DOM Manipulation in JavaScript
Styles and Classes in the DOM
The manipulation of styles and classes in the DOM is essential for changing the appearance of elements on a web page in response to user interactions or data changes. With JavaScript, it is possible to add, remove, or modify inline styles and classes of elements dynamically.
Inline Styles Manipulation
Each DOM element has a style
property that allows us to set CSS styles directly on the element.
Setting Styles
We can set styles using the style
property and access the corresponding CSS properties.
javascript
Getting Computed Styles
To get the computed styles of an element (those that are actually applied after processing all CSS rules, including inheritance and browser rules), we use getComputedStyle
.
javascript
Class Manipulation
Handling classes is done through the classList
property. This property provides convenient methods to add, remove, toggle, and check classes on an element.
add
Adds one or more classes to the element.
javascript
remove
Removes one or more classes from the element.
javascript
toggle
Toggles a class on the element. If the class is present, it removes it; if not, it adds it.
javascript
contains
Checks if the element has a specific class.
javascript
replace
Replaces one class with another.
javascript
Example of Class and Style Manipulation
Below is an example that combines inline style manipulation and class manipulation using JavaScript:
html
Dynamic Example with Events
The manipulation of styles and classes is especially useful along with DOM events. Let's see an example where the appearance of a button changes when clicked:
html
Conclusion
The manipulation of styles and classes in the DOM allows us to create dynamic and responsive user interfaces. By combining these techniques with event handling, we can create rich, interactive experiences for users. In the next chapter, we will explore how to navigate through the different nodes of the DOM.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
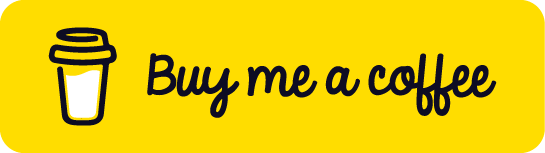
Chat with Chuck
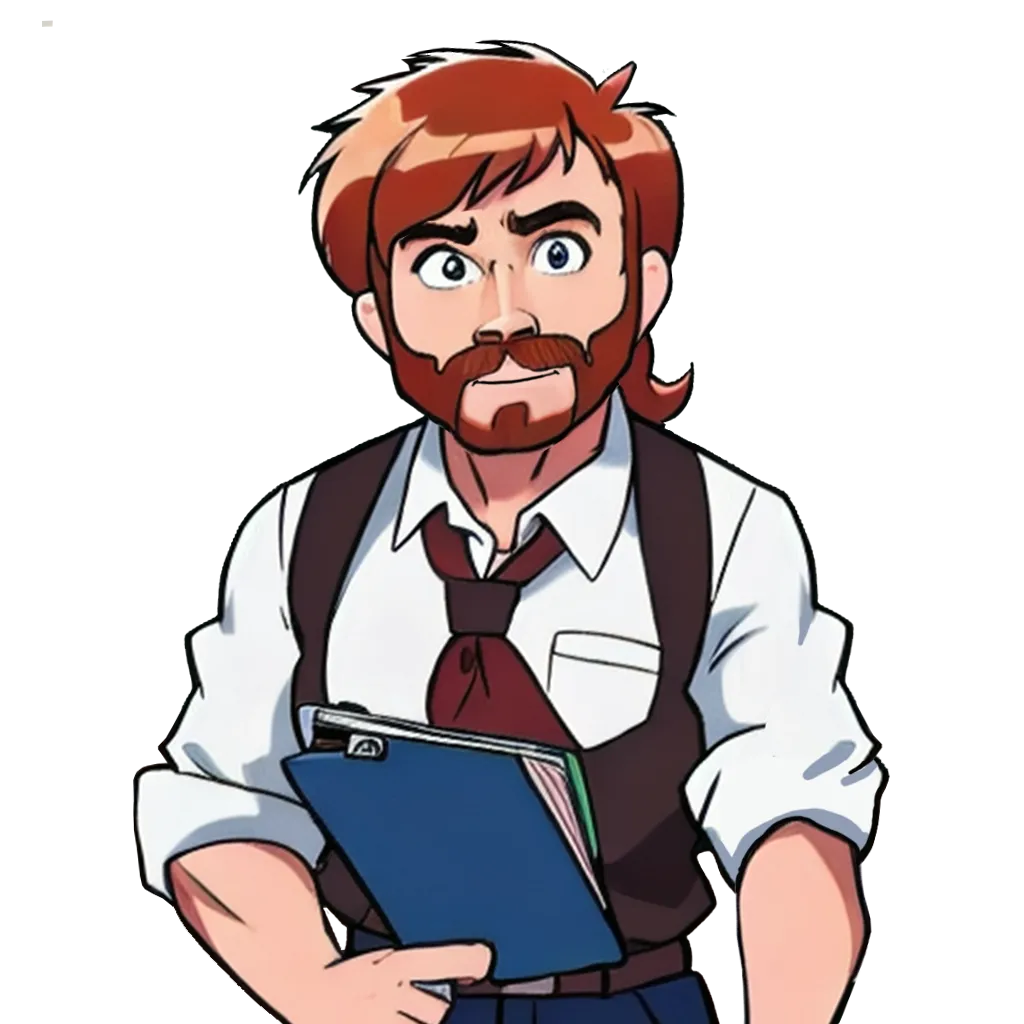
- Introduction to DOM Manipulation
- Basic Concepts of the DOM
- Selecting Elements in the DOM
- Manipulating Attributes and Properties
- Modifying Content and Structure of the DOM
- Creating and Deleting Elements
- Handling Events in the DOM
- Event Delegation
- Styles and Classes in the DOM
- Navigating the DOM
- Animations and Transitions in the DOM
- Integration of the DOM with AJAX and Fetch API
- Best Practices and Optimization for DOM Manipulation
- Tools and Libraries for DOM Manipulation
- Conclusions and Next Steps in DOM Manipulation