React Hooks
The useImperativeHandle Hook
useImperativeHandle Hook
Introduction
The useImperativeHandle
Hook allows you to customize the ref instance exposed to parent components when using forwardRef
. It is useful when you need to finely control how components handle and expose their references, providing a more specific and controlled API from the child component.
Basic Syntax
useImperativeHandle
is used alongside forwardRef
to expose specific methods of the child component through the provided reference.
javascript
Example Description
In this example:
FancyInput
is a child component that usesforwardRef
to accept a reference from its parent.useImperativeHandle
is used to expose thefocus
andclear
methods through theinputRef
reference.ParentComponent
interacts withFancyInput
by calling thefocus
andclear
methods viafancyInputRef
.
Use Cases
useImperativeHandle
is useful when:
- You need to expose specific functionalities: Providing a controlled API from child components to their parents.
- Detailed control of references: Managing how a reference interacts with internal elements of the component.
Advanced Example
Consider a custom image that needs to be rotated and scaled via exposed methods.
javascript
Advanced Example Description
In this example:
CustomImage
handles an image that can be rotated and scaled using methods exposed throughuseImperativeHandle
.App
contains buttons that interact withCustomImage
via theimageRef
reference, allowing easy rotation and scaling of the image.
Comparison with simply using ref
While you can manipulate DOM elements directly with ref
, useImperativeHandle
provides a more controlled and declarative way of exposing specific functionalities, improving maintainability and clarity of the component.
Warnings
useImperativeHandle
should be used sparingly and when really necessary. Exposing too many internal functionalities can break the encapsulation of the component, making its parts too dependent on each other.
Usage Considerations
- Encapsulation: Expose only what is necessary to maintain the encapsulation and independence of the component.
- Maintainability: Ensure that the exposed methods make sense and are well-documented for future use.
[Placeholder for an image depicting the use of useImperativeHandle: Diagram showing the interaction between forwardRef
, useImperativeHandle
, and how methods from the child component are exposed to the parent component, highlighting the flow of references and methods.]
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
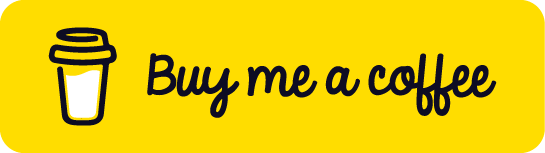
Chat with Chuck
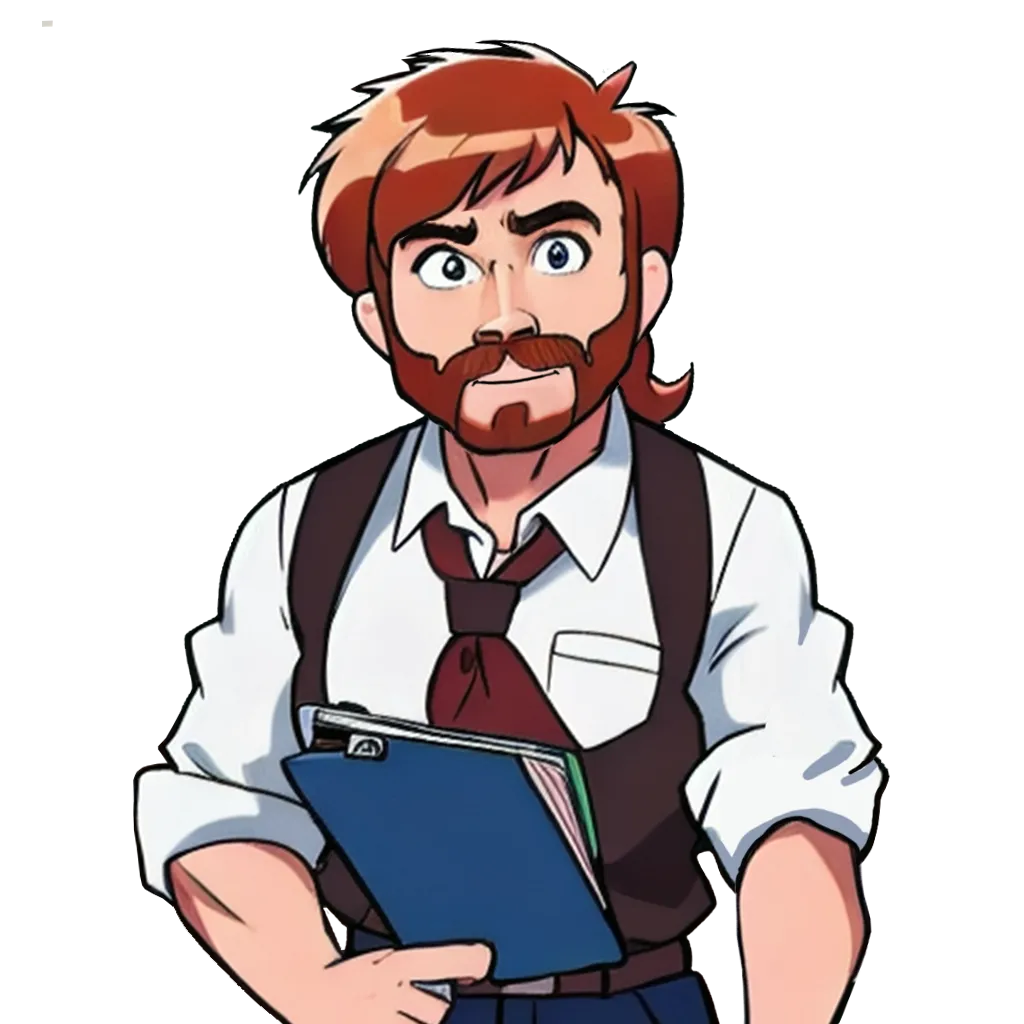