Testing in Node.js with Mocha and Chai
Asserts and Matchers with Chai
Chai is a powerful assertion library for JavaScript that integrates seamlessly with Mocha, providing a wide range of methods to validate the behavior of your code. Chai supports three main assertion styles: Assert, Expect, and Should. Each style has its own syntax and advantages, allowing you to choose the one that best fits your needs and preferences.
Assertion Styles in Chai
Assert
The assert
style is based on simple functions to perform comparisons. It is ideal if you prefer a more direct and explicit syntax.
Examples:
javascript
Expect
The expect
style provides a more expressive and chainable syntax, which is often considered more readable and easier to understand.
Examples:
javascript
Should
The should
style extends Object.prototype
with assertion methods, offering a similar fluency to the expect
style.
Examples:
javascript
Common Assertions
Below is a list of some of the most common assertions available in Chai, using the expect
style:
Equality and Types
javascript
Property and Length
javascript
Nulls and Undefined
javascript
Booleans
javascript
Complete Example
Below is a complete example that uses various Chai assertions to validate the behavior of a function:
javascript
Conclusion
Chai provides a rich variety of assertion methods that allow you to validate almost any aspect of your code's behavior. Whether you prefer the simplicity of the assert
style, the expressiveness of the expect
style, or the fluency of the should
style, Chai has something for you. In the next chapter, we will see how to apply Test-Driven Development (TDD) using Mocha and Chai.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
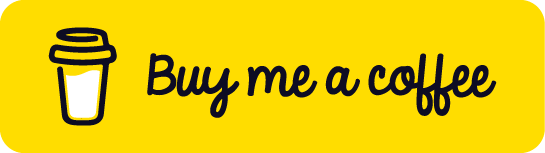
Chat with Chuck
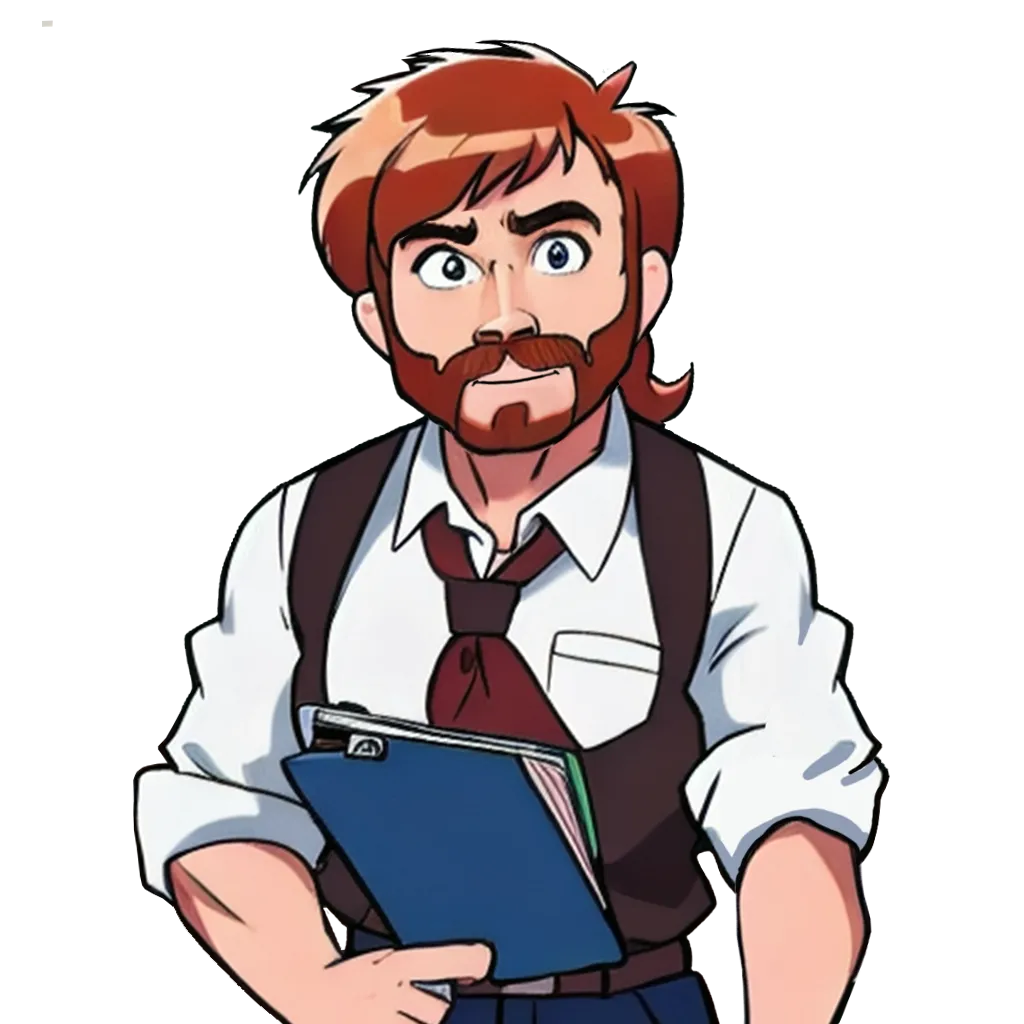
- Introduction to Testing in Node.js
- Installation and Configuration of Mocha and Chai
- Basic Testing Concepts
- Structure of a Test with Mocha
- Asserts and Matchers with Chai
- Test Driven Development (TDD) with Mocha and Chai
- Mocks and Stubs with Sinon.js
- Testing Asynchronous Functions
- Testing RESTful APIs
- Integration of Tests in the CI/CD Process
- Load and Performance Testing
- Best Practices in Testing
- Complementary Tools for Testing
- Practical Examples and Use Cases
- Conclusions and Next Steps