DOM Manipulation in JavaScript
Integration of the DOM with AJAX and Fetch API
The integration of the DOM with AJAX and the Fetch API allows for the construction of dynamic and responsive web applications. AJAX (Asynchronous JavaScript and XML) and the Fetch API are techniques that enable us to send and receive data from a server in the background, making it possible to update parts of the webpage without needing to reload it completely.
What is AJAX?
AJAX is a technique for creating interactive web applications that communicate with the server asynchronously and update the user interface without reloading the entire page. Although its name indicates XML, it can be used with a variety of formats such as JSON and HTML.
XMLHttpRequest
The XMLHttpRequest
interface is a traditional way to make AJAX requests in JavaScript.
Example of XMLHttpRequest
javascript
Fetch API
The Fetch API is a modern way to make asynchronous requests and promises several benefits over XMLHttpRequest
, such as better promise handling and cleaner code.
Basic Fetch API Example
javascript
POST Requests with Fetch API
We can use the Fetch API to send data to the server through POST requests.
Example of POST Request
javascript
Dynamic DOM Update with Fetch API
Below is a complete example of how to use the Fetch API to fetch data and dynamically update the DOM:
html
Error Handling
It is crucial to handle errors in AJAX and Fetch API requests to provide a good user experience.
Example of Error Handling with Fetch API
javascript
Using Async/Await with Fetch API
The use of async
and await
can further simplify the code that handles asynchronous requests.
Example of Async/Await with Fetch API
javascript
Conclusion
The integration of the DOM with AJAX and the Fetch API enables the creation of interactive and dynamic web applications. By understanding how to make asynchronous requests and update the DOM, we can significantly improve the user experience by reducing the need for complete page reloads. In the next chapter, we will explore best practices and optimization for DOM manipulation to ensure optimal performance.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
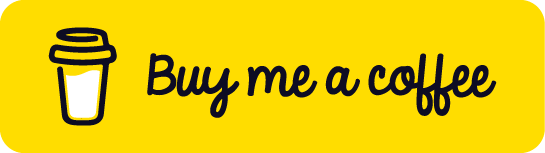
Chat with Chuck
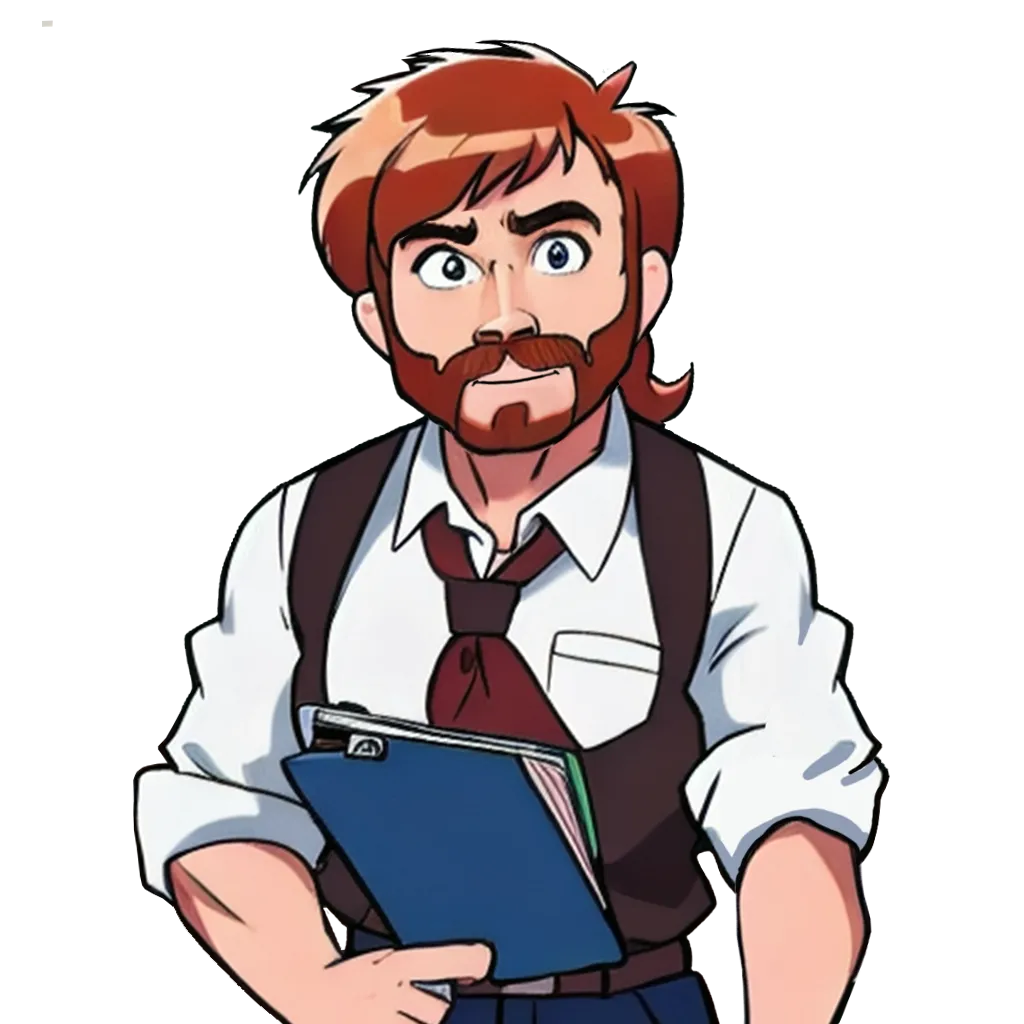
- Introduction to DOM Manipulation
- Basic Concepts of the DOM
- Selecting Elements in the DOM
- Manipulating Attributes and Properties
- Modifying Content and Structure of the DOM
- Creating and Deleting Elements
- Handling Events in the DOM
- Event Delegation
- Styles and Classes in the DOM
- Navigating the DOM
- Animations and Transitions in the DOM
- Integration of the DOM with AJAX and Fetch API
- Best Practices and Optimization for DOM Manipulation
- Tools and Libraries for DOM Manipulation
- Conclusions and Next Steps in DOM Manipulation