JavaScript are described.
Common Keyboard Events
keydown
The keydown
event is triggered when a key is pressed.
Example:
html
keyup
The keyup
event is triggered when a key is released.
Example:
html
keypress
The keypress
event is triggered when a key is pressed and released (this event is considered obsolete and it is preferable to use keydown
and keyup
).
Example:
html
Useful Keyboard Event Properties
- event.key: Returns the value of the key pressed, such as "a", "Enter", or "Esc".
- event.code: Returns the physical code of the key on the keyboard, such as "KeyA", "Enter", or "Escape".
- event.shiftKey, event.ctrlKey, event.altKey, event.metaKey: Return
true
if the Shift, Ctrl, Alt, or Meta keys were pressed when the event was triggered.
Advanced Example:
html
Detecting Text Input and Validation
Keyboard events are very useful for performing real-time validation as the user types in a text field.
Real-Time Validation Example:
html
Compatibility Considerations
As with other events, it is important to note that keyboard events may behave differently in different browsers and devices. For example, some mobile keyboards may not trigger the same events as physical keyboards.
Placeholder for image
Conclusion
Handling keyboard events in JavaScript is essential for creating interactive and accessible web applications. With these events, you can capture and respond to user actions, providing a richer and more dynamic user experience.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
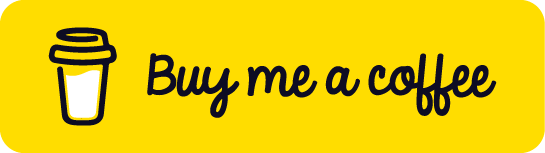
Chat with Chuck
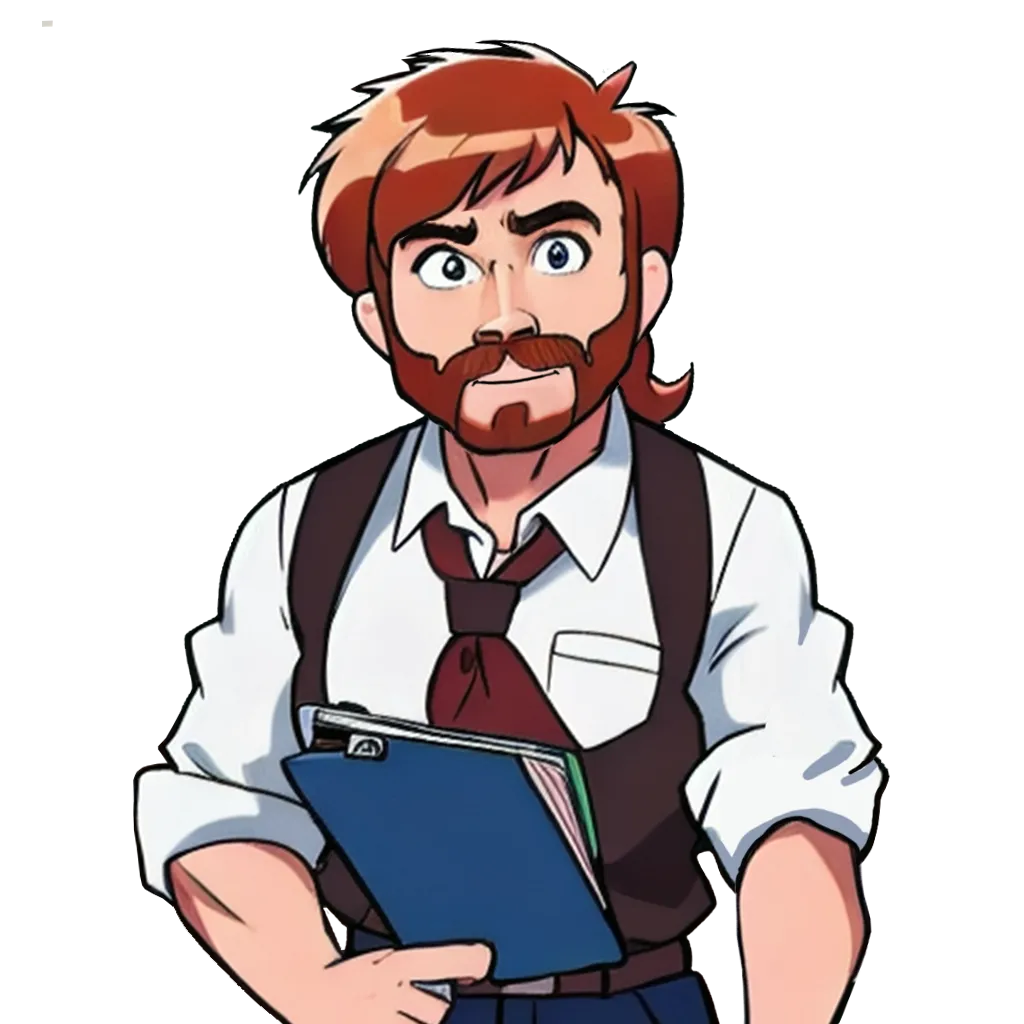
- Introduction to Event Handling in JavaScript
- Types of Events in JavaScript
- Mouse Events
- Keyboard Events
- Form Events
- Loading and Unloading Events
- Focus and Blur Events
- Time Events
- Event Delegation
- Event Propagation and Bubbling
- Preventing Default Events
- Custom Events
- Event Handling with jQuery
- Best Practices in Event Handling
- Conclusion and Next Steps in Event Handling in JavaScript