Event Handling in JavaScript
Conclusion and Next Steps in Event Handling in JavaScript
Throughout this course, we have deeply explored event handling in JavaScript, from basic concepts to advanced techniques. Understanding how events work and how to handle them effectively is fundamental to developing interactive and dynamic web applications.
Summary of What We Learned
- Introduction to Event Handling: Understanding what events are and why they are important in user interaction.
- Types of Events: Exploring common events such as mouse events, keyboard events, form events, and temporal events.
- Mouse and Keyboard Events: Learning to handle events like
click
,mousemove
,keydown
, andkeyup
. - Form Events: Validating and handling data in real-time using events like
submit
,change
,focus
, andblur
. - Load and Unload Events: Using
load
,unload
, andbeforeunload
to handle resource loading and prevent data loss. - Focus and Blur Events: Managing input focus in forms and other elements.
- Time Events: Scheduling actions over time using
setTimeout
andsetInterval
. - Event Delegation: Improving performance and simplifying code by handling events at the level of a common ancestor element.
- Event Propagation and Bubbling: Understanding how event propagation phases work and controlling propagation with
stopPropagation
andstopImmediatePropagation
. - Preventing Default Events: Using
preventDefault
to control the native browser behavior. - Custom Events: Creating and handling custom events using
CustomEvent
. - Event Handling with jQuery: Using jQuery to simplify event handling.
- Best Practices: Following best practices to maintain clean, efficient, and accessible code.
Next Steps
1. Constant Practice
The best way to master event handling in JavaScript is through constant practice. Create small projects or contribute to open-source projects to apply what you have learned.
2. Explore Frameworks and Libraries
While this course focused on event handling with pure JavaScript and jQuery, there are many other frameworks and libraries that offer advanced patterns and tools for event handling, such as React, Vue, and Angular.
3. Continuous Improvement
The JavaScript ecosystem is constantly evolving. Stay updated with the latest trends and best practices by following blogs, attending conferences, and participating in developer communities.
4. Accessibility and Testing
Ensure your applications are accessible to all users and perform thorough testing to ensure events work correctly across different browsers and devices.
Additional Resources
Placeholder for Image
Conclusion
Effective event handling in JavaScript is a fundamental skill for any web developer. By understanding and applying the techniques covered in this course, you will be well-equipped to create more interactive, efficient, and accessible web applications. Keep practicing and exploring new tools and approaches to continue improving your event handling skills in JavaScript.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
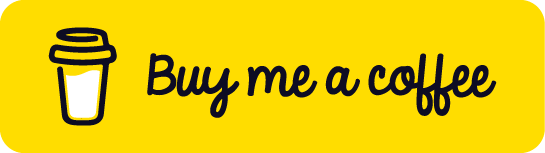
Chat with Chuck
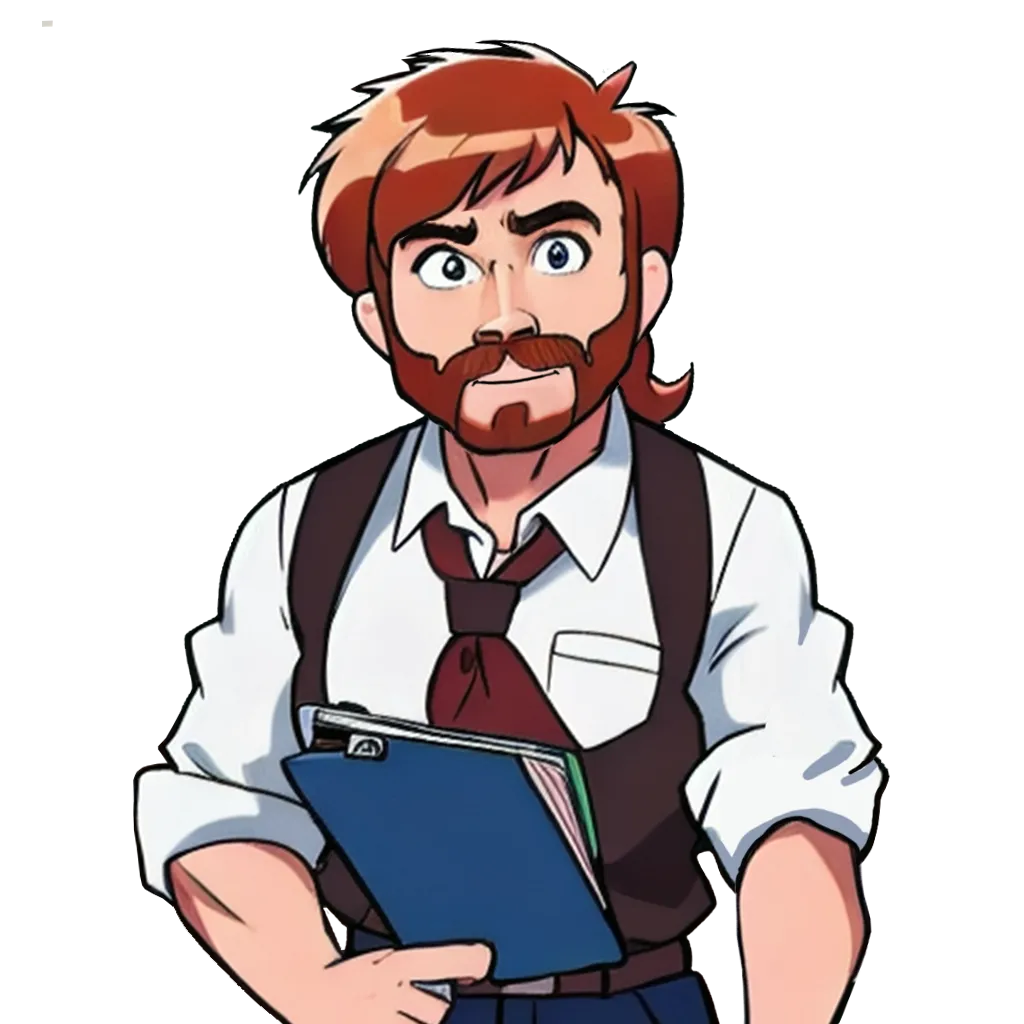
- Introduction to Event Handling in JavaScript
- Types of Events in JavaScript
- Mouse Events
- Keyboard Events
- Form Events
- Loading and Unloading Events
- Focus and Blur Events
- Time Events
- Event Delegation
- Event Propagation and Bubbling
- Preventing Default Events
- Custom Events
- Event Handling with jQuery
- Best Practices in Event Handling
- Conclusion and Next Steps in Event Handling in JavaScript