DOM Manipulation in JavaScript
Handling Events in the DOM
Event handling is a fundamental part of interactivity in web applications. Events allow users to interact with the web page in various ways, such as clicks, key presses, mouse movements, among others. In this chapter, we will learn how to listen and respond to events in the DOM using JavaScript.
What is an Event?
An event is anything that happens in the browser that can be detected by JavaScript. This includes user actions, like clicks and key presses, and also browser events, like page load.
Event Listening
To handle events, we first need to listen to those events on DOM elements. There are several ways to add these "event listeners":
addEventListener
The addEventListener
method allows you to add an event handler to an element. This is the most recommended method as it offers greater flexibility.
javascript
Direct Assignment
Another way to add an event handler is by assigning a function directly to the element's event property.
javascript
Removing Event Handlers
To remove an event handler, use removeEventListener
.
javascript
Examples of Common Events
Below are some examples of common events and how to handle them:
Click Event
javascript
Key Press Event
javascript
Mouse Move Event
javascript
Form Events
Form events are crucial for validating and processing user input.
Form Submit Event
javascript
Event Delegation
Event delegation is a technique where a single event listener is used to handle events on multiple child elements. This is especially useful for handling events on dynamically created elements.
Event Delegation Example
javascript
Complete Example
The following example covers several common events and how to handle them:
html
Conclusion
Handling events in the DOM allows us to create interactive and responsive web applications. By understanding how to listen to and manipulate events, we can improve user experience and create more dynamic applications. In the next chapter, we will explore event delegation for even more efficient event management in the DOM.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
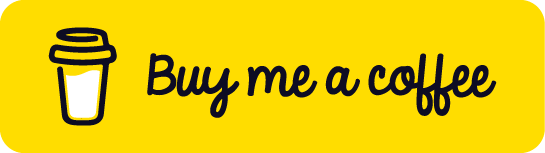
Chat with Chuck
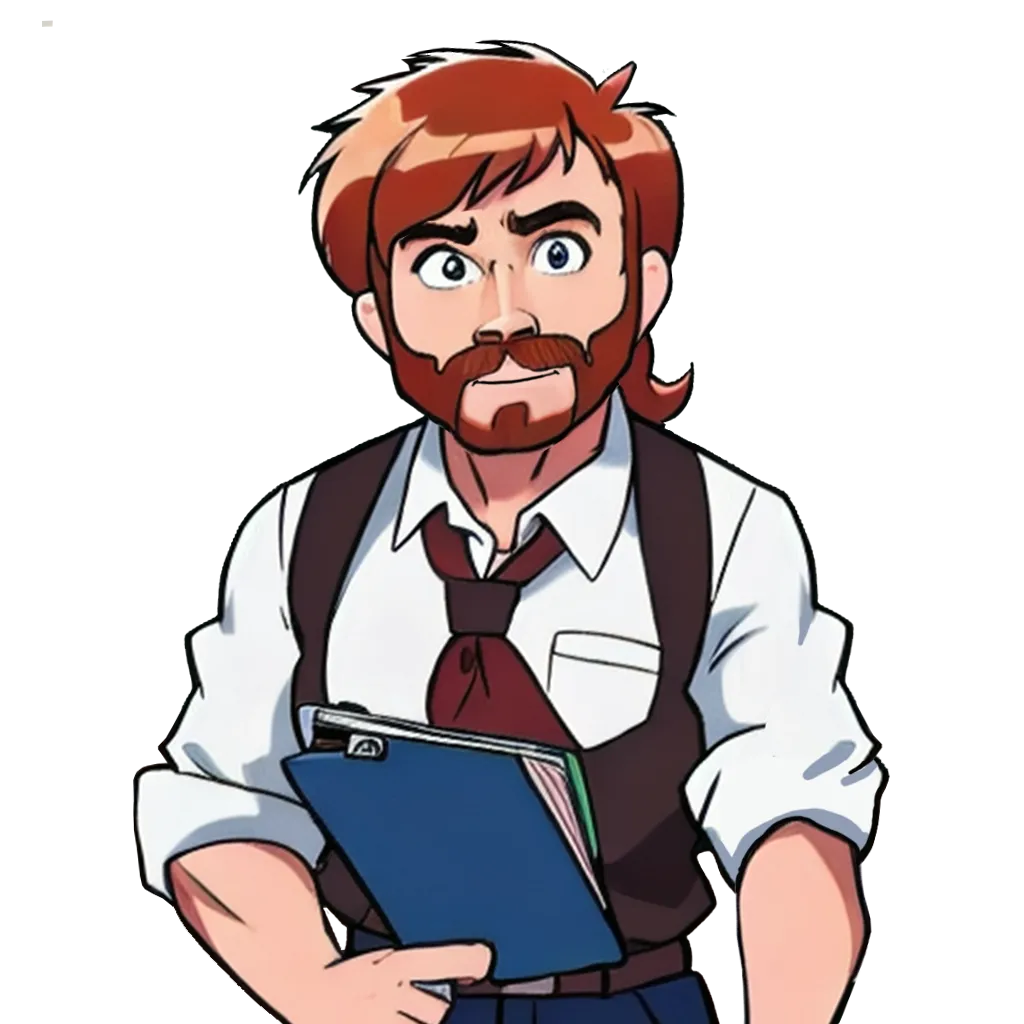
- Introduction to DOM Manipulation
- Basic Concepts of the DOM
- Selecting Elements in the DOM
- Manipulating Attributes and Properties
- Modifying Content and Structure of the DOM
- Creating and Deleting Elements
- Handling Events in the DOM
- Event Delegation
- Styles and Classes in the DOM
- Navigating the DOM
- Animations and Transitions in the DOM
- Integration of the DOM with AJAX and Fetch API
- Best Practices and Optimization for DOM Manipulation
- Tools and Libraries for DOM Manipulation
- Conclusions and Next Steps in DOM Manipulation