React Hooks
The useState Hook
useState Hook
Introduction
The useState
Hook is one of the most commonly used Hooks in React. It allows you to add state to functional components. By using useState
, you can declare state variables within your functional component and update them as data changes.
Basic Syntax
useState
is invoked with the initial state value and returns an array with two elements: the current state variable and a function to update this variable.
javascript
Example Description
In this example:
useState(0)
declares a new state variablecount
and initializes it to 0.setCount
is the function that updates the state ofcount
. Each time the user clicks the button,setCount
incrementscount
by 1.
Types of Values for State
useState
can handle different types of values:
- Numbers:
useState(0)
- Strings:
useState('Hello')
- Booleans:
useState(true)
- Objects:
useState({ key: 'value' })
- Arrays:
useState([1, 2, 3])
Using Functions to Update State
You can also pass a function to setState
that receives the current state as an argument and returns the new state. This is useful when the new state depends on the previous state.
javascript
In this example, prevCount
is the previous state, and setCount(prevCount => prevCount + 1)
uses that value to compute the new state.
Limitations
It is important to note that useState
does not merge the state when it updates. If the state is an object, you must ensure to keep the properties that you are not updating.
javascript
[Placeholder for image about the useState workflow: Diagram showing the data flow when using the useState
Hook, including state declaration, state update, and component re-rendering.]
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
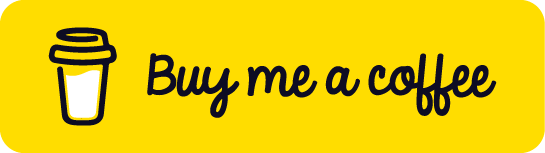
Chat with Chuck
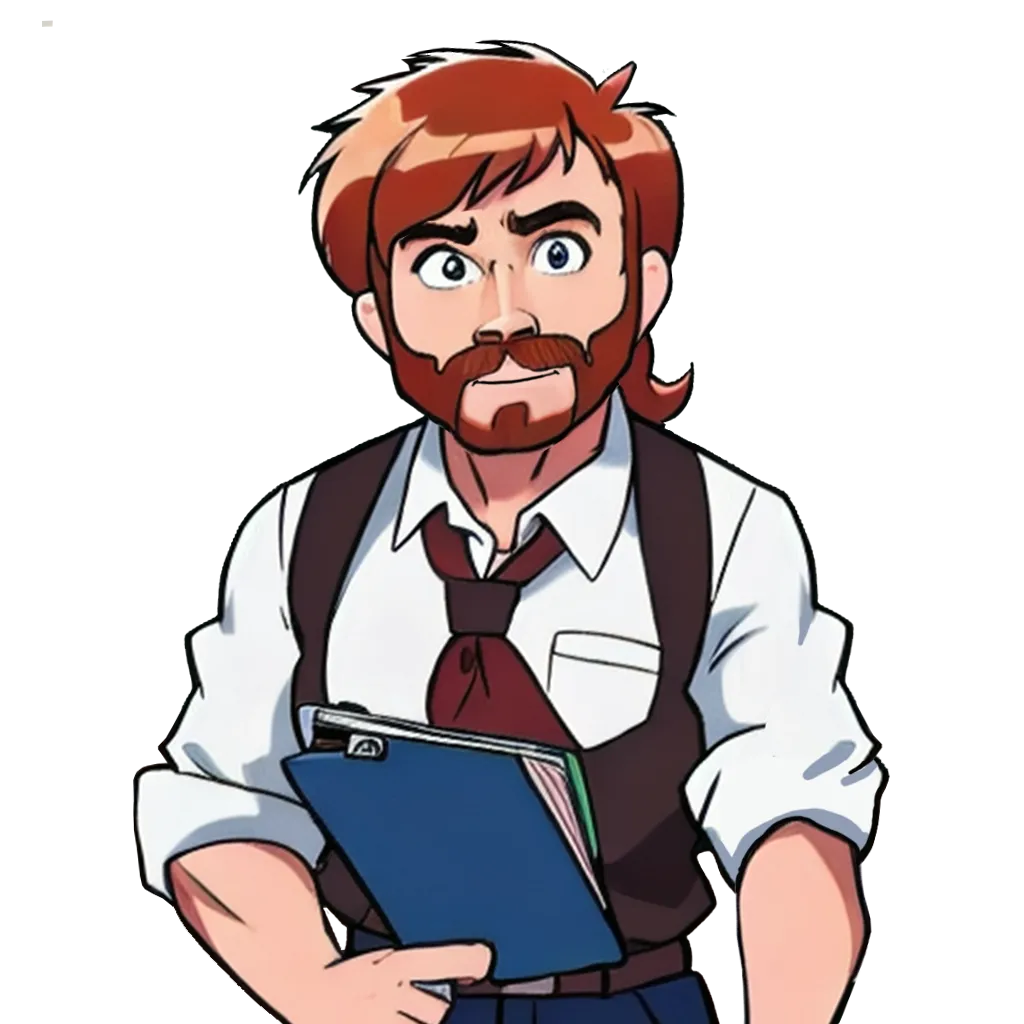