React Hooks
useRef Hook
useRef Hook
Introduction
The useRef
Hook allows you to create a mutable reference that persists throughout the component's lifecycle. It is useful for accessing and manipulating DOM elements directly, maintaining any mutable value that does not cause a re-render when it changes, and storing previous values between renders.
Basic Syntax
useRef
is used to create a reference and can be assigned to DOM elements.
javascript
Example Description
In this example:
inputEl
is a reference created withuseRef
.inputEl
is assigned to theinput
element, soinputEl.current
points to the DOM node.- The function
onButtonClick
focuses the input by callinginputEl.current.focus()
.
Other useRef Applications
useRef
is not only for manipulating the DOM; it can also hold any mutable value between renders that does not cause a re-render when it changes.
javascript
Advanced Example Description
In this example:
countRef
is used to store the current value ofcount
so that thesetInterval
interval always has the latest value without needing to be stopped and restarted.
Common useRef Uses
- Direct access to DOM elements: Focusing inputs, stopping animations, manipulating canvas, etc.
- Storing previous instance values: Keeping a reference to previous values without causing a re-render.
- Holding any mutable value: Ideal for storing data that needs to persist through renders but should not cause a re-render.
Difference between useRef and useState
While useState
causes the component to re-render when its value changes, useRef
does not. This makes useRef
more suitable for values that change frequently and do not need to trigger re-renders, such as interval IDs or any other mutable reference.
Warnings
Although useRef
is powerful, it should be used with caution: it can lead to difficult-to-debug behavior if combined with uncontrolled DOM mutations or complex application logic.
[Placeholder for an image about the use of useRef: Diagram showing how useRef
interacts with the DOM, including the creation of references and their use in direct DOM manipulations.]
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
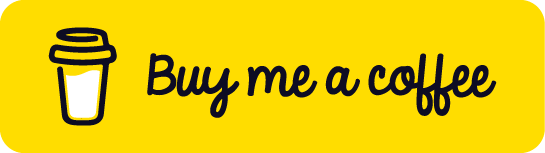
Chat with Chuck
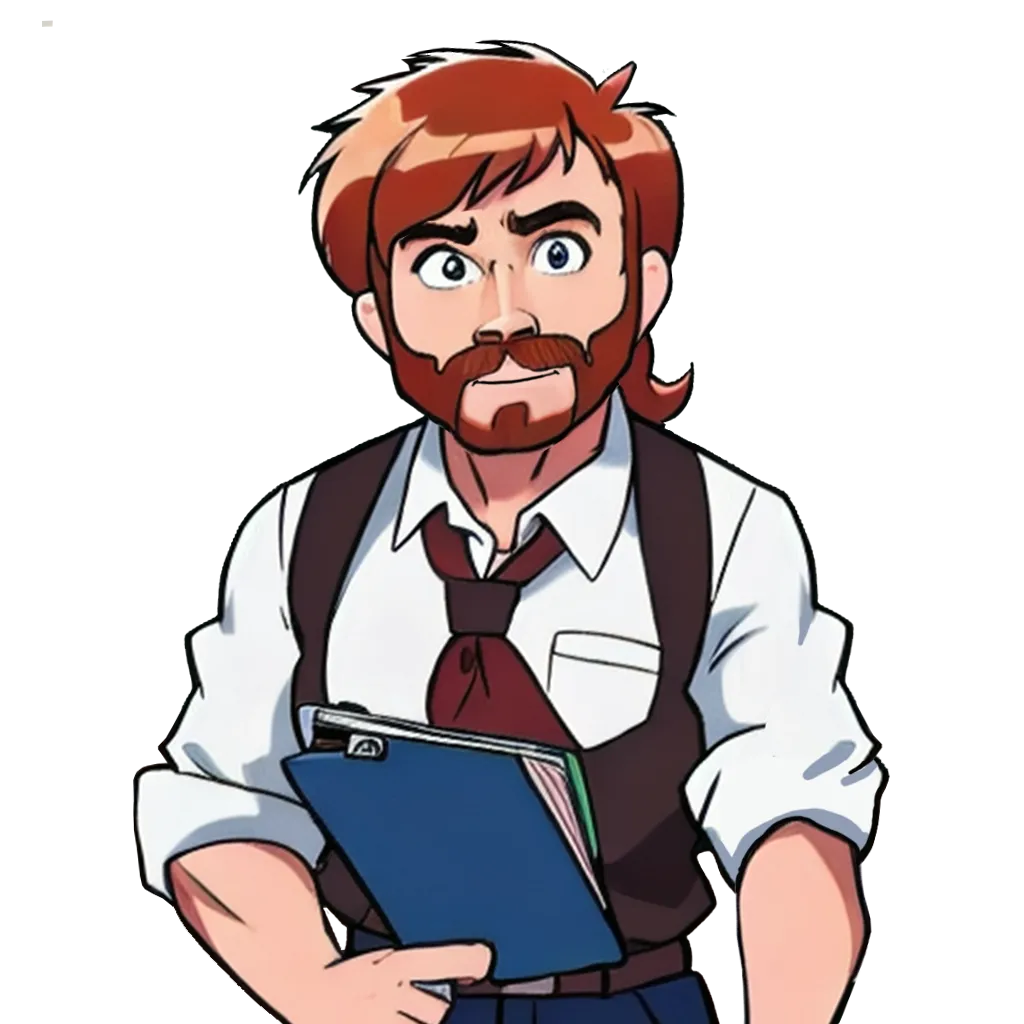