Event Handling in JavaScript
Types of Events in JavaScript
Events in JavaScript can be classified into several categories based on the nature of the interaction or the source of the event. Knowing the different types of events will allow you to handle user interactions and other occurrences more efficiently and organized.
Mouse Events
Mouse events are those that occur when the user interacts with the mouse. Some of the most common are:
- click: Triggered when an element is clicked.
- dblclick: Triggered when an element is double-clicked.
- mouseover: Triggered when the mouse cursor moves over an element.
- mouseout: Triggered when the mouse cursor leaves an element.
- mousemove: Triggered when the mouse moves within an element.
Example:
html
Keyboard Events
These events occur when the user interacts with the keyboard. The most common include:
- keydown: Triggered when a key is pressed.
- keyup: Triggered when a key is released.
- keypress: Triggered when a key is pressed and released.
Example:
html
Form Events
These events occur mainly in relation to HTML forms. The most used are:
- submit: Triggered when a form is submitted.
- change: Triggered when the value of a form element changes.
- focus: Triggered when an element gains focus.
- blur: Triggered when an element loses focus.
Example:
html
Load Events
These events relate to the loading of resources on the web page.
- load: Triggered when a resource (like an image or script) has been completely loaded.
- unload: Triggered when the page is about to be unloaded.
Example:
html
Focus and Blur Events
These events are activated when an element gains or loses focus.
- focus: Triggered when an element gains focus.
- blur: Triggered when an element loses focus.
Example:
html
Time Events
These events occur in relation to time.
- setTimeout: Executes a function after a specific period of time.
- setInterval: Executes a function repeatedly at specific intervals.
Example:
html
Placeholder for image
These are just some of the types of events that JavaScript offers. Mastering the handling of these events will allow you to create more interactive and dynamic web applications.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
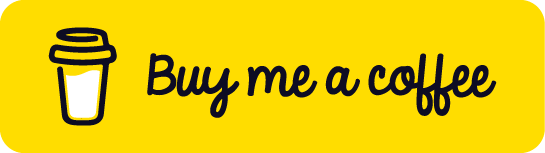
Chat with Chuck
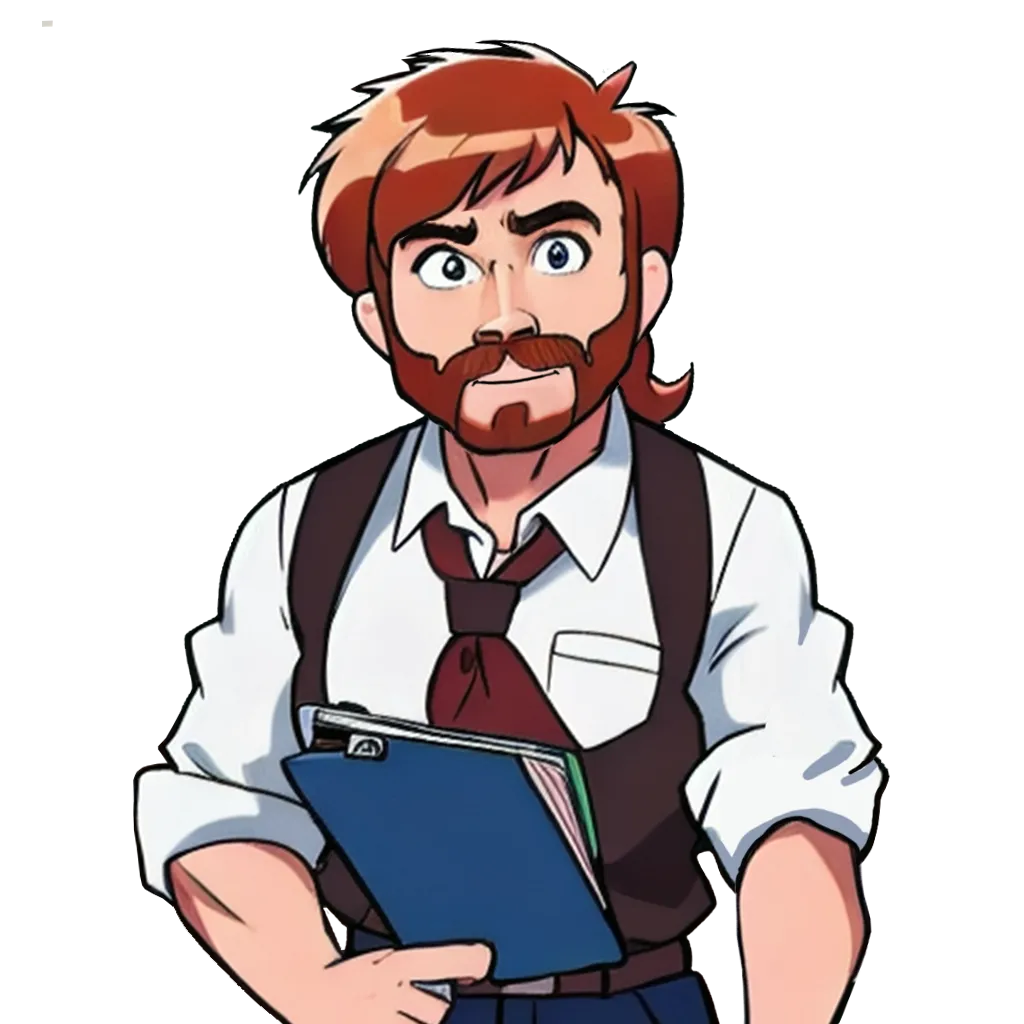
- Introduction to Event Handling in JavaScript
- Types of Events in JavaScript
- Mouse Events
- Keyboard Events
- Form Events
- Loading and Unloading Events
- Focus and Blur Events
- Time Events
- Event Delegation
- Event Propagation and Bubbling
- Preventing Default Events
- Custom Events
- Event Handling with jQuery
- Best Practices in Event Handling
- Conclusion and Next Steps in Event Handling in JavaScript