React Hooks
The useReducer Hook
useReducer Hook
Introduction
The useReducer
Hook is an alternative to useState
for managing state in functional components. It is useful for handling complex states and update logic that involves multiple sub-values or when the next state depends on the previous one. useReducer
is based on the concept of a reducer, similar to the pattern used in Redux.
Basic Syntax
useReducer
is invoked with two arguments: a reducer and an initial state. It returns an array that contains the current state and the dispatch to send actions.
javascript
Example Description
In this example:
initialState
is the initial state of the counter.reducer
is a function that determines how the state changes in response to a dispatched action.Counter
usesuseReducer
to manage the state of the counter. Clicking on the buttons sends actions that the reducer handles to update the state.
When to use useReducer
useReducer
is especially useful when:
- You have complex state logic involving multiple sub-values.
- The next state depends on the current state.
- You want to optimize the performance of components that might re-render due to state changes.
Advanced Example
Consider a more advanced example with a login form.
javascript
Advanced Example Description
In the advanced example:
initialState
contains the initial values of the form.formReducer
handles actions to update specific fields, set errors, and reset the form.
Advantages of useReducer
- Logical state and action handling: Allows for a clear and centralized layer in the state update logic.
- Readability: It is easier to read and maintain in complex states.
Limitations
Although useReducer
is powerful, it might be unnecessarily complex for simple states. Consider using useState
for straightforward cases.
[Placeholder for an image about the useReducer flow: Diagram showing how the state is managed using the useReducer
Hook, including the initial state, reducer, dispatched action, and how it affects the state.]
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
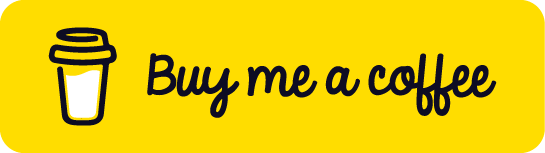
Chat with Chuck
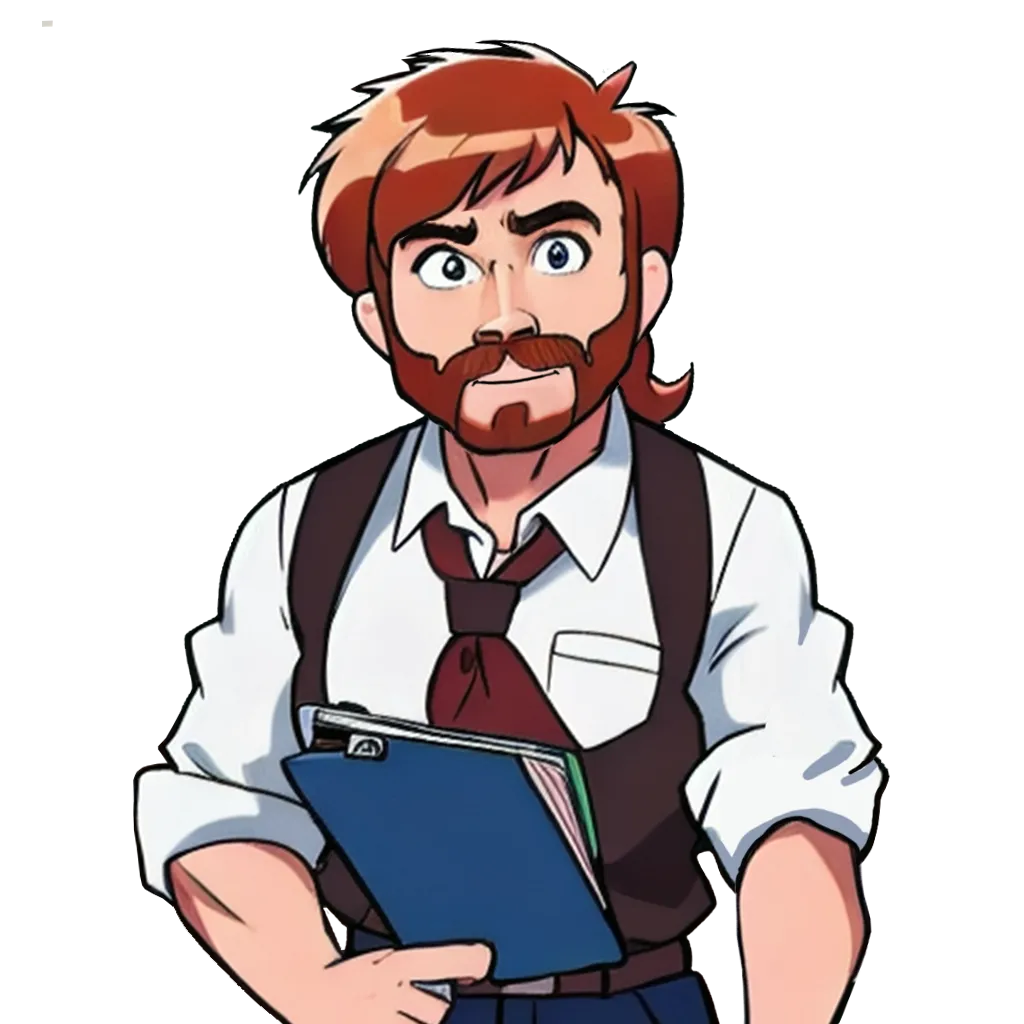