DOM Manipulation in JavaScript
Manipulating Attributes and Properties
Once we have selected the DOM elements, the next step is to learn how to manipulate their attributes and properties. This skill allows us to modify the appearance and behavior of HTML elements dynamically.
Difference between Attributes and Properties
- Attributes: These are part of the HTML tag and are defined directly in the HTML code. Examples include
src
,alt
,class
,id
, etc. - Properties: These are representations in the DOM of the attributes, but they can have different values than those of the attributes in the HTML code. Properties also include characteristics not represented in HTML, such as
innerText
orvalue
.
Manipulating Attributes
We can use the methods getAttribute
, setAttribute
, and removeAttribute
to work with attributes.
getAttribute
This method retrieves the value of a specific attribute.
javascript
setAttribute
This method sets the value of an attribute.
javascript
removeAttribute
This method removes an attribute.
javascript
Manipulating Properties
Unlike attributes, properties are manipulated directly as JavaScript objects.
javascript
Manipulating Classes and Styles
Classes and styles can be considered both attributes and properties, but they are typically manipulated in specific ways.
Manipulating Classes
We can add, remove, or check if an element has a class using the classList
properties.
javascript
Manipulating Styles
Inline styles can be manipulated directly using the style
property.
javascript
Complete Example
Here is an example that combines various techniques for manipulating attributes and properties:
html
Conclusion
Manipulating DOM attributes and properties is a powerful tool that allows us to dynamically modify the content and appearance of our HTML elements. In the next chapter, we will explore how to modify the content and structure of the DOM in more detail.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
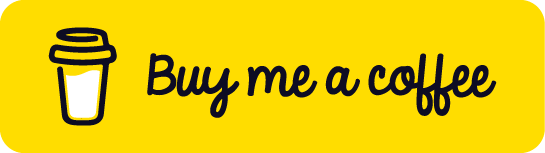
Chat with Chuck
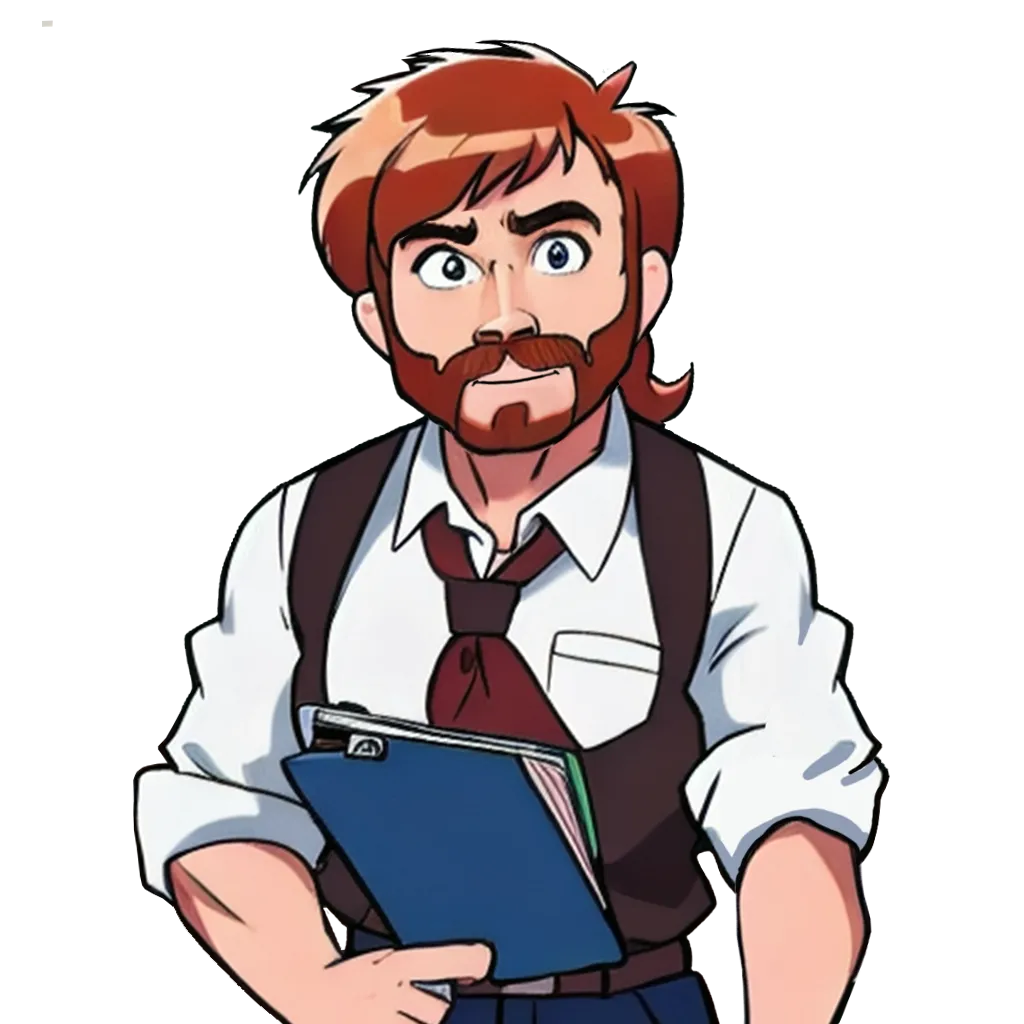
- Introduction to DOM Manipulation
- Basic Concepts of the DOM
- Selecting Elements in the DOM
- Manipulating Attributes and Properties
- Modifying Content and Structure of the DOM
- Creating and Deleting Elements
- Handling Events in the DOM
- Event Delegation
- Styles and Classes in the DOM
- Navigating the DOM
- Animations and Transitions in the DOM
- Integration of the DOM with AJAX and Fetch API
- Best Practices and Optimization for DOM Manipulation
- Tools and Libraries for DOM Manipulation
- Conclusions and Next Steps in DOM Manipulation