React Hooks
Custom Hooks
Custom Hooks
Introduction
Custom Hooks are JavaScript functions whose names start with 'use' and that can call other React Hooks. They allow you to extract and reuse state logic between components, making your code more modular and readable.
Creating a Custom Hook
A Custom Hook is simply a function that can use other React Hooks. use
is a required prefix so that React can identify it as a Custom Hook.
javascript
Using the Custom Hook
Once the Custom Hook is created, you can easily use it inside any functional component.
javascript
Advantages of Custom Hooks
- Logic Reusability: You can extract shared logic between components.
- Modularity: Improves code organization and maintainability.
- Separation of Responsibilities: State logic and rendering handled separately.
Advanced Example
Consider a Custom Hook that handles user authentication.
javascript
Using the Advanced Custom Hook
Incorporating the authentication logic in a component.
javascript
Best Practices
- 'use' Prefix in the Name: Ensure the Custom Hook's name starts with 'use' to adhere to React conventions.
- Single Responsibility: Try to make each Custom Hook handle a single responsibility, making the code easier to test and maintain.
- Documentation: Document your Custom Hooks so that other developers can quickly understand what they do and how to use them.
Conclusion
Custom Hooks are a powerful tool that allows you to extract and reuse complex logic in your React applications. By encapsulating logic in custom Hooks, you can enhance the modularity and maintainability of your code, allowing your components to focus on rendering the UI.
Placeholder for image on the creation and use of Custom Hooks: Diagram showing how a Custom Hook is created, how it uses other React Hooks, and how components can use the Custom Hook, indicating the flow of data and logic reuse.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
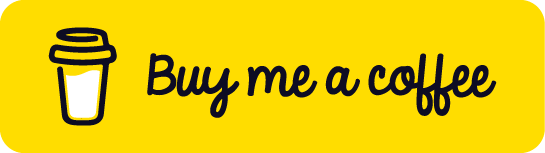
Chat with Chuck
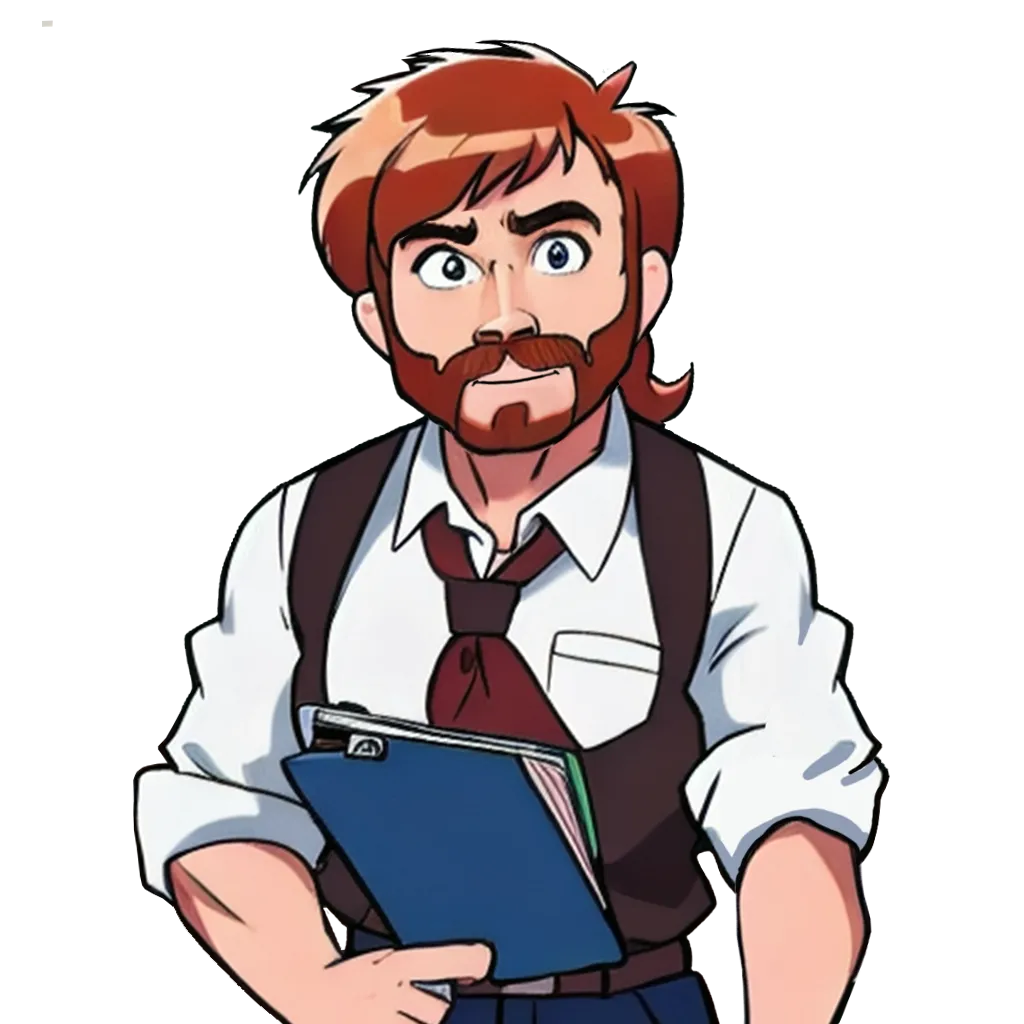