Testing in Node.js with Mocha and Chai
Testing RESTful APIs
RESTful APIs are a fundamental part of many modern applications, providing an interface to interact with services and data over HTTP. Ensuring that these APIs work correctly and handle requests and responses appropriately is crucial. In this chapter, we will explore how to test RESTful APIs using Mocha, Chai, and Supertest.
Installing Supertest
Supertest is a library that makes it easy to perform HTTP requests and verify responses in your tests. You can install Supertest along with Mocha and Chai with the following command:
shell
Server Setup
Suppose we have an Express application with an endpoint to get user information. Below is an example of how you might structure your server:
Server Implementation:
javascript
Starting the Server:
javascript
Testing Endpoints with Supertest
Now, let's write tests for the /usuario/:id
endpoint. We will create cases to verify both successful and error responses.
Test Implementation:
javascript
Advanced Considerations
Using Hooks
Use the before
and after
hooks to set up and tear down your test environment. This is useful if you need to start a server before all tests and close it after all tests.
Handling Authentication
If your API requires authentication, you can use Supertest to send tokens or credentials in the request headers.
Mocking External Responses
If your API interacts with external services, consider using tools like Sinon.js to mock these interactions and ensure your tests are deterministic.
Full Integration Tests
In addition to testing individual endpoints, consider performing integration tests that cover multiple endpoints and validate the full data flow through your API.
Complete Example with Hooks:
Below is a more advanced example that uses hooks and tests protected endpoints:
Server Implementation with Authentication:
javascript
Testing Endpoints with Authentication:
javascript
Conclusion
Testing RESTful APIs is essential to ensure that your application interacts correctly with clients and other services. By using Mocha, Chai, and Supertest, you can create robust and reliable tests that ensure the proper functioning of your endpoints. In the upcoming chapters, we will explore how to integrate these tests into CI/CD processes and how to perform load and performance testing.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
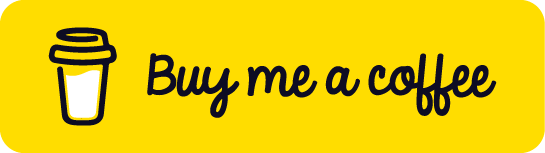
Chat with Chuck
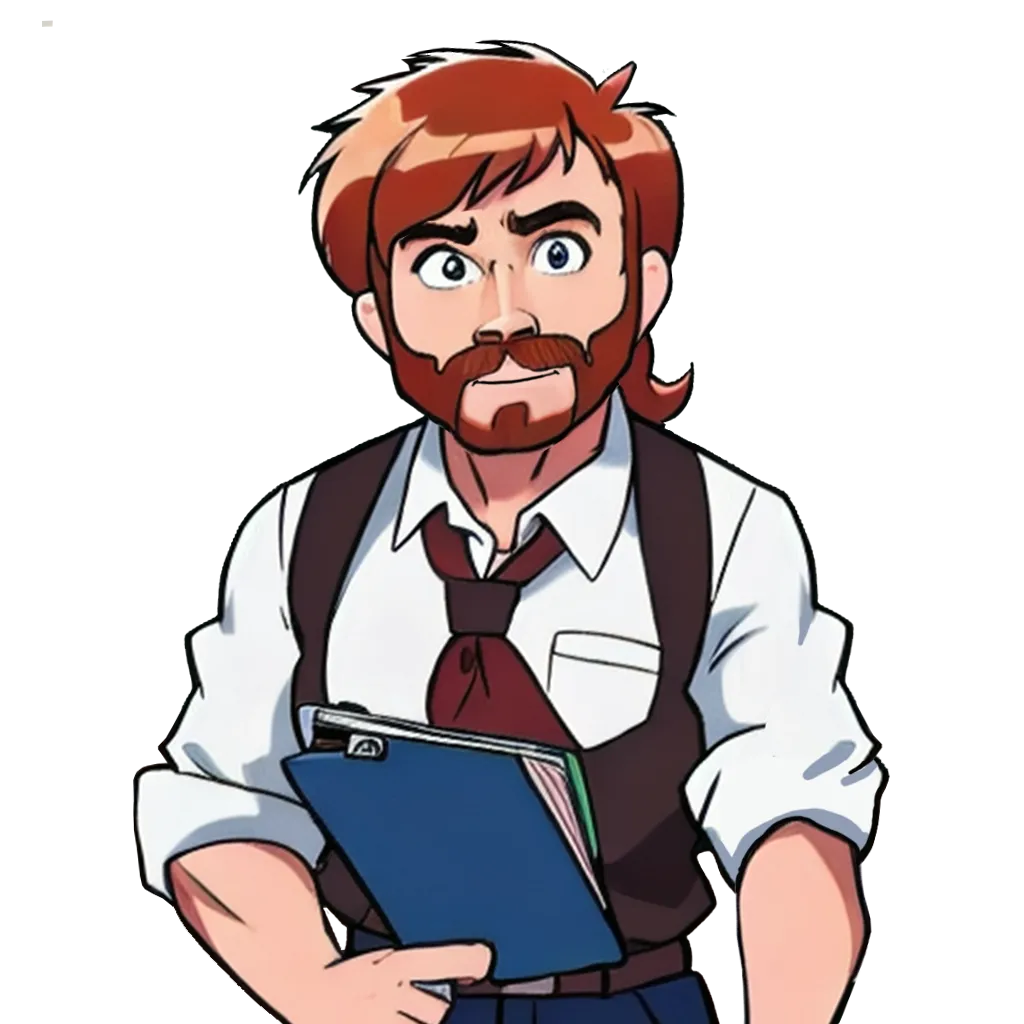
- Introduction to Testing in Node.js
- Installation and Configuration of Mocha and Chai
- Basic Testing Concepts
- Structure of a Test with Mocha
- Asserts and Matchers with Chai
- Test Driven Development (TDD) with Mocha and Chai
- Mocks and Stubs with Sinon.js
- Testing Asynchronous Functions
- Testing RESTful APIs
- Integration of Tests in the CI/CD Process
- Load and Performance Testing
- Best Practices in Testing
- Complementary Tools for Testing
- Practical Examples and Use Cases
- Conclusions and Next Steps