Testing in Node.js with Mocha and Chai
Introduction to Testing in Node.js
Modern software development is not complete without an emphasis on code quality. Software testing is crucial to ensure that our applications work as expected and to detect bugs early in the development stages. In this course, we will focus on how to perform effective testing in Node.js applications using Mocha and Chai, two libraries that make the testing process more structured and straightforward.
What is Testing?
Testing, or software testing, is the process of evaluating and verifying that a software or application does what it is supposed to do. This process may include various techniques and types of tests, such as unit tests, integration tests, functional tests, among others.
Importance of Testing
- Early Bug Detection: Tests help to identify issues in the code as early as possible, making it easier to resolve them.
- Code Confidence: Having a robust test suite allows developers to make changes to the code with confidence that they won't break existing functionalities.
- Living Documentation: Tests can act as a form of documentation on how the system should behave in different circumstances.
- Code Maintenance: Facilitates code refactoring without fear of breaking something, as tests would validate potential errors.
Why Node.js?
Node.js has gained immense popularity due to its efficiency and the ability to use JavaScript on both the client and server sides. By introducing tests in Node.js, we can leverage this highly efficient environment and ensure the quality of our applications.
Mocha and Chai
- Mocha: A JavaScript testing framework that runs on Node.js and in the browser. Mocha provides a simple and flexible environment for writing and executing tests.
- Chai: An assertion library that complements Mocha, offering a set of assertions that make it easy to validate the behavior of our code.
In the following chapters, we will explore how to install and configure these tools, the basic concepts of testing, and best practices to ensure that our tests are effective and maintain code quality.
This course will guide you through the process from initial setup to the implementation of complex tests and provide practical examples and use cases that you can apply to your projects. Let's get started!
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
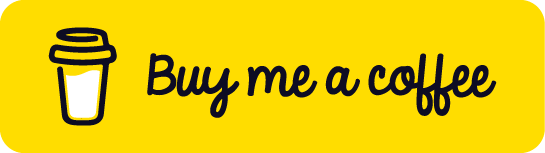
Chat with Chuck
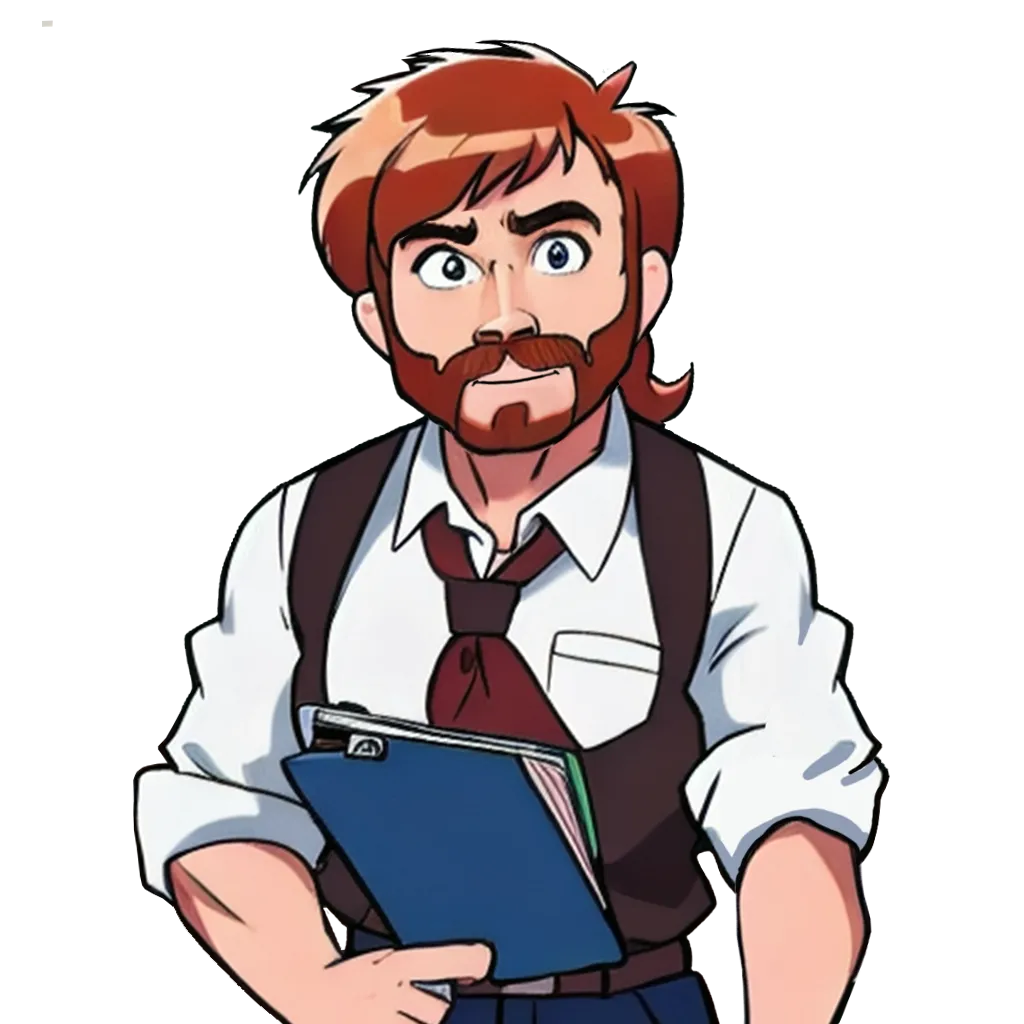
- Introduction to Testing in Node.js
- Installation and Configuration of Mocha and Chai
- Basic Testing Concepts
- Structure of a Test with Mocha
- Asserts and Matchers with Chai
- Test Driven Development (TDD) with Mocha and Chai
- Mocks and Stubs with Sinon.js
- Testing Asynchronous Functions
- Testing RESTful APIs
- Integration of Tests in the CI/CD Process
- Load and Performance Testing
- Best Practices in Testing
- Complementary Tools for Testing
- Practical Examples and Use Cases
- Conclusions and Next Steps