Event Handling in JavaScript
Event Delegation
Event delegation is an advanced technique in JavaScript that enhances performance and simplifies event handling in web applications. Instead of attaching an event listener to each individual element, a single listener is attached to a common ancestor of these elements. This common listener can capture events occurring in its child elements, even if these elements are added dynamically after the page is loaded.
Why Use Event Delegation?
- Improved Performance: Reducing the number of event listeners improves performance, especially in applications with a large number of interactive elements.
- Simplified Maintenance: Allows for centralized event handling for multiple elements.
- Compatibility with Dynamic Content: Effective for handling events in elements that are dynamically added to the DOM.
How Event Delegation Works
Event delegation relies on the event propagation property in the DOM, also known as "event bubbling." This technique captures events as they propagate from the target element to its ancestor elements.
Basic Example of Event Delegation
Let's assume we have a list of elements and we want to handle clicks on the list elements.
Without Event Delegation:
html
With Event Delegation:
html
Event Delegation with Dynamic Elements
Event delegation is especially useful when working with dynamic content. Event listeners on the ancestor element will capture events on child elements that did not exist when the listener was attached.
Example:
html
Considerations and Best Practices
- Element Verification: Ensure
event.target
is verified before acting on the event. This ensures the function executes only for the desired elements. - Using
closest
: Theclosest
method can help improve the efficiency and readability of the code.html - Event Propagation: In some cases, it may be necessary to stop the event propagation.
html
Placeholder for Image
Conclusion
Event delegation is a powerful and efficient technique that improves the performance and maintainability of your code, especially when working with many interactive elements or dynamic content. Implementing this technique properly can greatly simplify event management in your web applications.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
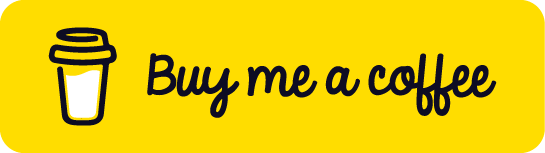
Chat with Chuck
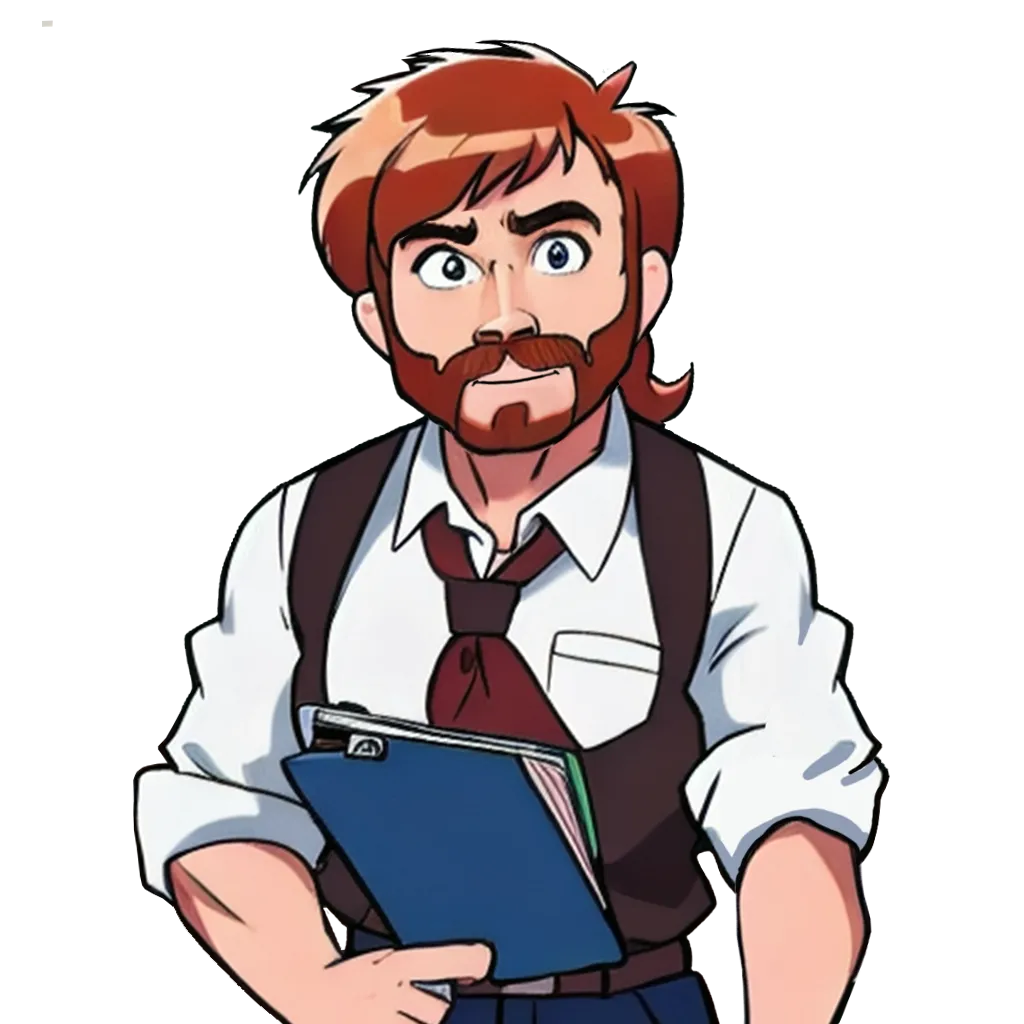
- Introduction to Event Handling in JavaScript
- Types of Events in JavaScript
- Mouse Events
- Keyboard Events
- Form Events
- Loading and Unloading Events
- Focus and Blur Events
- Time Events
- Event Delegation
- Event Propagation and Bubbling
- Preventing Default Events
- Custom Events
- Event Handling with jQuery
- Best Practices in Event Handling
- Conclusion and Next Steps in Event Handling in JavaScript