DOM Manipulation in JavaScript
Creating and Deleting Elements
Creating and deleting elements in the DOM is an essential operation for any dynamic web application. This capability allows developers to add new content and components to their web pages dynamically, as well as remove elements that are no longer needed.
Creating Elements
To create a new element in the DOM, we use the document.createElement
method.
document.createElement
This method creates a new element of the specified type.
javascript
Inserting Elements into the DOM
Once we have created a new element, we need to insert it into the DOM. We can use several methods for this:
appendChild
insertBefore
insertAdjacentElement
,insertAdjacentHTML
, andinsertAdjacentText
appendChild
Adds a new node as the last child of an element.
javascript
insertBefore
Adds a new node before an existing node.
javascript
insertAdjacentElement
, insertAdjacentHTML
, and insertAdjacentText
These methods allow us to insert new nodes at specific positions in relation to a reference element.
javascript
[Placeholder: Image showing the difference between appendChild
, insertBefore
, and insertAdjacentElement
with graphical examples.]
Deleting Elements
We can delete elements from the DOM using removeChild
or remove
.
removeChild
This method removes a specific child from an element.
javascript
remove
This method removes the element itself from the DOM.
javascript
Complete Example
Below is a complete example that creates, inserts, and deletes elements in the DOM:
html
Conclusion
The ability to create and delete elements in the DOM is crucial for building dynamic and responsive web applications. It allows us to efficiently add new content and remove unnecessary components in real-time. In the upcoming chapters, we will explore how to handle events in the DOM to make our applications even more interactive.
- Introduction to DOM Manipulation
- Basic Concepts of the DOM
- Selecting Elements in the DOM
- Manipulating Attributes and Properties
- Modifying Content and Structure of the DOM
- Creating and Deleting Elements
- Handling Events in the DOM
- Event Delegation
- Styles and Classes in the DOM
- Navigating the DOM
- Animations and Transitions in the DOM
- Integration of the DOM with AJAX and Fetch API
- Best Practices and Optimization for DOM Manipulation
- Tools and Libraries for DOM Manipulation
- Conclusions and Next Steps in DOM Manipulation
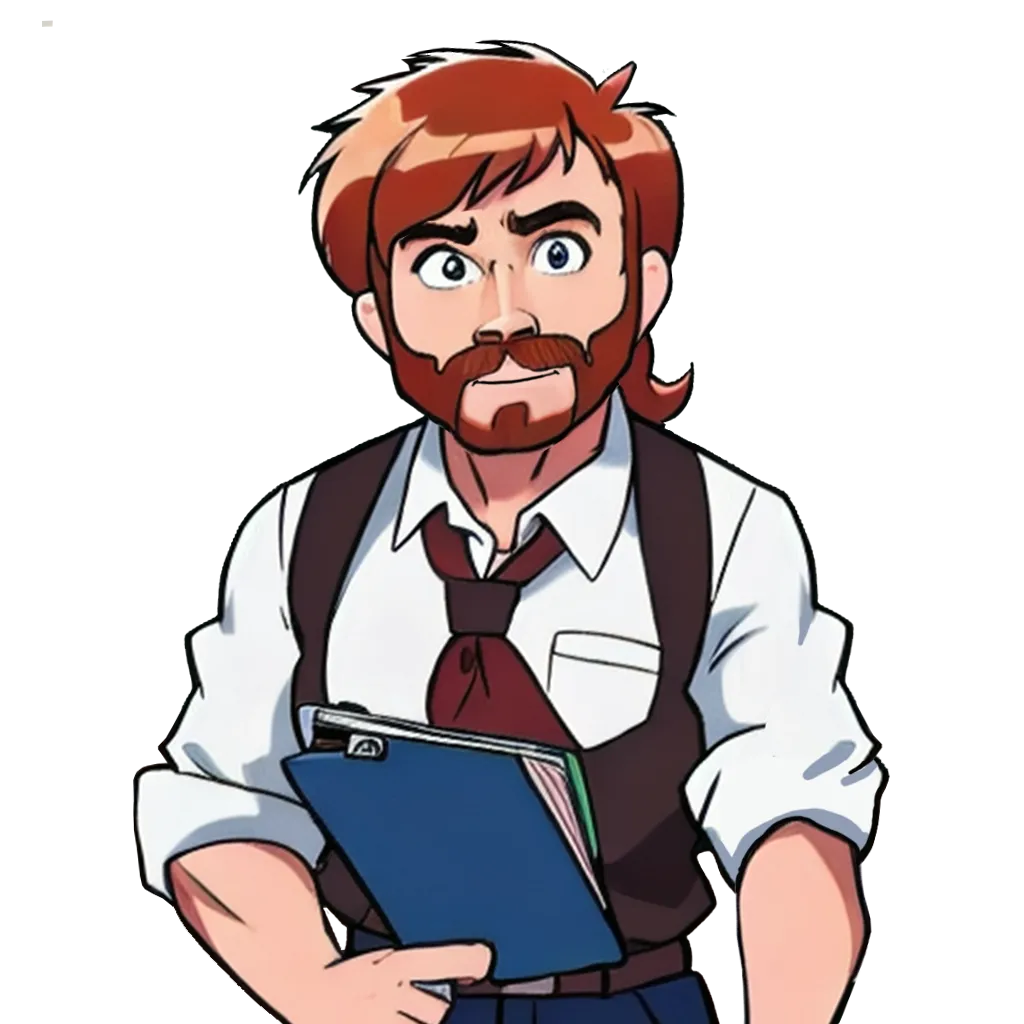