Event Handling in JavaScript
Introduction to Event Handling in JavaScript
Event handling is one of the most essential and powerful features of JavaScript, especially in the context of web development. Events allow web pages to react to user actions and other triggered events, offering a dynamic and interactive way to enhance the user experience.
What is an Event?
An event is any action or occurrence that happens in the browser environment and can be detected by the JavaScript of our site. Common examples of events include:
- Button click
- Mouse movement
- Key press
- Form submission
- Page load completion
Listening to Events
For our JavaScript code to react to events, we need to "listen" for them. This is commonly done with the addEventListener
function, which is used to register a function as an event listener for a specific event.
Basic Example of an Event Handler
html
In the example above, we are registering a function that displays an alert whenever the button is clicked.
Common Event Types
There are many types of events in JavaScript. Here are some of the most common ones:
- Mouse Events:
click
,dblclick
,mouseover
,mouseout
,mousemove
- Keyboard Events:
keydown
,keyup
,keypress
- Form Events:
submit
,change
,focus
,blur
- Load Events:
load
,unload
,error
Purpose of Event Handling
Event handling is crucial to making web applications interactive and responsive. It allows for immediate actions in response to user interactions, improves accessibility, and provides a rich and dynamic user experience.
Placeholder for Image
In later chapters, we will delve deeper into each event type and learn how to handle them effectively.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
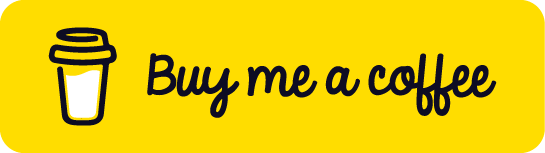
Chat with Chuck
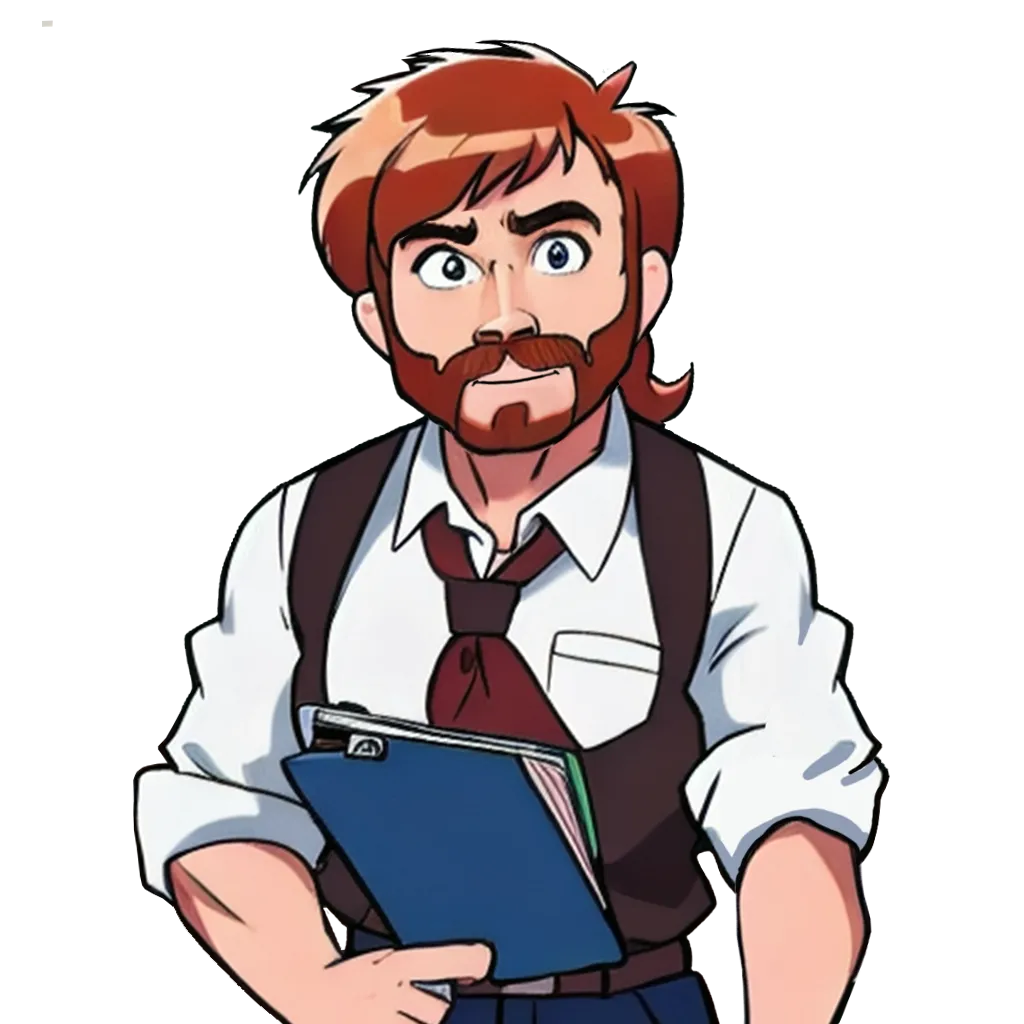
- Introduction to Event Handling in JavaScript
- Types of Events in JavaScript
- Mouse Events
- Keyboard Events
- Form Events
- Loading and Unloading Events
- Focus and Blur Events
- Time Events
- Event Delegation
- Event Propagation and Bubbling
- Preventing Default Events
- Custom Events
- Event Handling with jQuery
- Best Practices in Event Handling
- Conclusion and Next Steps in Event Handling in JavaScript