DOM Manipulation in JavaScript
Selecting Elements in the DOM
Selecting elements in the DOM is a fundamental skill for any web developer. It allows us to locate and work with specific elements within the HTML document. In this chapter, we will explore different methods for selecting elements in the DOM using JavaScript.
Selecting Elements by ID
The simplest and most direct method to select an element is to use its ID. IDs must be unique within an HTML document.
javascript
Selecting Elements by Class
We can select all elements that have a specific class using getElementsByClassName
.
javascript
Selecting Elements by Tag
To select all elements of a specific tag type, we use getElementsByTagName
.
javascript
Selecting Elements with CSS Selectors
The querySelector
and querySelectorAll
methods allow us to select elements using CSS selectors. This gives us great flexibility.
document.querySelector(selector)
: Selects the first element that matches the CSS selector.document.querySelectorAll(selector)
: Selects all elements that match the CSS selector.
Example of querySelector
and querySelectorAll
javascript
Differences between HTMLCollection and NodeList
- HTMLCollection: It is a live collection of document elements. If the DOM changes, the collection updates automatically.
- NodeList: It can be static or live, depending on the method that generated it. NodeLists returned by
querySelectorAll
are static, meaning they do not change if the document is updated.
Iterating through Collections of Elements
We can iterate through both HTMLCollection
and NodeList
using loops.
javascript
Conclusion
The ability to efficiently select elements is crucial for DOM manipulation. By using different selection methods, we can easily access any part of our document. In the upcoming chapters, we will start manipulating these elements by modifying their attributes, content, and structure.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
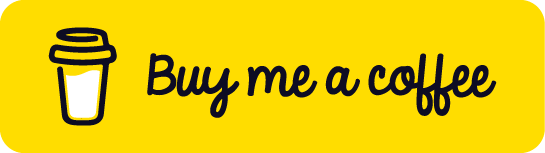
Chat with Chuck
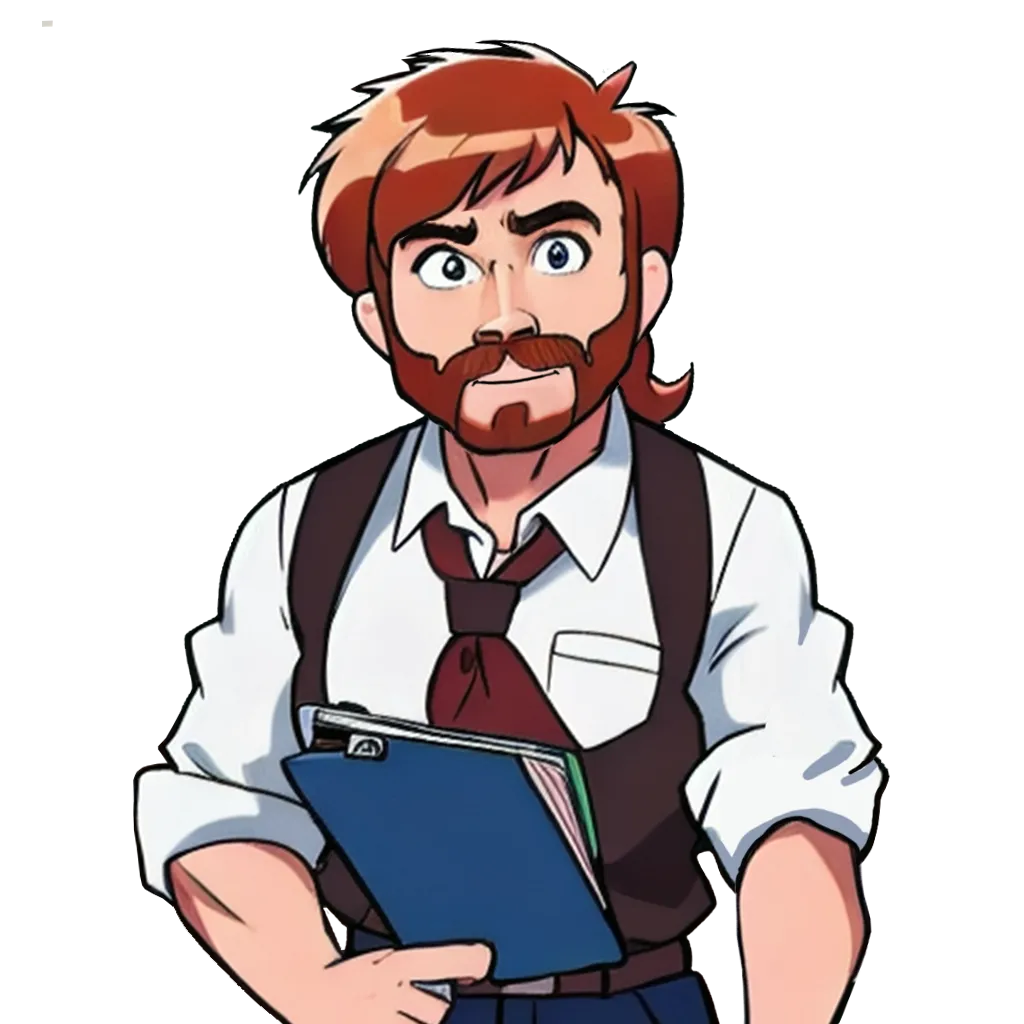
- Introduction to DOM Manipulation
- Basic Concepts of the DOM
- Selecting Elements in the DOM
- Manipulating Attributes and Properties
- Modifying Content and Structure of the DOM
- Creating and Deleting Elements
- Handling Events in the DOM
- Event Delegation
- Styles and Classes in the DOM
- Navigating the DOM
- Animations and Transitions in the DOM
- Integration of the DOM with AJAX and Fetch API
- Best Practices and Optimization for DOM Manipulation
- Tools and Libraries for DOM Manipulation
- Conclusions and Next Steps in DOM Manipulation