Testing in Node.js with Mocha and Chai
Testing Asynchronous Functions
In Node.js application development, it is very common to work with asynchronous functions that handle operations such as file reads/writes, HTTP requests, and database access. Testing these functions can be more complex than testing synchronous functions, but Mocha and Chai make this process easier with their support for asynchronous testing. In this chapter, you will learn how to write and execute tests for asynchronous functions using Mocha and Chai.
Methods for Testing Asynchronous Functions
There are several ways to test asynchronous functions in Mocha:
- Callbacks
- Promises
- async/await
Using Callbacks
Mocha automatically detects that a test function is asynchronous when it takes a done
argument. This argument is a function that must be called when the test is finished, indicating to Mocha that it can proceed to the next test.
Example with Callback:
javascript
Using Promises
Mocha also supports returning Promises from a test. If the Promise is resolved, the test passes. If the Promise is rejected, the test fails.
Example with Promises:
javascript
Using async/await
Using async
/await
is a more modern and readable way to handle asynchrony in JavaScript. By combining this with Mocha, tests become intuitive and easy to maintain.
Example with async/await:
javascript
Complete Example of Asynchronous Testing
Suppose we have a function fetchDataFromAPI
that makes an HTTP request to an external API to get data. We want to test this function without making actual requests to the API.
Implementation of fetchDataFromAPI:
javascript
Test fetchDataFromAPI with Stub:
We can use Sinon.js to create a stub for axios.get
and return fake data during the test.
javascript
Final Considerations
- Error Handling: Make sure to test not only success cases but also how your function handles errors.
- Cleanup: Use global hooks (
before
,after
) and inner hooks (beforeEach
,afterEach
) to set up and clean the test environment. - Timeouts: If your tests depend on timers or waiting time, consider using tools like
sinon
to control the timing.
Summary
Testing asynchronous functions is essential for robust and reliable Node.js applications. Whether using callbacks, Promises, or async/await, Mocha and Chai make this process simple and efficient. Combine these techniques with Sinon.js stubs and mocks for more controlled and effective tests. In the next chapter, we will explore testing RESTful APIs.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
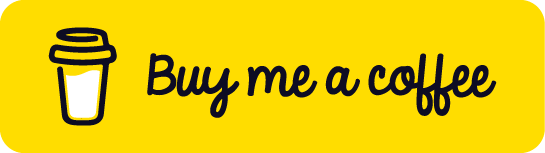
Chat with Chuck
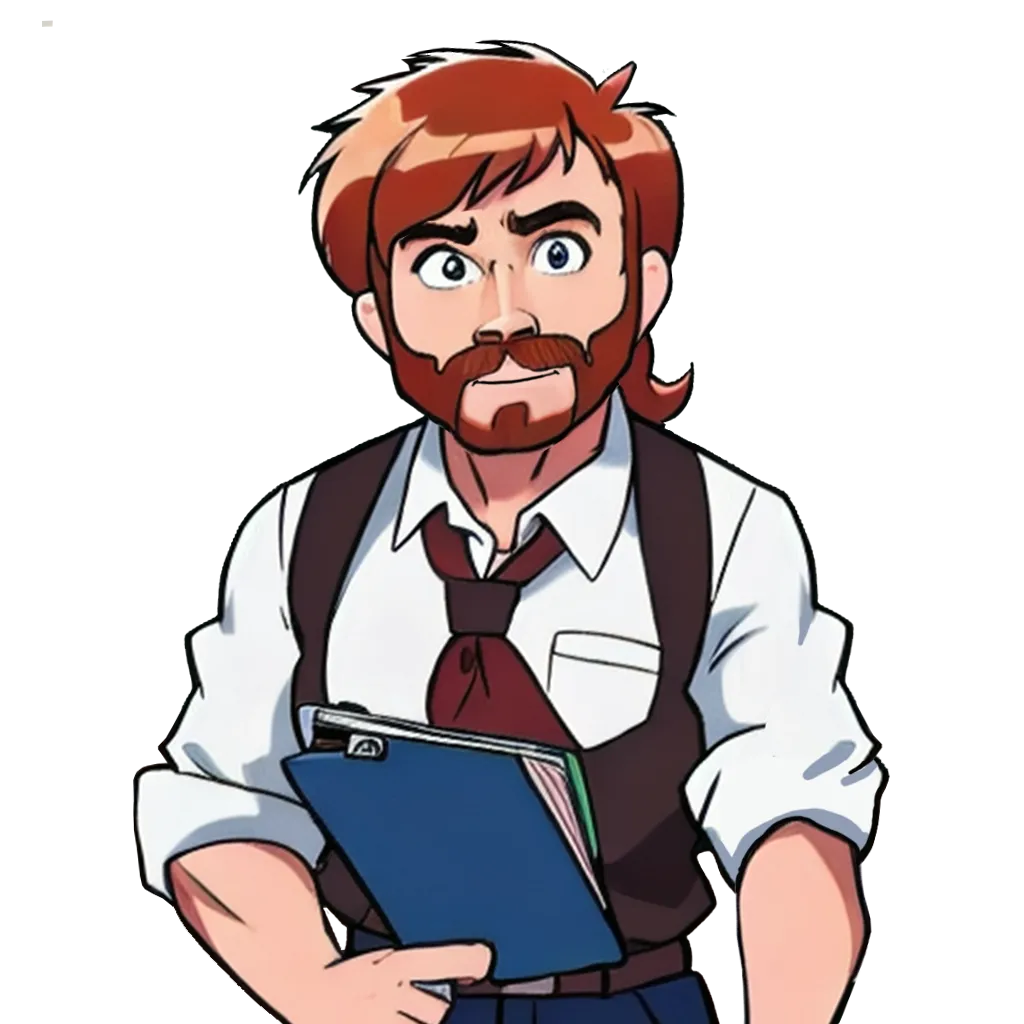
- Introduction to Testing in Node.js
- Installation and Configuration of Mocha and Chai
- Basic Testing Concepts
- Structure of a Test with Mocha
- Asserts and Matchers with Chai
- Test Driven Development (TDD) with Mocha and Chai
- Mocks and Stubs with Sinon.js
- Testing Asynchronous Functions
- Testing RESTful APIs
- Integration of Tests in the CI/CD Process
- Load and Performance Testing
- Best Practices in Testing
- Complementary Tools for Testing
- Practical Examples and Use Cases
- Conclusions and Next Steps