DOM Manipulation in JavaScript
Modifying Content and Structure of the DOM
Modifying the content and structure of the DOM is a common task when creating interactive web applications. Whether we need to change the text of an element, add new elements, or rearrange existing ones, JavaScript provides a variety of methods and techniques to do so.
Modifying Content
To change the content of a DOM element, we can use the properties innerText
, innerHTML
, and textContent
.
innerText
Modifies the visible text within an element. Only includes the text that is rendered on the screen.
javascript
innerHTML
Allows modification of the HTML content inside an element. This method can insert dynamic HTML content.
javascript
textContent
Similar to innerText
, but includes all the text in the node tree, including text hidden by CSS.
javascript
[Placeholder: Image of an HTML element before and after modifying its content with innerText
and innerHTML
.]
Inserting Elements
We can insert new elements into the DOM using methods like appendChild
, insertBefore
, and innerHTML
.
appendChild
Adds a new node as the last child of an element.
javascript
insertBefore
Adds a new node before an existing node.
javascript
Removing Elements
We can remove elements from the DOM using methods like removeChild
and remove
.
removeChild
Removes a specific child from an element.
javascript
remove
Removes the element itself from the DOM.
javascript
Replacing Elements
We can replace an existing element with a new one using replaceChild
.
javascript
Cloning Elements
We can clone an existing node and its descendants using cloneNode
.
javascript
Complete Example
The following example shows how to insert, delete, and modify elements in the DOM:
html
Conclusion
The ability to modify the content and structure of the DOM allows us to create dynamic and interactive web applications. In the upcoming chapters, we will explore how to handle events and make interactions even more dynamic and responsive.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
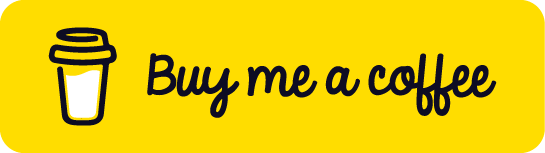
Chat with Chuck
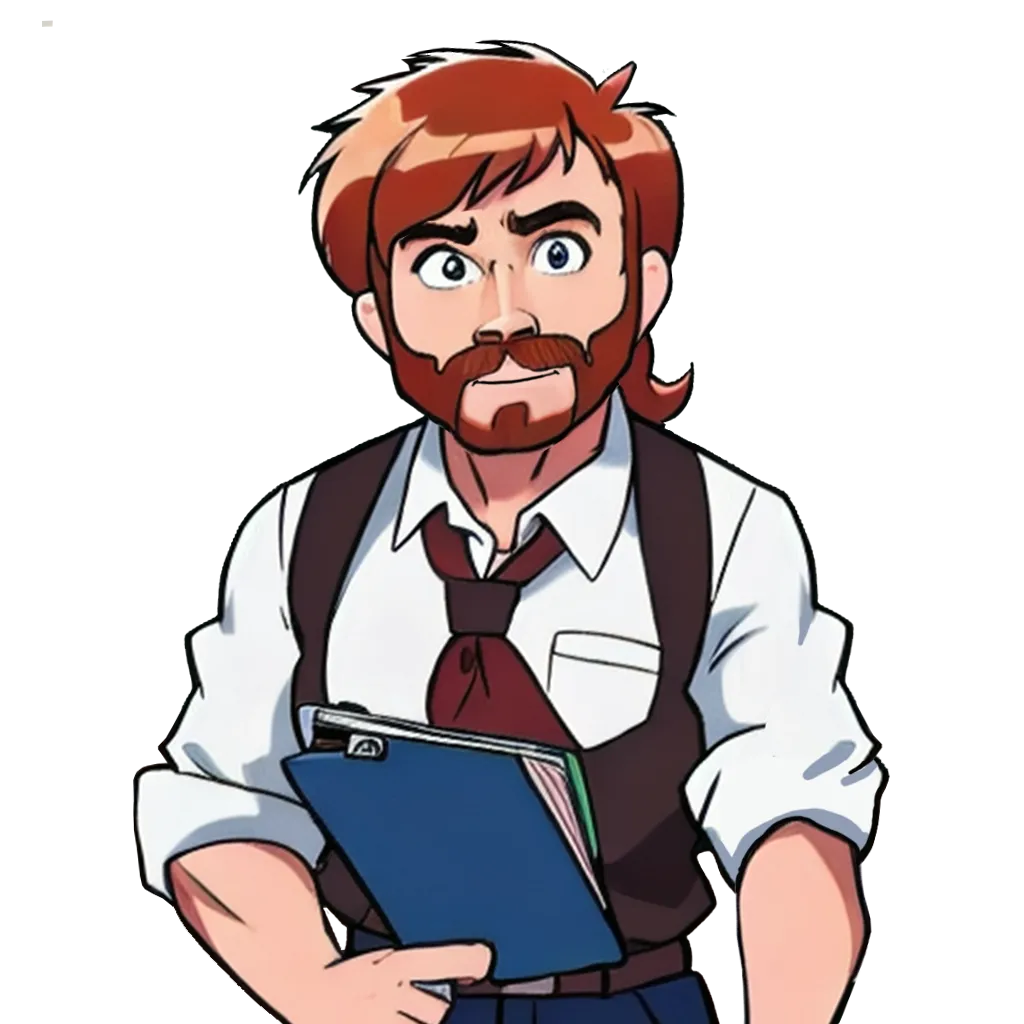
- Introduction to DOM Manipulation
- Basic Concepts of the DOM
- Selecting Elements in the DOM
- Manipulating Attributes and Properties
- Modifying Content and Structure of the DOM
- Creating and Deleting Elements
- Handling Events in the DOM
- Event Delegation
- Styles and Classes in the DOM
- Navigating the DOM
- Animations and Transitions in the DOM
- Integration of the DOM with AJAX and Fetch API
- Best Practices and Optimization for DOM Manipulation
- Tools and Libraries for DOM Manipulation
- Conclusions and Next Steps in DOM Manipulation