Event Handling in JavaScript
Loading and Unloading Events
Loading and unloading events are essential in JavaScript for managing the initialization and cleanup of resources on a web page. These events allow you to execute functions when the page or a specific resource has finished loading, as well as perform tasks when the page is about to be left. Below are the most common loading and unloading events and how to handle them.
Common Loading Events
load
The load
event is triggered when a resource has been completely loaded. This event can be applied to the browser window and individual elements like images, iframes, and scripts.
Example (In the window):
html
Example (In an image):
html
Example (In an iframe):
html
Common Unloading Events
unload
The unload
event is triggered when the page is unloading, i.e., when the user leaves the page or closes the browser. This event is less frequently used now and has largely been replaced by the beforeunload
event.
Example:
html
beforeunload
The beforeunload
event is triggered before the page begins the unloading process. This event allows asking the user if they really want to leave the page, which is useful for preventing the loss of unsaved data.
Example:
html
Common Applications of Loading and Unloading Events
Preloading Resources
The load
event is useful for ensuring that all necessary resources are available before executing certain code.
Example:
html
Auto-Saving
The beforeunload
event can be useful for implementing an auto-save feature or for reminding the user to save their work before leaving the page.
Example:
html
Placeholder for Image
[Placeholder: Diagram showing the lifecycle of a web page, highlighting the points where load
, unload
, and beforeunload
events are triggered.]
Conclusion
Handling loading and unloading events is crucial for controlling the behavior of your web application throughout its lifecycle. These events allow you to ensure that all necessary resources have been loaded and to clean up or save data before the user leaves the page.
- Introduction to Event Handling in JavaScript
- Types of Events in JavaScript
- Mouse Events
- Keyboard Events
- Form Events
- Loading and Unloading Events
- Focus and Blur Events
- Time Events
- Event Delegation
- Event Propagation and Bubbling
- Preventing Default Events
- Custom Events
- Event Handling with jQuery
- Best Practices in Event Handling
- Conclusion and Next Steps in Event Handling in JavaScript
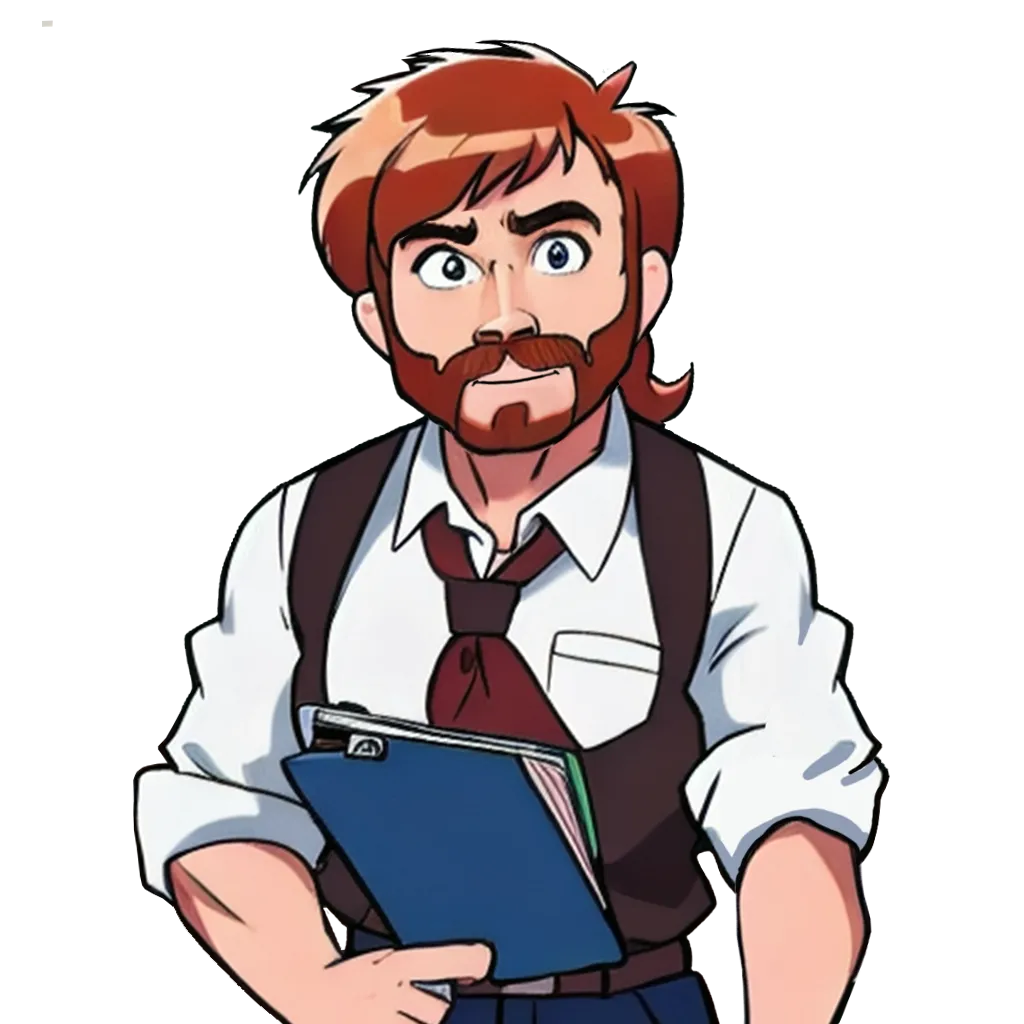