React Hooks
The useCallback Hook
useCallback Hook
Introduction
The useCallback
Hook is similar to useMemo
, but instead of memoizing a value, it memoizes a function. This Hook is useful for avoiding the re-creation of functions on every render, especially when they are passed as props to child components that depend on the function reference.
Basic Syntax
useCallback
takes a function and a dependency list, and only re-creates the function if one of the dependencies changes.
javascript
Example Description
In this example:
ParentComponent
declares a functionhandleClick
usinguseCallback
.handleClick
is created only once during the component's lifecycle because its dependency (the empty array) does not change.ChildComponent
receiveshandleClick
as a prop, and this reference will not change between renders, preventing unnecessary re-renders of the child component.
Use Cases
useCallback
is particularly useful when:
- Function props are causing unwanted re-renders: When passing a function as a prop to a child component that might re-render because the function reference has changed.
- Performance optimization: In components that depend on memoized functions to avoid additional renders or complex calculations.
Advanced Example
Consider a button that only re-renders when specific props change.
javascript
Advanced Example Description
In this example:
handleClick
is memoized usinguseCallback
and is only re-created whencount
changes.- The
Button
component only re-renders when the text or the function referencehandleClick
changes.
When Not to Use useCallback
Similar to useMemo
, do not use useCallback
excessively. Its use can add unnecessary complexity if you do not have specific performance issues to solve.
Comparison with useMemo
While useMemo
memoizes values, useCallback
memoizes functions. Both have their own use cases and benefits in optimizing an application's performance.
Warnings
As with any performance optimization, you should measure and be sure that useCallback
has a clear positive impact. Unmeasured use can lead to maintenance difficulties without real benefits.
[Placeholder for image on the use of useCallback: Diagram showing how useCallback
works to avoid function re-creation on each render, highlighting dependency on values and relationship with child component props.]
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
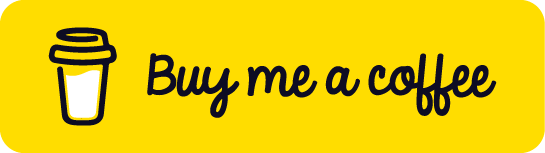
Chat with Chuck
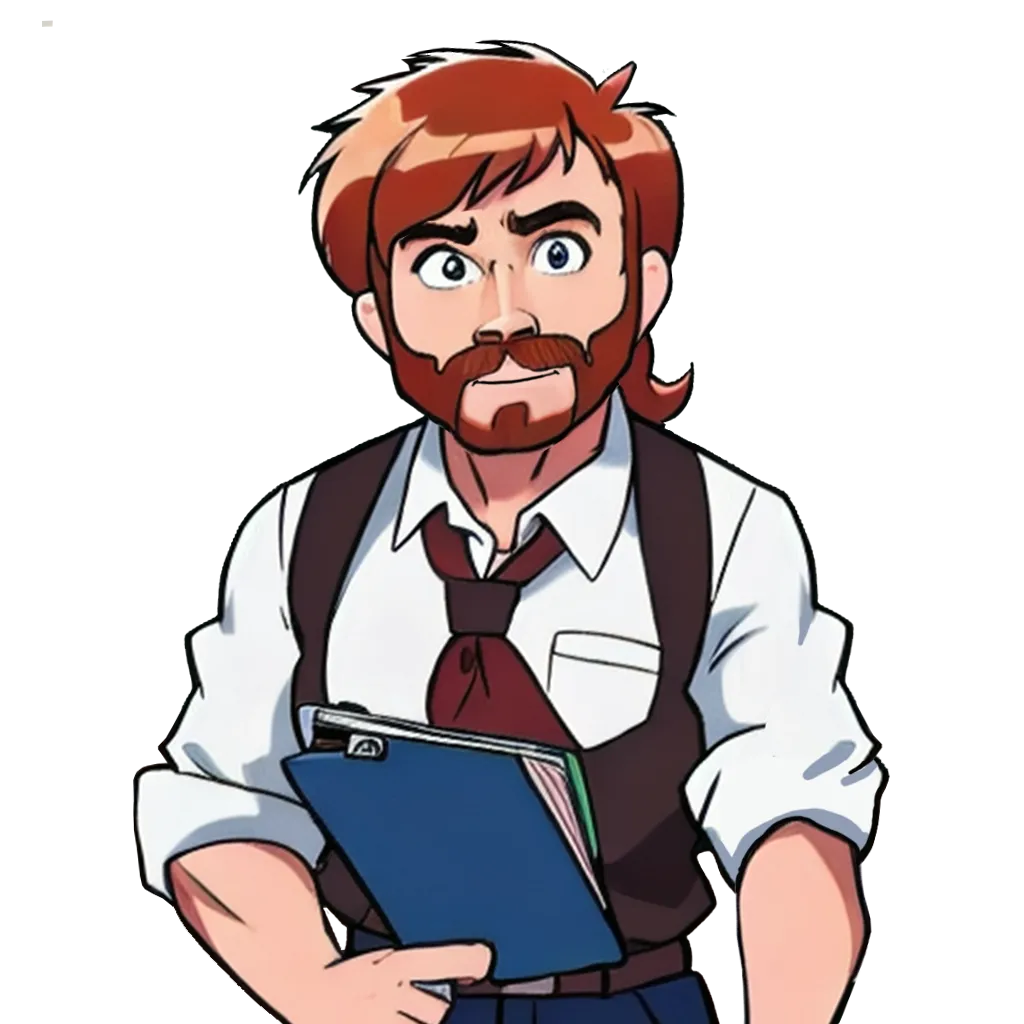