React Hooks
The useMemo Hook
useMemo Hook
Introduction
The useMemo
Hook is used to memoize computed values, optimizing performance by avoiding costly recalculations on every render. This Hook is particularly useful when you need to perform intensive calculations or have components that depend on complex data that should not be recalculated on every render.
Basic Syntax
useMemo
takes a function and a dependencies list, and only recalculates the memoized value when any of the dependencies change.
javascript
Example Description
In this example:
ExpensiveComponent
usesuseMemo
to compute theexpensiveValue
.- The calculation is only performed when
a
orb
change, not on every render of the component. - Even though
App
is re-rendered every timecount
is incremented, the memoized value inExpensiveComponent
is not recalculated unlessa
orb
change.
Use Cases
useMemo
is useful in various scenarios, such as:
- Intensive Calculations: Perform costly calculations only when inputs change.
- Conditional Rendering: Prevent unnecessary renderings of child components.
- List Filtering and Sorting: Memoize results to avoid repeating calculations.
Advanced Example
Consider the case of a filtered list of users.
javascript
Advanced Example Description
In this example:
UsersList
usesuseMemo
to memoize the filtered list of users, avoiding recalculating the filtered list on every render.- The console will display 'Filtering users...' only when the filter changes, optimizing performance.
When Not to Use useMemo
Avoid overusing useMemo
. Use it only when you have clear evidence of performance issues. In most cases, React is efficient at handling component updates on its own.
Warnings
While useMemo
can improve performance, it also adds a layer of complexity. Use it with caution and ensure it is truly benefiting your application's performance.
[Placeholder for image about using useMemo: Diagram showing how useMemo
avoids recalculating values on each render, highlighting the dependency process and memoization of results.]
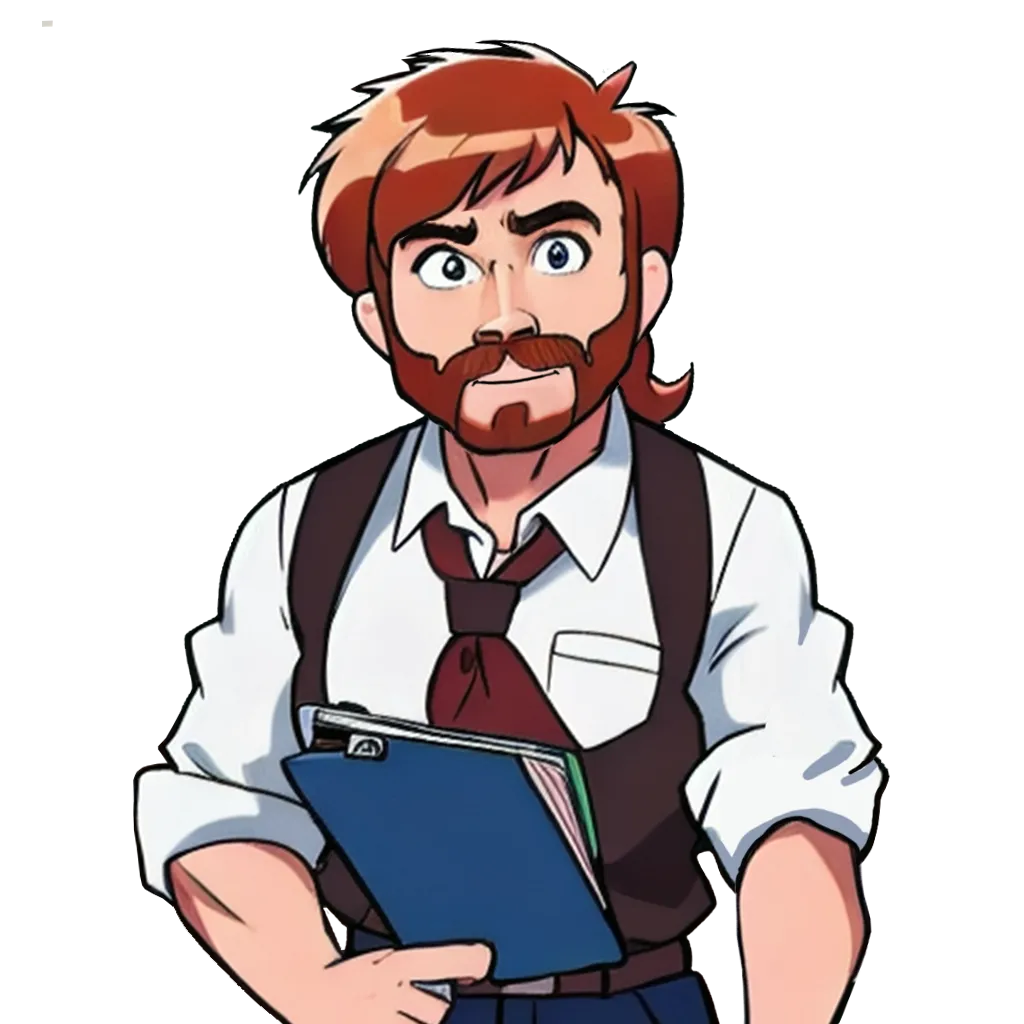