Testing in Node.js with Mocha and Chai
Complementary Tools for Testing
In addition to Mocha, Chai, and Sinon.js, there are several complementary tools that can enhance and simplify the testing process for your Node.js applications. These tools can help you generate test coverage reports, perform browser tests, integrate tests into your CI/CD workflows, and much more. In this chapter, we will explore some of the most useful complementary tools for testing.
Istanbul (NYC)
Istanbul is a popular tool for generating code coverage reports, and NYC is the command-line interface for Istanbul that makes it easier to use with Mocha.
Installation
shell
Basic Configuration
Add a test script using NYC in your package.json
:
json
Execution
Run your tests and generate a code coverage report:
shell
This command will generate a coverage report and place it in the coverage
folder.
Configuration Options
You can customize NYC's configuration with a .nycrc
file or by adding a nyc
section in your package.json
. Example with .nycrc
:
json
desktop-image: Placeholder for screenshot of a code coverage report generated by NYC, showing coverage graphs and metrics.
ESLint
ESLint is a static code analysis tool that identifies and fixes problems in your JavaScript/Node.js code.
Installation
shell
Configuration
Configure ESLint with the following command:
shell
Follow the instructions to create a .eslintrc.json
configuration file.
Integration with Prettier
Prettier is a tool for automatically formatting code. You can use ESLint together with Prettier to ensure your code follows a consistent style.
Installing Prettier
shell
Configuration for .eslintrc.json
json
Cypress
Cypress is a powerful tool for end-to-end testing in web applications. It provides a fast and reliable development experience for testing the UI and complete functionality.
Installation
shell
Configuration
Open Cypress:
shell
This will open Cypress's user interface where you can configure and run your tests.
Example Cypress Test
Create a test file in cypress/integration/example.spec.js
:
javascript
desktop-image: Placeholder for screenshot of Cypress UI showing a passing test and the loaded application in the browser.
Jest
Jest is an all-in-one testing framework that includes a test runner, assertions, and code coverage generation. Although not specific to Node.js, it has become popular due to its simplicity and integrated features.
Installation
shell
Basic Configuration
Update the test script in your package.json
:
json
Example Jest Test
javascript
javascript
Execution:
shell
Nock
Nock is a library for intercepting and mocking HTTP requests in Node.js, useful for tests involving external API calls.
Installation
shell
Usage Example
javascript
Conclusion
Using complementary tools for testing not only improves the coverage and quality of your tests but also makes the development and maintenance of your application easier. Tools like Istanbul for code coverage, Cypress for E2E testing, and Nock for mocking HTTP requests are just a few of the many options available to strengthen your testing strategy. In the next chapter, we will explore practical examples and use cases that illustrate how to combine these tools to achieve effective and efficient testing.
- Introduction to Testing in Node.js
- Installation and Configuration of Mocha and Chai
- Basic Testing Concepts
- Structure of a Test with Mocha
- Asserts and Matchers with Chai
- Test Driven Development (TDD) with Mocha and Chai
- Mocks and Stubs with Sinon.js
- Testing Asynchronous Functions
- Testing RESTful APIs
- Integration of Tests in the CI/CD Process
- Load and Performance Testing
- Best Practices in Testing
- Complementary Tools for Testing
- Practical Examples and Use Cases
- Conclusions and Next Steps
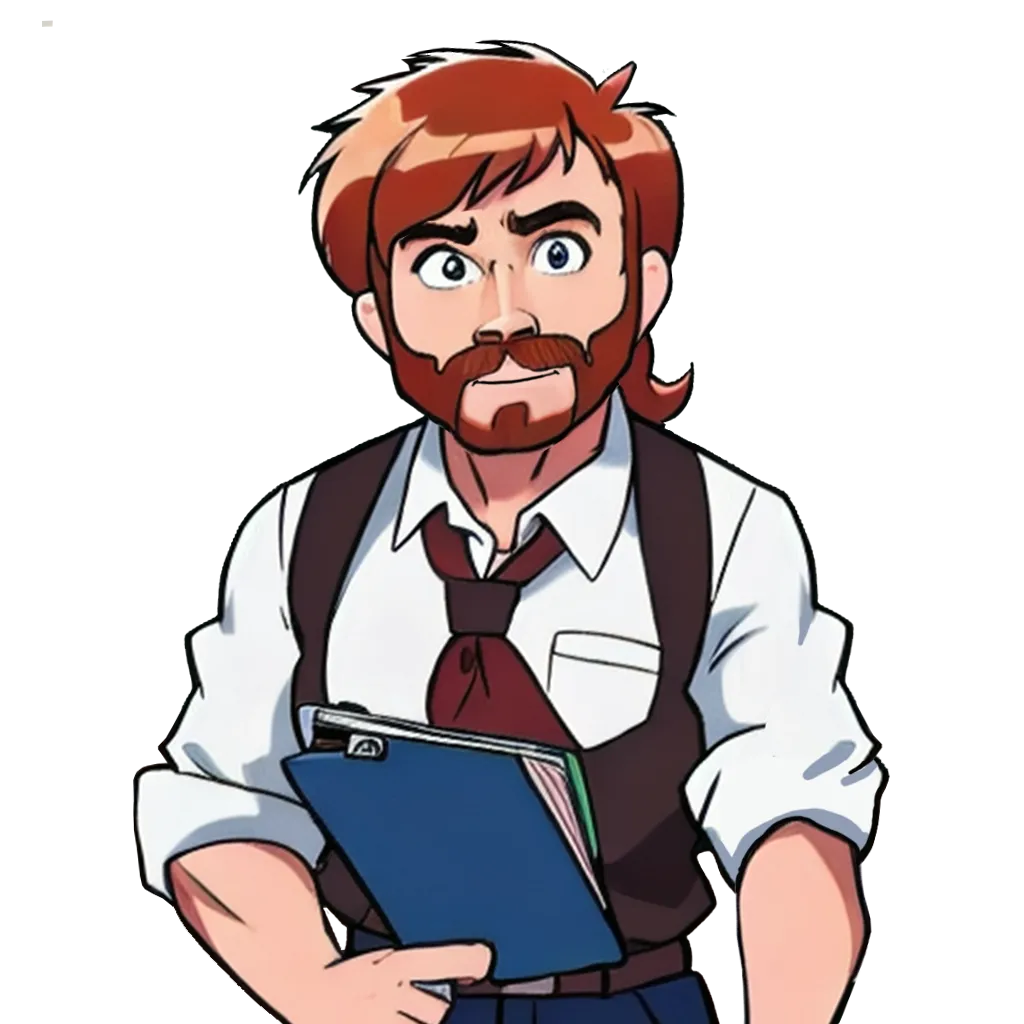