React Hooks
The useId Hook
useId Hook
Introduction
The useId
Hook is a Hook that allows generating unique identifiers that are stable over time, useful for associating components with DOM elements uniquely and avoiding conflicts when rendering multiple instances of a component.
Basic Syntax
useId
is used to create a unique identifier that can be used on DOM elements.
javascript
Example Description
In this example:
useId
generates a unique identifier that is assigned to the<label>
and<input>
tag within theFormField
component.- By instantiating
FormField
multiple times inApp
, each instance will have a unique identifier, avoiding conflicts on theid
andhtmlFor
attributes.
Use Cases
useId
is especially useful in situations where you need to:
- Associate DOM elements: Like
<label>
and<input>
pairs. - Unique identifiers in a list of components: Avoid identifier conflicts in components rendered as a list.
Advanced Example
Consider a form with multiple fields, where each field has its own unique identifier generated with useId
.
javascript
Advanced Example Description
In this example:
TextInput
is a reusable component that generates unique identifiers for its<label>
and<input>
thanks touseId
.SignUpForm
usesTextInput
to create a registration form with unique fields, ensuring no identifier conflicts.
Advantages of using useId
- Guaranteed Uniqueness: Provides unique identifiers that help maintain DOM integrity.
- Simplicity: Makes managing unique identifiers simple and straightforward, without the need for manual solutions.
Warnings
useId
should be used when there's a need to generate unique and stable identifiers; however, it should not be replaced by dynamic identifiers that frequently change in response to user data.
Comparison with Other Solutions
useId
is preferable over manual solutions that use Math.random()
or libraries like uuid
when uniqueness needs to be guaranteed in the context of React rendering.
Usage Considerations
Ensure to use useId
in scenarios where a unique identity is needed for element interaction, such as in accessible forms linking labels and input fields.
Conclusion
The useId
Hook facilitates the generation of unique identifiers, improving accessibility and avoiding conflicts in DOM elements. It is an efficient tool for managing unique identities in React components.
[Placeholder for image on the use of useId: Diagram illustrating how useId
generates unique identifiers for DOM elements, highlighting the stability and uniqueness generated for multiple instances of a component.]
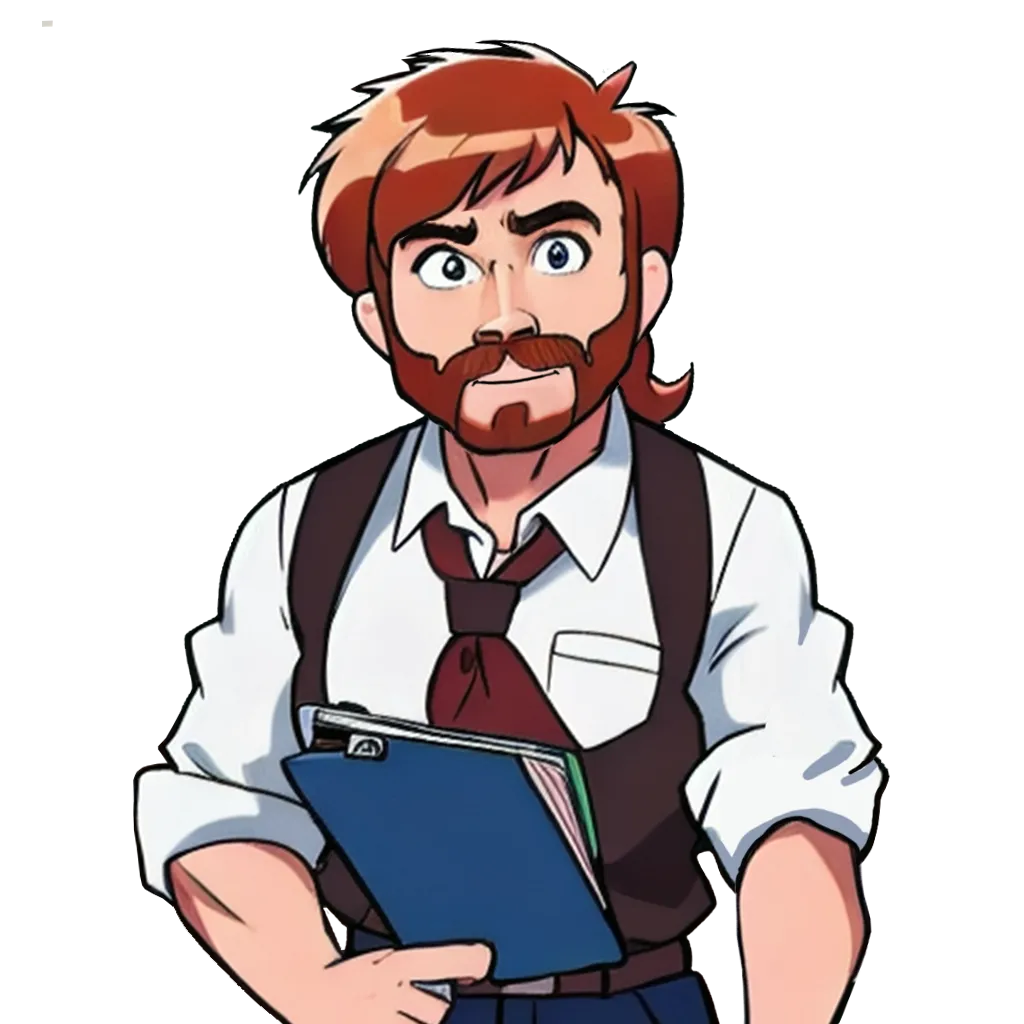