Testing in Node.js with Mocha and Chai
Best Practices in Testing
Software testing is a fundamental part of developing high-quality applications. Following best practices in testing not only improves the coverage and effectiveness of your tests but also facilitates code maintenance and scalability. In this chapter, we will discuss some of the best practices you can follow when writing and maintaining tests for Node.js applications using Mocha, Chai, and other testing tools.
General Principles
Automate Everything Possible
- Automation: Automate your tests as much as possible. This includes unit tests, integration tests, end-to-end (E2E) tests, and performance tests.
- CI/CD: Integrate your tests into the CI/CD pipeline to ensure that tests run on every commit or deployment.
Keep Your Tests Simple and Clear
- Readability: Write tests that are easy to understand. Use clear and meaningful descriptions for your tests and
describe
blocks. - KISS (Keep It Simple, Stupid): Avoid unnecessary complexity in your tests. Keep the focus on what you are testing.
One Test, One Behavior
- Isolation: Ensure that each test case verifies a single aspect of the system's behavior. This makes it easier to identify errors when a test fails.
- No Dependency: Tests should not depend on each other. Each test should be able to run independently.
Unit Tests
Cover Edge Cases
- Normal and Edge Cases: Ensure that you cover both normal scenarios and extreme or edge cases.
- Invalid Inputs: Test how your code behaves with invalid or unexpected inputs.
Use Mocks and Stubs Effectively
- Sinon.js: Use libraries like Sinon.js to mock and stub functions, objects, and external services.
- Isolation: Mocks and stubs allow your unit tests to focus solely on the unit of code under test, without depending on external factors.
Integration Tests
Verify Interactions
- Multiple Components: In integration tests, verify that multiple components work together as expected.
- Database and External Services: These tests may involve interactions with databases, external services, and APIs. Use realistic test environments.
Cleanup and Setup
- Hooks: Use hooks (
before
,beforeEach
,after
,afterEach
) to set up and clean up the test environment. - Test Data: Ensure you have consistent and clean test data. Reset the state before each test if necessary.
End-to-End (E2E) Tests
Simulate Real User
- Tools like Cypress: Use tools like Cypress or Selenium to simulate user interaction with your application.
- Complex Flows: Test complex flows that include multiple pages and components.
Environment Isolation
- Dedicated Environment: Run E2E tests in a dedicated environment that is as similar as possible to the production environment.
- Predictable Data: Use predictable and controlled data to avoid discrepancies in test results.
Performance Tests
Realistic Scenarios
- Realistic Load: Configure your load tests to simulate realistic traffic scenarios.
- Tools like Artillery and k6: Use these tools to measure how your application handles different levels of traffic.
Monitoring and Analysis
- Real-Time Monitoring: Implement real-time monitoring solutions to get a continuous view of your application's performance.
- Result Analysis: Analyze performance test results to identify and mitigate bottlenecks.
Test Code Management
Code Organization
- Project Structure: Organize your test code in a logical and modular way.
- Separate Folders: Place tests in separate folders according to their type (unit, integration, E2E).
Maintainability
- Refactoring: Regularly refactor your tests to keep them clean and efficient.
- Avoid Redundancies: Avoid redundancies and repetitive code by using helper functions and constants when necessary.
