Event Handling in JavaScript
Event Handling with jQuery
jQuery is a JavaScript library that simplifies DOM manipulation and event handling. It provides an easy-to-use set of methods for working with events, making your code cleaner and easier to maintain. In this chapter, we will explore how to handle events using jQuery.
Introduction to Event Handling with jQuery
jQuery offers methods that allow you to attach, handle, and remove events in a very straightforward manner. The basic syntax for handling events in jQuery is as follows:
javascript
- event: Type of event to handle, such as
click
,focus
,blur
, etc. - childSelector: (Optional) Selector for children elements.
- data: (Optional) Data to be passed to the event handler.
- function: Function to execute when the event occurs.
Common Methods for Event Handling
on
The on
method is used to attach one or more event handlers to the selected elements and their optional children.
Example of on
:
html
Alternative Methods for Shortening on
click
, mouseover
, mouseout
, etc.
jQuery provides specific shorthand methods for common events. These methods are syntactic sugar over the on
method.
Example of click
:
html
Event Delegation
Event delegation in jQuery is easily handled using the on
method with a childSelector
. This is particularly useful for handling events on dynamically added elements.
Event Delegation Example:
html
Removing Event Handlers
off
The off
method is used to remove previously attached event handlers.
Example of off
:
html
Passing Data to Event Handlers
You can pass additional data to your event handlers in jQuery using the data
parameter of the on
method.
Example:
html
Custom Events in jQuery
jQuery also allows you to create and handle custom events easily.
Custom Event Example:
html
Deprecated: bind
, unbind
, delegate
, and undelegate
These methods are older versions of event handling and are considered obsolete in favor of on
and off
. It is best to avoid using them in new projects.
Placeholder for Image
[Placeholder: Flowchart illustrating how on
, off
, event delegation, and custom events work in jQuery with visual examples.]
Conclusion
Using jQuery for event handling can significantly simplify your code and make it cleaner and more maintainable. With methods like on
, off
, and the ability to handle custom events, jQuery offers a robust solution for managing events in your web applications.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
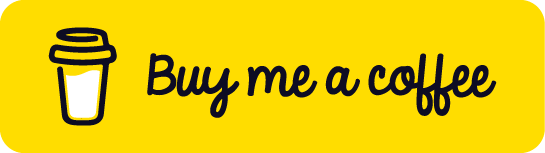
Chat with Chuck
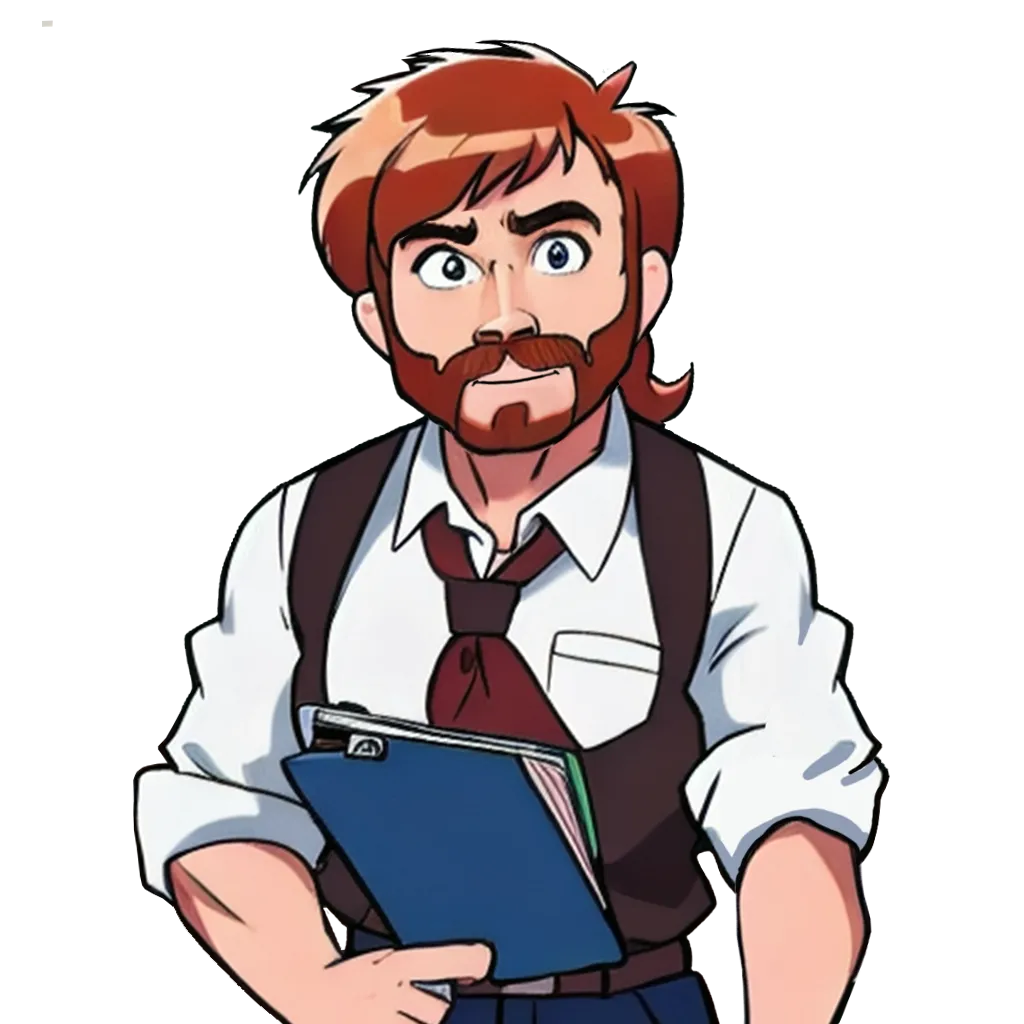
- Introduction to Event Handling in JavaScript
- Types of Events in JavaScript
- Mouse Events
- Keyboard Events
- Form Events
- Loading and Unloading Events
- Focus and Blur Events
- Time Events
- Event Delegation
- Event Propagation and Bubbling
- Preventing Default Events
- Custom Events
- Event Handling with jQuery
- Best Practices in Event Handling
- Conclusion and Next Steps in Event Handling in JavaScript