DOM Manipulation in JavaScript
Basic Concepts of the DOM
To gain a solid understanding of how to manipulate the DOM with JavaScript, we first need to familiarize ourselves with the basic concepts and structure of the DOM. These concepts are fundamental for any type of manipulation we conduct later on.
DOM Tree
The DOM represents the structure of an HTML document as a tree of nodes. Each node in the tree can be an element, an attribute, or a fragment of text. The root of the tree is the document
object, which represents the entire document.
[Placeholder: Diagram of a DOM tree with document
as the root and several child nodes representing HTML elements.]
Node Types
In the DOM, there are several types of nodes; the most common are:
- Elements: Represent HTML tags, e.g.,
<div>
,<p>
,<a>
, etc. - Attributes: Provide additional information about an element, e.g., the
src
attribute of an<img>
tag. - Text: Represents textual content within an element.
- Comments: Represent comments in HTML.
Accessing the DOM
Access to the DOM is achieved through a scripting language like JavaScript. Once we have access to the DOM, we can use various functions to select, modify, and manipulate nodes.
Element Selection
Before you can manipulate elements in the DOM, you first need to select them. Basic techniques include:
document.getElementById(id)
: Selects an element by its ID.document.getElementsByClassName(className)
: Selects all elements with the specified class.document.getElementsByTagName(tagName)
: Selects all elements with the specified tag name.document.querySelector(selector)
: Selects the first element that matches the specified CSS selector.document.querySelectorAll(selector)
: Selects all elements that match the specified CSS selector.
Example of Element Selection
Let's look at a simple example of element selection:
html
Conclusion
Understanding the basic concepts of the DOM and how to access different DOM elements is crucial for its manipulation. In the upcoming chapters, we will delve deeper into how to interact with these elements more advancedly, modifying attributes, content, and structures.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
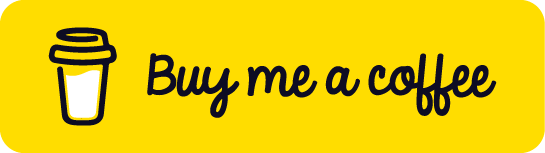
Chat with Chuck
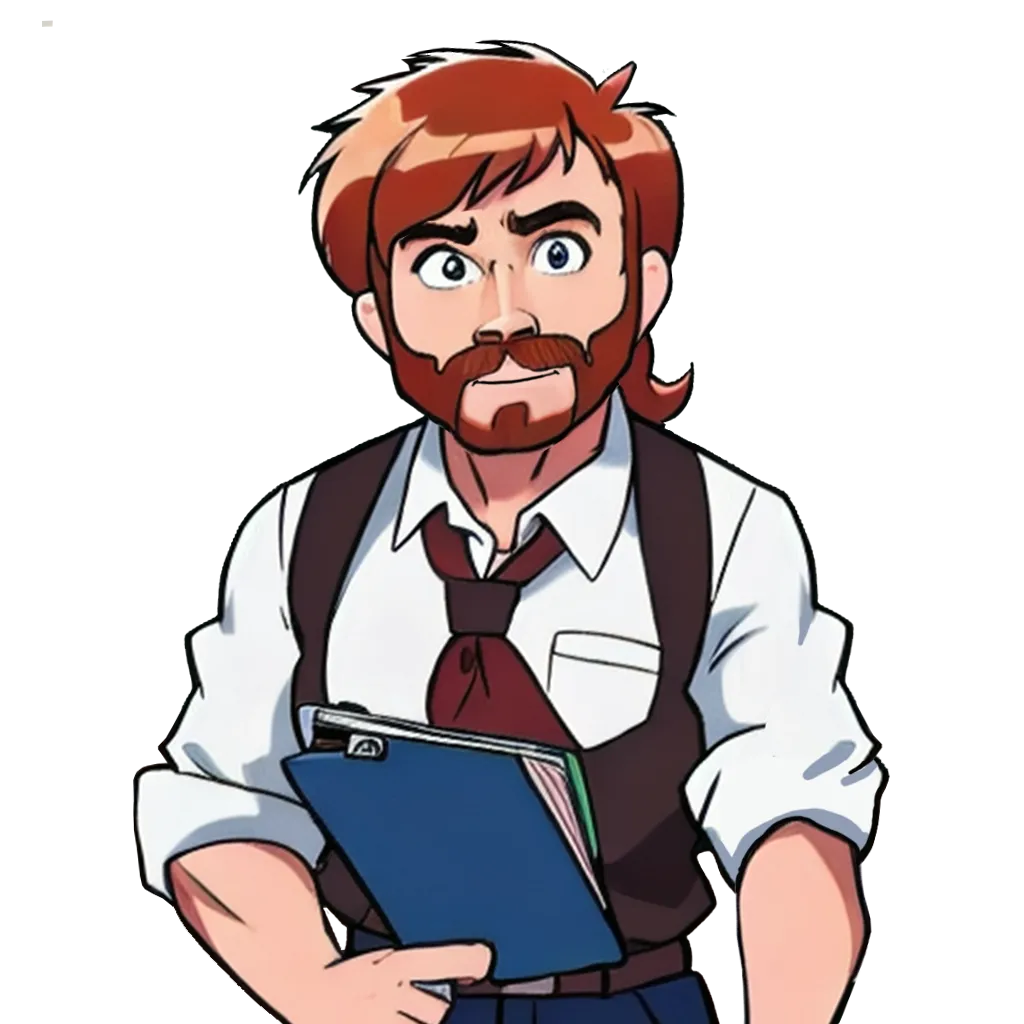
- Introduction to DOM Manipulation
- Basic Concepts of the DOM
- Selecting Elements in the DOM
- Manipulating Attributes and Properties
- Modifying Content and Structure of the DOM
- Creating and Deleting Elements
- Handling Events in the DOM
- Event Delegation
- Styles and Classes in the DOM
- Navigating the DOM
- Animations and Transitions in the DOM
- Integration of the DOM with AJAX and Fetch API
- Best Practices and Optimization for DOM Manipulation
- Tools and Libraries for DOM Manipulation
- Conclusions and Next Steps in DOM Manipulation