Example of Best Practices
Project Structure:
Example of Well-Structured Unit Test:
javascript
Conclusion
Best practices in testing ensure that your tests are effective, maintainable, and scalable. By following these principles, you can significantly improve the quality of your code and reduce the risk of errors in production. In the next chapter, we will explore complementary tools to enhance your testing strategy and maximize the efficiency of your tests.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
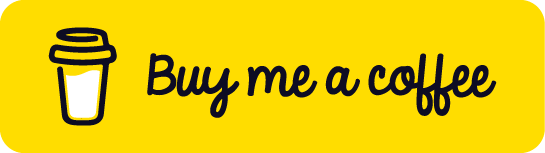
Chat with Chuck
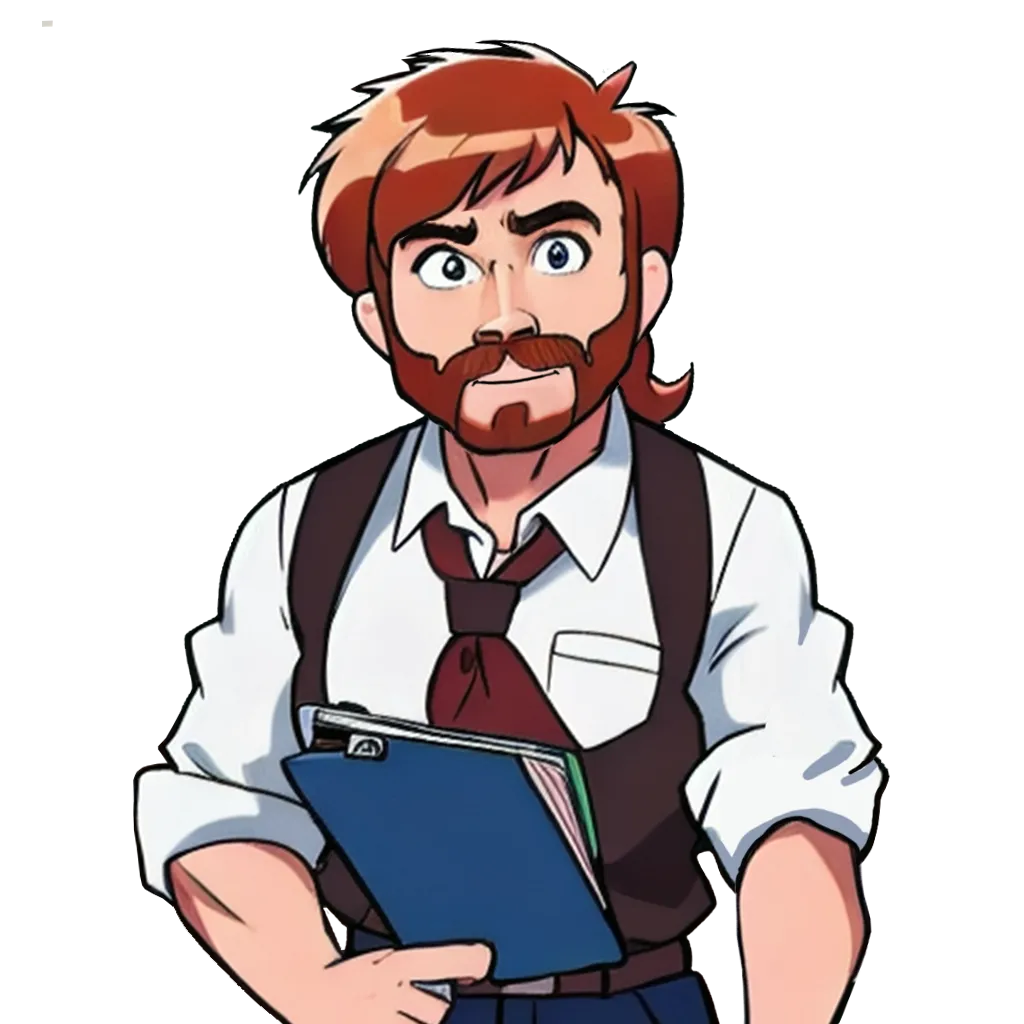
- Introduction to Testing in Node.js
- Installation and Configuration of Mocha and Chai
- Basic Testing Concepts
- Structure of a Test with Mocha
- Asserts and Matchers with Chai
- Test Driven Development (TDD) with Mocha and Chai
- Mocks and Stubs with Sinon.js
- Testing Asynchronous Functions
- Testing RESTful APIs
- Integration of Tests in the CI/CD Process
- Load and Performance Testing
- Best Practices in Testing
- Complementary Tools for Testing
- Practical Examples and Use Cases
- Conclusions and Next Steps