Event Handling in JavaScript
Custom Events
Custom events are a powerful feature in JavaScript that allows you to define and invoke your own events instead of relying solely on native DOM events. They are very useful for creating modular and reusable components and for facilitating communication between different parts of your application. In this chapter, we will learn how to create and handle custom events.
Creating Custom Events
To create a custom event in JavaScript, you can use the CustomEvent
constructor. This constructor allows you to define a new event and provide custom data through the detail
property.
Basic Example:
html
Advanced Usage of Custom Events
Example with Communication between Components:
Imagine a scenario where we have two components, and one needs to notify the other when a certain action occurs.
html
Properties of Custom Events
When creating a custom event, you can define additional properties to control how the event behaves.
- bubbles: Determines whether the event bubbles. Default value is
false
. - cancelable: Determines whether the event can be canceled. Default value is
false
. - detail: An object containing custom data about the event.
Example with Additional Properties:
html
Dispatching Events
The dispatchEvent
method is used to dispatch (trigger) events on an element. This will trigger any event listeners that are attached to the specified event type.
Example of Dispatching an Event:
html
Placeholder for image
[Placeholder: Diagram illustrating the flow of creating, listening, and dispatching a custom event with explanations of the bubbles
, cancelable
, and detail
properties.]
Conclusion
Custom events in JavaScript provide a powerful and flexible way to handle communication between different parts of your application. By using CustomEvent
, dispatchEvent
, and the various associated properties, you can create more modular and manageable applications, enabling more intuitive and efficient interaction.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
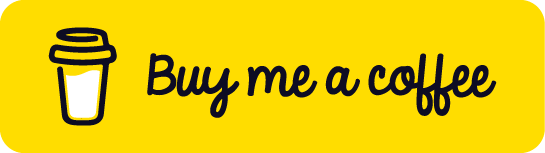
Chat with Chuck
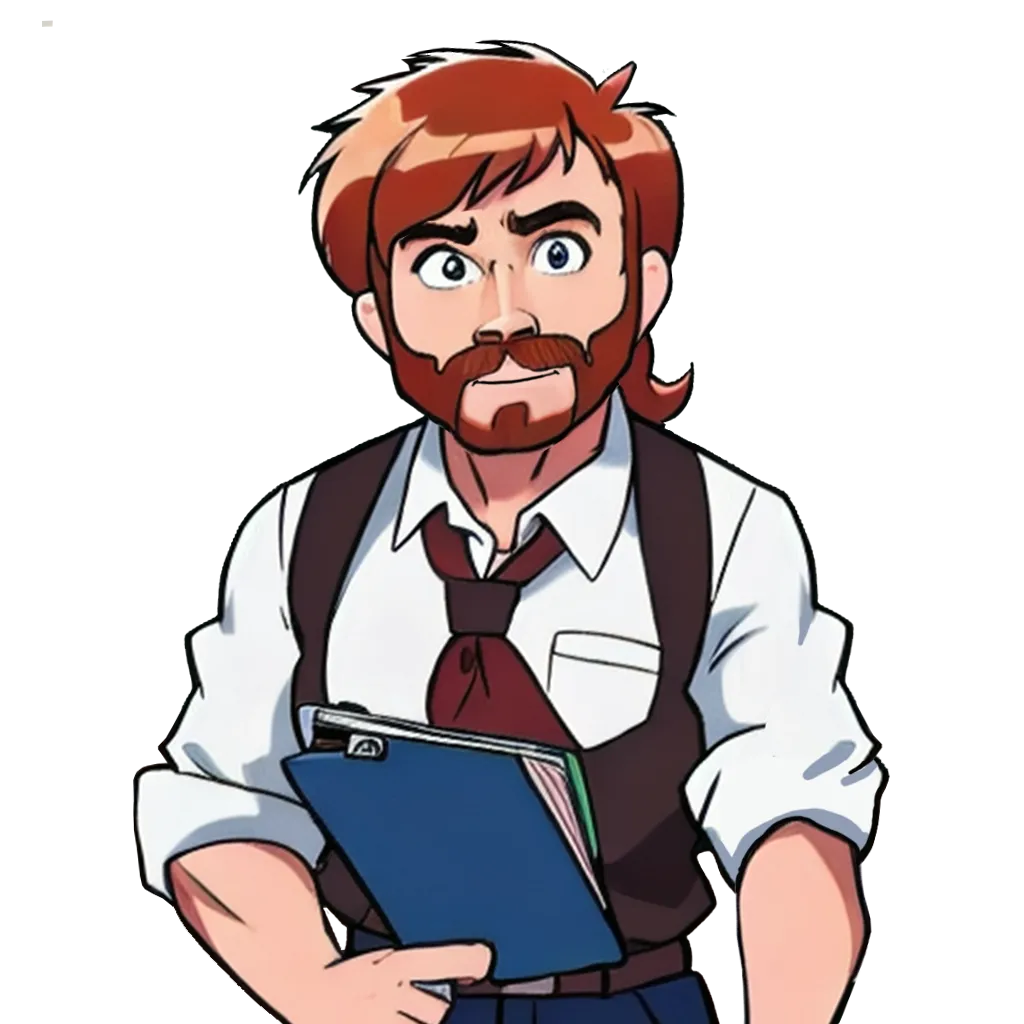
- Introduction to Event Handling in JavaScript
- Types of Events in JavaScript
- Mouse Events
- Keyboard Events
- Form Events
- Loading and Unloading Events
- Focus and Blur Events
- Time Events
- Event Delegation
- Event Propagation and Bubbling
- Preventing Default Events
- Custom Events
- Event Handling with jQuery
- Best Practices in Event Handling
- Conclusion and Next Steps in Event Handling in JavaScript