DOM Manipulation in JavaScript
Best Practices and Optimization for DOM Manipulation
DOM manipulation can significantly impact the performance of a web page. If not handled properly, it can lead to slowdowns, UI blocking, and a negative user experience. In this chapter, we will review some best practices and optimization techniques to improve efficiency and performance when manipulating the DOM.
Minimizing Reflows and Repaints
Every time you modify the DOM structure or the layout of an element, the browser performs a reflow (layout recalculation) and repaint (screen redraw). These processes can be costly in terms of performance.
Best Practices:
-
Avoid Multiple Changes: Try to make multiple changes at once instead of changing them one by one.
javascript -
Document Fragments: Use DocumentFragments to make multiple additions or changes to the DOM at once.
javascript -
Node Cloning: Clone a node, modify it, and then replace the original.
javascript
Limiting DOM Accesses
Accessing the DOM is an expensive operation. Try to minimize the number of accesses by making all possible modifications in a single operation.
Caching Elements
Store references to DOM elements in variables to avoid repeated searches.
javascript
Efficient Use of Selectors
Use efficient and specific selectors to minimize search time.
Using IDs and Specific Classes
javascript
Avoid Direct Layout Manipulations
Some properties like offset
, scroll
, and client
can force a reflow. Try to avoid using them repeatedly.
Example of Poor Use
javascript
Using Variables
javascript
Decoupling the DOM
Separate business logic from DOM manipulation and use frameworks or libraries that optimize these tasks, such as React, Vue, or Angular.
Use of requestAnimationFrame
For animations, use requestAnimationFrame
instead of setTimeout
or setInterval
. requestAnimationFrame
optimizes animation by synchronizing with the browser's refresh rate.
Example of requestAnimationFrame
javascript
Debouncing and Throttling
To handle events that fire frequently, like scroll
or resize
, use debouncing and throttling techniques.
Example of Debouncing
javascript
Example of Throttling
javascript
Conclusion
Applying best practices and optimization techniques when manipulating the DOM is crucial to maintaining high performance and offering a smooth user experience. From minimizing reflows and repaints to using advanced techniques like requestAnimationFrame
, debouncing, and throttling, these strategies help us improve the efficiency of our web applications. In the next chapter, we will explore various tools and libraries that can facilitate DOM manipulation.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
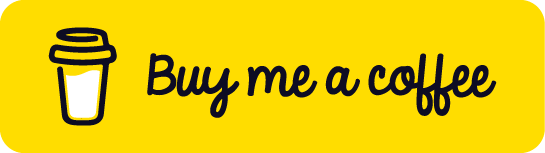
Chat with Chuck
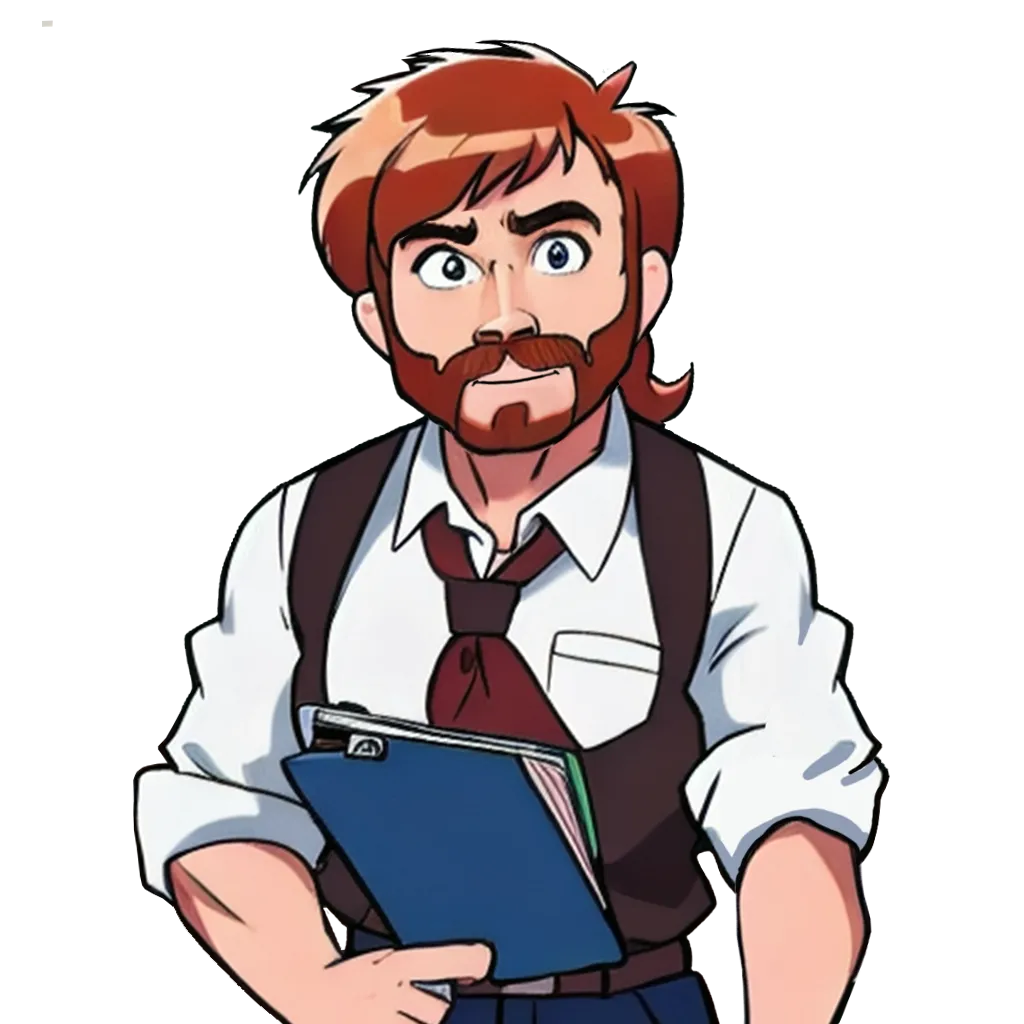
- Introduction to DOM Manipulation
- Basic Concepts of the DOM
- Selecting Elements in the DOM
- Manipulating Attributes and Properties
- Modifying Content and Structure of the DOM
- Creating and Deleting Elements
- Handling Events in the DOM
- Event Delegation
- Styles and Classes in the DOM
- Navigating the DOM
- Animations and Transitions in the DOM
- Integration of the DOM with AJAX and Fetch API
- Best Practices and Optimization for DOM Manipulation
- Tools and Libraries for DOM Manipulation
- Conclusions and Next Steps in DOM Manipulation