React Hooks
The useLayoutEffect Hook
useLayoutEffect Hook
Introduction
The useLayoutEffect
Hook is similar to useEffect
, but it differs in when it runs. While useEffect
runs after the DOM has been updated, useLayoutEffect
runs synchronously after React has made all the DOM updates but before the browser paints the screen. This makes it particularly useful for reading the DOM and making calculations before the screen is painted by the browser.
Basic Syntax
useLayoutEffect
is used similarly to useEffect
, with an effect function and optionally a list of dependencies.
javascript
Example Description
In this example:
divRef
is a reference created withuseRef
that is assigned to thediv
element.useLayoutEffect
is used to calculate the height of thediv
after it has been updated in the DOM, but before the browser paints the screen.
Use Cases
useLayoutEffect
is particularly useful for:
- Reading and manipulating the DOM: Situations where you need to take measurements of the DOM and apply changes before the paint.
- Synchronizing with other DOM effects: Cases where changes need to be reflected before the browser paints.
Comparison with useEffect
The main difference between useLayoutEffect
and useEffect
is the timing of their execution:
- useEffect: Runs after the browser has painted the screen. Ideal for non-critical effects (data fetching, subscriptions).
- useLayoutEffect: Runs before the paint, after all the DOM modifications. Perfect for synchronized reads and writes to the DOM that need to be done together.
Advanced Example
Consider a case where you need to calculate and adjust visual elements before they are fully rendered.
javascript
Advanced Example Description
In this example:
boxRef
is a reference to thediv
containing horizontally scrollable content.useLayoutEffect
measures thescrollWidth
of thediv
and updates thescrollWidth
state before the browser paints the screen.
Warnings
Although useLayoutEffect
is powerful, it should be used with caution. It was created for cases where you need to read and write to the DOM before the browser paints, but it can slow down the user experience if used incorrectly.
Performance Considerations
Since useLayoutEffect
runs synchronously, it can block the browser's paint if the calculations are heavy. Use it only when absolutely necessary and try to optimize the logic inside the effect.
[Placeholder for image about the use of useLayoutEffect: Diagram showing how useLayoutEffect
runs before the browser's paint, highlighting its difference from useEffect
].
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
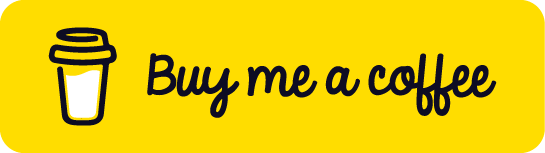
Chat with Chuck
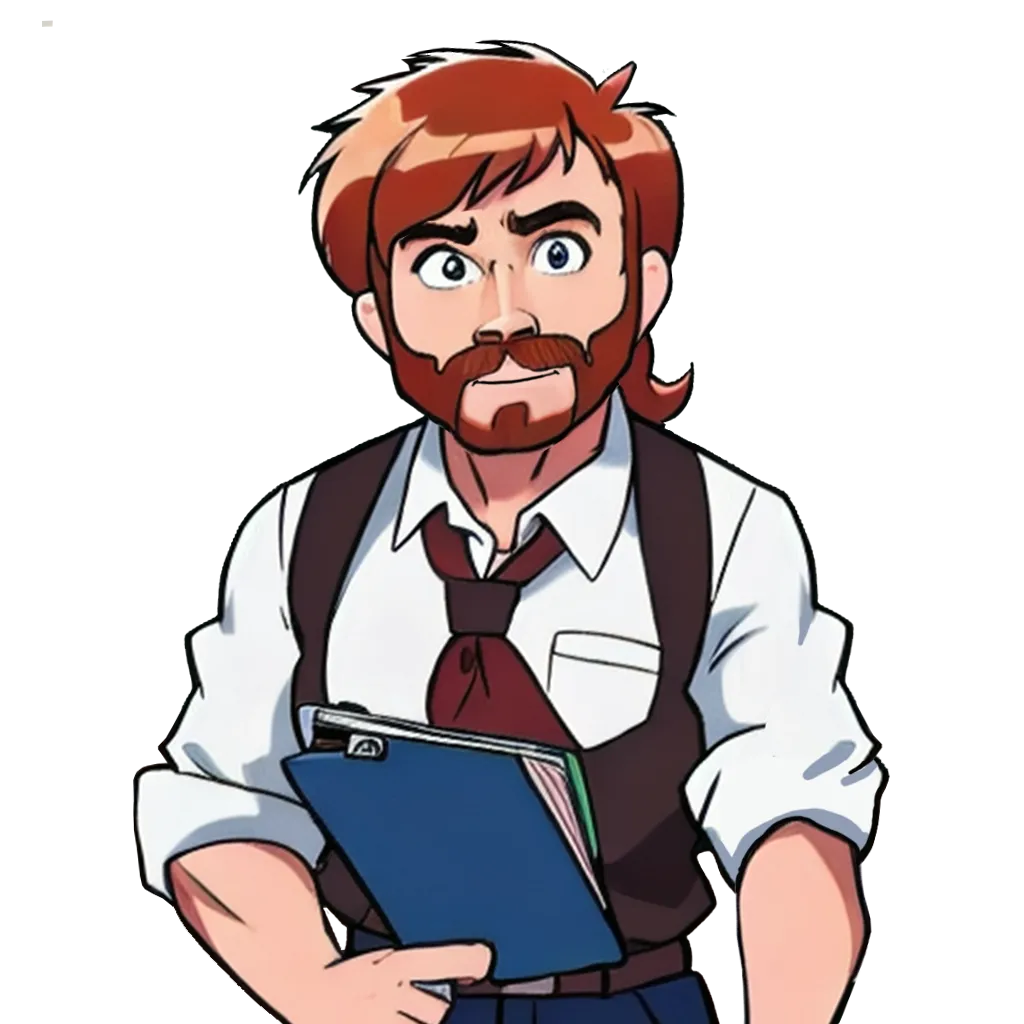