DOM Manipulation in JavaScript
Navigating the DOM
Navigating the DOM is essential for accessing specific elements and manipulating their content, styles, or properties. The DOM is a hierarchical structure of nodes, and JavaScript provides us with various properties and methods to traverse this structure and locate elements.
Navigation Properties
Below are some of the most common navigation properties available on DOM nodes:
parentNode
Accesses the parent node of the current element.
javascript
childNodes
and children
childNodes
returns a collection of all child nodes, including text, comments, and elements. children
returns only the child elements.
javascript
firstChild
and firstElementChild
firstChild
returns the first child node, while firstElementChild
returns the first child element.
javascript
lastChild
and lastElementChild
Similar to firstChild
, but returns the last child node or the last child element.
javascript
nextSibling
and nextElementSibling
nextSibling
returns the next sibling node at the same level, while nextElementSibling
returns the next sibling element.
javascript
previousSibling
and previousElementSibling
Similar to nextSibling
, but returns the previous node or previous element.
javascript
DOM Navigation Example
Below is an example demonstrating how to navigate through various levels of the DOM:
html
[Placeholder: Image showing a diagram of the DOM with the elements parentElement
, firstChild
, secondChild
, and thirdChild
, and how to navigate between them.]
Navigation with Query Selectors
Besides navigation properties, we can use querySelector
and querySelectorAll
to select nodes based on CSS selectors.
javascript
Using closest
The closest
method allows finding the nearest ancestor that matches a given selector.
javascript
Complete Navigation Example
In this complete example, we'll combine various navigation techniques to manipulate elements in a more complex DOM tree:
html
Conclusion
Navigating the DOM is a fundamental skill for any web developer. By mastering the various navigation properties and methods, we can access and manipulate any part of the document efficiently. In the next chapter, we'll delve into animations and transitions in the DOM to enhance the user experience.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
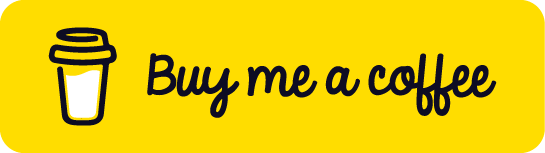
Chat with Chuck
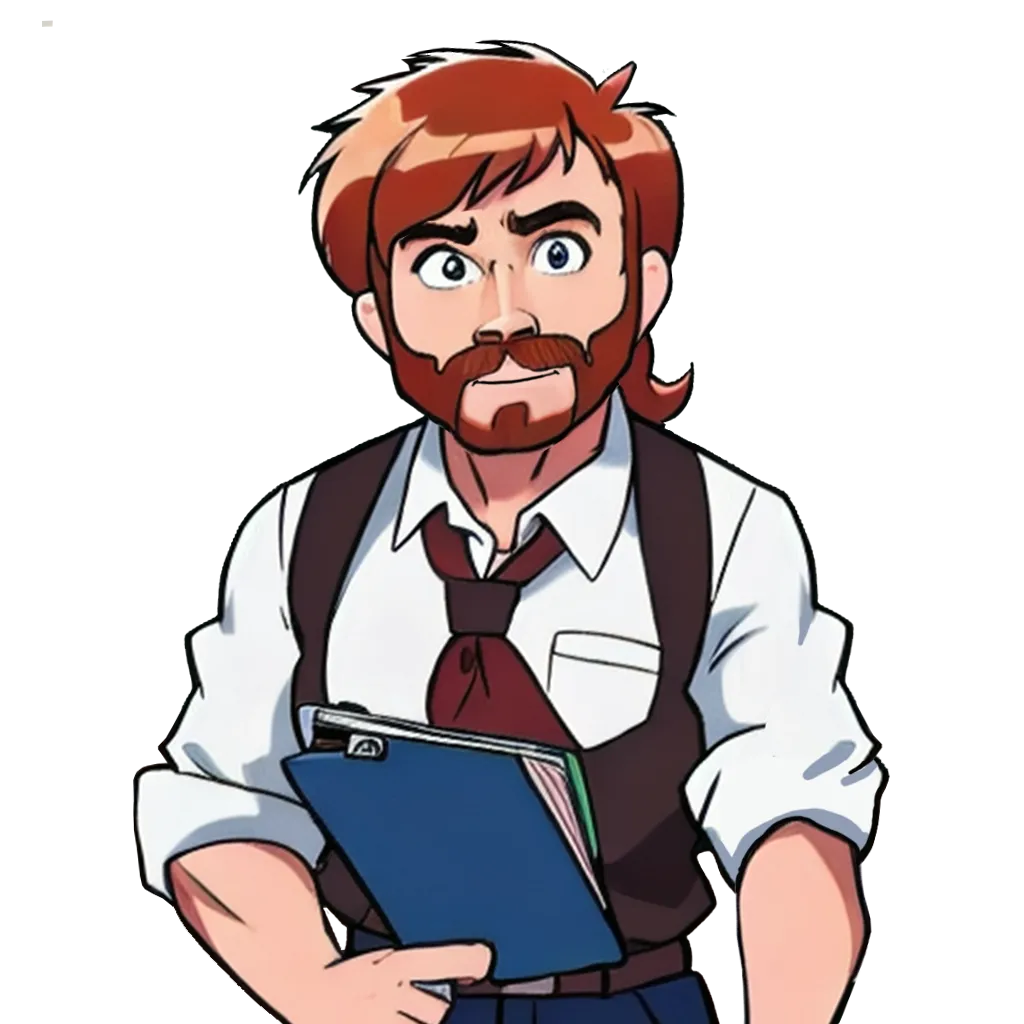
- Introduction to DOM Manipulation
- Basic Concepts of the DOM
- Selecting Elements in the DOM
- Manipulating Attributes and Properties
- Modifying Content and Structure of the DOM
- Creating and Deleting Elements
- Handling Events in the DOM
- Event Delegation
- Styles and Classes in the DOM
- Navigating the DOM
- Animations and Transitions in the DOM
- Integration of the DOM with AJAX and Fetch API
- Best Practices and Optimization for DOM Manipulation
- Tools and Libraries for DOM Manipulation
- Conclusions and Next Steps in DOM Manipulation