DOM Manipulation in JavaScript
Event Delegation
Event delegation is an advanced technique in DOM manipulation that allows us to manage events more efficiently. Instead of attaching an event listener to each element that might generate an event, a single listener is attached to a common ancestor. This single listener can then handle events triggered by child elements.
Why Use Event Delegation?
Event delegation is especially useful when working with dynamically generated elements or when reducing the number of event listeners on a page. Some advantages include:
- Improved performance: Reducing the number of event listeners that need to be attached to elements.
- Simplicity in management: Facilitating event management, especially when elements are dynamically added or removed.
How Event Delegation Works
Event delegation leverages the event propagation technique in the DOM, especially the bubbling phase. During this phase, an event propagates from the element that triggered it upwards through its ancestors in the DOM tree.
Basic Example of Event Delegation
Suppose we have a list of items and want to handle clicks on any item in the list using a single event listener.
html
In this example:
- We attach a single event listener to the
ul
(parent element). - When a
li
(child element) is clicked, the event bubbles up to theul
. - We use
event.target
to check if the clicked element is ali
.
Using matches
and closest
To work with event delegation, two useful methods are matches
and closest
.
matches(selector)
: Checks if the calling element matches the specified selector.closest(selector)
: Returns the closest ancestor that matches the selector, or the element itself if it matches.
Example using closest
html
Event Delegation and Dynamic Elements
Event delegation is very useful when working with dynamically created elements, as the listener attached to the parent element can handle events from new elements without the need to add new listeners.
Example with dynamic elements
html
In this example, even though new items are dynamically added to the list, the single event listener on the parent ul
can handle clicks on all items, including the new ones.
Conclusion
Event delegation is a powerful and efficient technique for handling events in web applications, especially when working with dynamic components or seeking to optimize performance. In the next chapters, we will explore topics such as manipulating styles and classes in the DOM and how to navigate through different DOM nodes.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
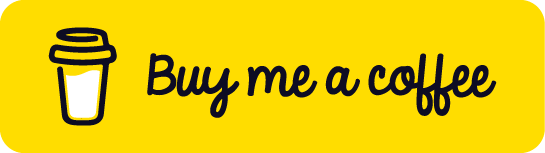
Chat with Chuck
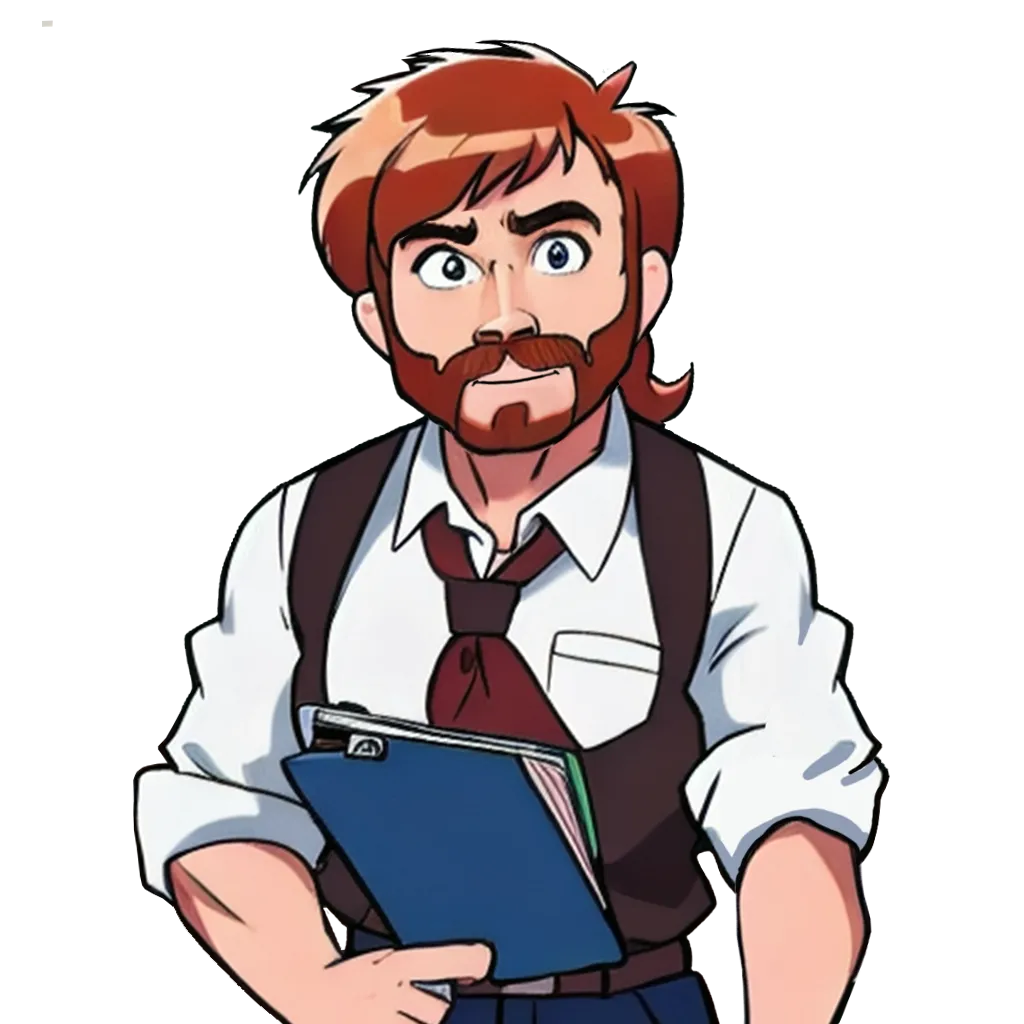
- Introduction to DOM Manipulation
- Basic Concepts of the DOM
- Selecting Elements in the DOM
- Manipulating Attributes and Properties
- Modifying Content and Structure of the DOM
- Creating and Deleting Elements
- Handling Events in the DOM
- Event Delegation
- Styles and Classes in the DOM
- Navigating the DOM
- Animations and Transitions in the DOM
- Integration of the DOM with AJAX and Fetch API
- Best Practices and Optimization for DOM Manipulation
- Tools and Libraries for DOM Manipulation
- Conclusions and Next Steps in DOM Manipulation